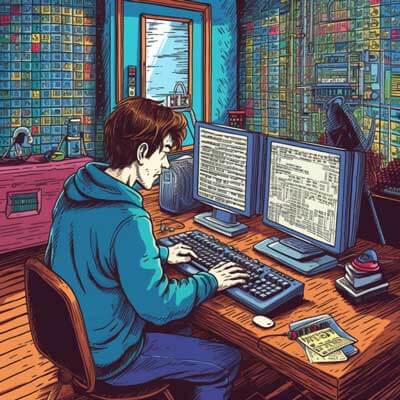
Table of Contents
The "foreach" function, also known as a "for-each" loop, is a useful construct in Python 3 for iterating over elements in a collection. It allows you to perform a specific operation on each item in the collection without the need to manually manage the index or length of the collection. In Python, the "foreach" function is implemented using the "for" loop.
Syntax
The syntax for using the "foreach" function in Python 3 is as follows:
for item in collection: # code to be executed for each item
In this syntax, "item" is a variable that represents each element in the collection, and "collection" is the iterable object you want to loop through. The code inside the loop will be executed once for each item in the collection.
Related Article: How to Measure Elapsed Time in Python
Example 1: Iterating over a List
Let's start with a simple example of using the "foreach" function to iterate over a list in Python:
fruits = ['apple', 'banana', 'orange'] for fruit in fruits: print(fruit)
Output:
apple banana orange
In this example, the "fruits" list contains three elements: 'apple', 'banana', and 'orange'. The "foreach" loop iterates over each item in the list and prints it to the console.
Example 2: Iterating over a Dictionary
You can also use the "foreach" function to iterate over a dictionary in Python. When iterating over a dictionary, the "item" variable represents the keys of the dictionary. To access the corresponding values, you can use the "item" variable as the key:
student_scores = {'John': 90, 'Jane': 85, 'Alex': 95} for student in student_scores: print(f"{student}: {student_scores[student]}")
Output:
John: 90 Jane: 85 Alex: 95
In this example, the "student_scores" dictionary contains the names of students as keys and their corresponding scores as values. The "foreach" loop iterates over each key in the dictionary and prints both the key (student name) and value (score) to the console.
Best Practices
When using the "foreach" function in Python, consider the following best practices:
1. Use descriptive variable names: Choose meaningful names for the "item" variable and the collection variable to improve the readability of your code. For example, if you are iterating over a list of books, you could use "book" as the variable name instead of "item".
2. Avoid modifying the collection: It is generally recommended not to modify the collection while iterating over it using a "foreach" loop. Modifying the collection can lead to unexpected behavior and errors. If you need to modify the collection, consider creating a copy of it before iterating.
3. Use list comprehension for transformations: If you need to transform the elements in the collection, consider using list comprehension instead of modifying the original collection within the "foreach" loop. List comprehension provides a concise and efficient way to create a new list based on an existing one.
4. Use the "enumerate" function for accessing both index and value: If you need to access both the index and value of each item in the collection, you can use the "enumerate" function in combination with the "foreach" loop. The "enumerate" function returns both the index and value of each item in the collection.
fruits = ['apple', 'banana', 'orange'] for index, fruit in enumerate(fruits): print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple Index: 1, Fruit: banana Index: 2, Fruit: orange
In this example, the "enumerate" function is used to get both the index and value of each fruit in the "fruits" list. The "foreach" loop then prints the index and fruit to the console.