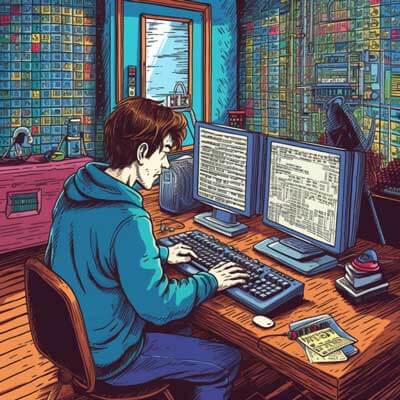
Table of Contents
To uninstall all pip packages in Python, you can follow the steps below:
Step 1: Checking installed packages
Before uninstalling all pip packages, it's a good practice to check the list of installed packages first. You can use the following command in the terminal or command prompt:
pip list
This will display a list of installed packages along with their versions.
Related Article: PHP vs Python: How to Choose the Right Language
Step 2: Uninstalling individual packages
To uninstall individual packages, you can use the pip uninstall
command followed by the package name. For example, to uninstall a package called requests
, you can use the following command:
pip uninstall requests
Repeat this command for each package you want to uninstall.
Step 3: Removing all packages
To remove all installed packages, you can use the pip freeze
command along with the xargs
command in Linux-based systems. Here's an example:
pip freeze | xargs pip uninstall -y
In this command, pip freeze
lists all installed packages and their versions. The xargs
command passes each package to the pip uninstall
command with the -y
flag, which automatically confirms the uninstallation.
Step 4: Alternative approach using requirements.txt file
Another approach to uninstall all packages is to use a requirements.txt
file that lists all installed packages. You can generate this file using the pip freeze
command:
pip freeze > requirements.txt
Once you have the requirements.txt
file, you can uninstall all packages by running the following command:
pip uninstall -r requirements.txt -y
This command uninstalls all packages listed in the requirements.txt
file.
Related Article: How to Structure Unstructured Data with Python
Step 5: Best practices
When uninstalling all pip packages, it's important to consider the following best practices:
- Make sure you are in the correct virtual environment if you are using one. Uninstalling packages outside the intended environment can cause issues.
- Double-check the list of packages before confirming the uninstallation. Removing packages without proper consideration can lead to unexpected behavior in your projects.
- If you are planning to reinstall packages after uninstalling, it's a good practice to create a virtual environment and install the necessary packages using a requirements.txt
file or manually.