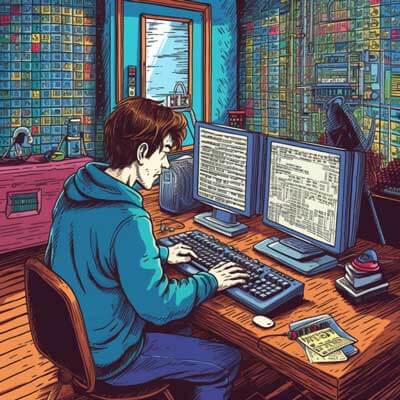
Table of Contents
To unzip files in Python, you can use the built-in zipfile
module. This module provides functionality to manipulate ZIP archives. Here are two possible approaches:
Approach 1: Using the zipfile
module
1. Import the zipfile
module:
import zipfile
2. Open the ZIP file using the ZipFile
class:
with zipfile.ZipFile('example.zip', 'r') as zip_ref: # Perform operations on the ZIP file
3. Extract all files from the ZIP archive:
zip_ref.extractall('destination_folder')
4. Extract a specific file from the ZIP archive:
zip_ref.extract('file.txt', 'destination_folder')
5. Close the ZIP file:
zip_ref.close()
Related Article: How to use the Python Random Module: Use Cases and Advanced Techniques
Approach 2: Using the shutil
module
1. Import the shutil
module:
import shutil
2. Extract all files from the ZIP archive:
shutil.unpack_archive('example.zip', 'destination_folder', 'zip')
3. Extract a specific file from the ZIP archive:
shutil.unpack_archive('example.zip', 'destination_folder', 'zip', 'file.txt')
These approaches provide a straightforward way to unzip files in Python using the zipfile
and shutil
modules. Remember to replace 'example.zip'
with the path to your ZIP file and 'destination_folder'
with the desired destination folder.
Best Practices
Related Article: How to Add New Keys to a Python Dictionary
Here are some best practices to consider when working with ZIP files in Python:
- Always use the with
statement when working with ZipFile
objects. This ensures that the file is properly closed after use, even if an exception occurs.
- Check if a file exists before extracting it from the ZIP archive to avoid overwriting existing files.
- Consider using the pathlib
module to handle file paths in a more platform-independent manner.
- Use exception handling to handle errors when working with ZIP files, such as handling cases where the file is not found or the extraction fails.