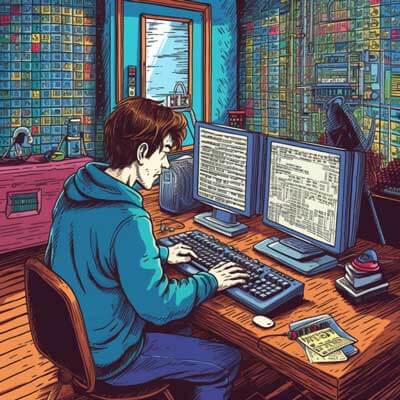
Table of Contents
In Java, splitting a string into multiple parts can be achieved using the split()
method provided by the String
class. This method allows you to split a string into an array of substrings based on a specified delimiter. This can be useful in various scenarios, such as parsing CSV files, extracting tokens from a sentence, or splitting a URL into its components.
Using the split() method
The split()
method in Java accepts a regular expression as the delimiter and returns an array of substrings. The regular expression can be a simple string or a more complex pattern. Here's the basic syntax of the split()
method:
String[] split(String regex)
Let's see some examples of how to use the split()
method to split a string in Java:
Example 1: Splitting a string using a simple delimiter
String str = "Hello,World,Java"; String[] parts = str.split(","); for (String part : parts) { System.out.println(part); }
Output:
Hello World Java
In this example, we split the string str
using a comma (,
) as the delimiter. The split()
method returns an array of substrings, which we iterate over using a for-each loop and print each substring.
Example 2: Splitting a string using a regular expression
String str = "Hello,World;Java"; String[] parts = str.split("[,;]"); for (String part : parts) { System.out.println(part); }
Output:
Hello World Java
In this example, we split the string str
using a regular expression [,;]
as the delimiter. This regular expression matches either a comma or a semicolon, allowing us to split the string on either delimiter.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Handling Special Characters
When using the split()
method, it's important to consider special characters that have a special meaning in regular expressions, such as .
(dot) and |
(pipe). If you want to split a string using these special characters as delimiters, you need to escape them using a backslash (\
).
Example: Splitting a string using a dot as the delimiter
String str = "www.example.com"; String[] parts = str.split("\\."); for (String part : parts) { System.out.println(part); }
Output:
www example com
In this example, we split the string str
using a dot (.
) as the delimiter. Since dot is a special character in regular expressions, we need to escape it using a backslash (\
). However, since backslash is also a special character in Java strings, we need to escape it again with another backslash (\\
).
Limiting the Number of Substrings
By default, the split()
method will split the entire string into as many substrings as possible. However, you can also specify a limit parameter to control the maximum number of substrings returned by the method.
Example: Splitting a string with a limit
String str = "one,two,three,four"; String[] parts = str.split(",", 2); for (String part : parts) { System.out.println(part); }
Output:
one two,three,four
In this example, we split the string str
using a comma (,
) as the delimiter and a limit of 2
. The split()
method returns an array with a maximum of 2
substrings. The first substring contains the part before the delimiter, and the second substring contains the remaining part of the string.
Why is this question asked?
The question "How to split a string in Java" is commonly asked by developers who need to manipulate and process textual data in their Java applications. Splitting a string into multiple parts is a fundamental operation when dealing with text processing tasks, such as parsing and extracting information from structured data.
Some potential reasons why this question is asked include:
1. Data parsing: Developers often need to split strings to extract specific pieces of information from structured data. For example, extracting values from CSV files, separating URL components, or parsing log files.
2. Tokenization: Splitting a string can be useful for breaking down sentences or paragraphs into individual words or tokens. This can be helpful for tasks like natural language processing, text analysis, or search indexing.
3. Data validation: Splitting a string can be used to validate and enforce specific formats or patterns. For example, checking if an input matches a certain format or extracting specific parts of an input string.
Related Article: How to Use Spring Configuration Annotation
Suggestions and Alternative Ideas
While the split()
method is a convenient way to split strings in Java, there are other techniques and libraries available that can also accomplish the same task:
1. Regular expressions: In addition to the split()
method, Java provides the Pattern
and Matcher
classes for more advanced string manipulation using regular expressions. Regular expressions offer powerful and flexible ways to split strings based on complex patterns.
2. StringTokenizer: The StringTokenizer
class is another option for splitting strings in Java. It provides methods for breaking a string into tokens based on a specified delimiter. However, note that StringTokenizer
is considered a legacy class, and the split()
method is generally preferred for modern Java applications.
3. Third-party libraries: There are also several third-party libraries available that offer additional string manipulation capabilities. For example, Apache Commons Lang provides the StringUtils
class, which includes various string manipulation methods, including string splitting.
Best Practices
When splitting strings in Java, consider the following best practices:
1. Choose the appropriate delimiter: Carefully choose the delimiter based on the structure and format of the string you want to split. Regular expressions can provide more flexibility when dealing with complex delimiters.
2. Handle special characters: Be aware of special characters that have special meanings in regular expressions and escape them properly if needed. Remember to escape backslashes in Java strings by using double backslashes.
3. Consider performance implications: Splitting strings can have performance implications, especially when dealing with large strings or performing frequent splitting operations. If performance is a concern, consider alternatives such as using StringTokenizer
or specialized libraries.
4. Validate input: Before splitting a string, validate the input to ensure it meets the expected format or pattern. This can help prevent unexpected errors or exceptions.
5. Handle edge cases: Consider how your code handles edge cases, such as empty strings, strings with leading or trailing delimiters, or strings with consecutive delimiters. Test your code with various inputs to ensure it behaves as expected in all scenarios.