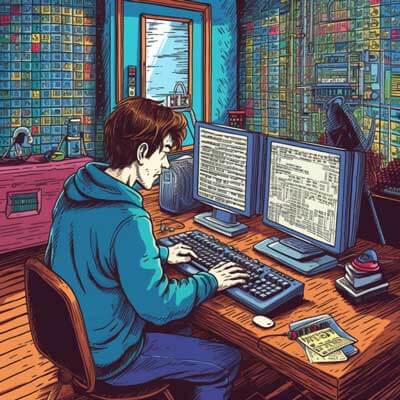
Table of Contents
To update a MySQL query based on a select query, you can use the UPDATE statement with a subquery. This allows you to update rows in one table based on the values returned from a select query on another table. Here are two possible approaches:
Approach 1: Using a Subquery
1. Start by writing a select query that retrieves the desired rows from the source table. For example, if you want to update the "price" column in the "products" table based on the average price of products in the "sales" table, you can use the following select query:
SELECT AVG(price) FROM sales;
2. Use this select query as a subquery in the UPDATE statement to update the desired rows in the target table. In this example, the UPDATE statement would look like this:
UPDATE products SET price = (SELECT AVG(price) FROM sales);
This will update the "price" column in the "products" table with the average price from the "sales" table.
Related Article: Integrating Fluent Bit with PostgreSQL Databases
Approach 2: Using JOIN
1. Start by writing a select query that retrieves the desired rows from both the source and target tables. Use a JOIN to combine the tables based on a common column. For example, if you want to update the "quantity" column in the "orders" table based on the "quantity" column in the "order_items" table, you can use the following select query:
SELECT o.order_id, oi.quantity FROM orders o JOIN order_items oi ON o.order_id = oi.order_id;
2. Use this select query as a subquery in the UPDATE statement to update the desired rows in the target table. In this example, the UPDATE statement would look like this:
UPDATE orders o JOIN order_items oi ON o.order_id = oi.order_id SET o.quantity = oi.quantity;
This will update the "quantity" column in the "orders" table with the corresponding values from the "order_items" table.
Best Practices
Related Article: How to Select Specific Columns in SQL Join Operations
- Before updating a table based on a select query, make sure to thoroughly test your select query to ensure it returns the correct results. This will help prevent unintended updates to your data.
- Use proper indexing on the columns used in the join condition to improve the performance of the update query.
- Always backup your database before performing any updates to avoid data loss in case of mistakes.
These are two possible approaches for updating a MySQL query based on a select query. Choose the one that best fits your specific requirements and follow the best practices to ensure the accuracy and efficiency of your updates.