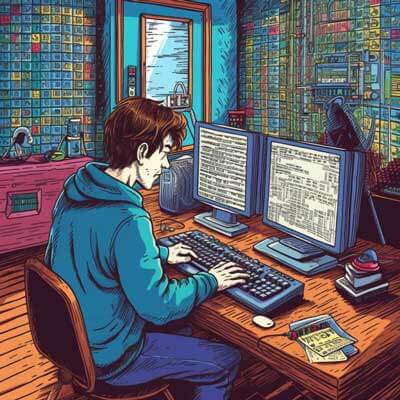
Regular expressions are a useful tool in JavaScript for pattern matching and manipulating strings. In the context of matching URLs, regular expressions can be used to validate and extract specific components from a URL string.
Matching a Basic URL
To match a basic URL in JavaScript using a regular expression, you can use the following pattern:
const urlPattern = /^(http|https):\/\/([\w-]+(\.[\w-]+)+)(\/[\w-./?%&=]*)?$/;
Let’s break down the components of this regular expression:
– ^
asserts the start of the string.
– (http|https)
matches either “http” or “https”.
– :\/\/
matches the characters “://”.
– ([\w-]+(\.[\w-]+)+)
matches the domain name, allowing alphanumeric characters, hyphens, and dots. It ensures that there is at least one dot and one alphanumeric character in the domain name.
– (\/[\w-./?%&=]*)?
matches the optional path, allowing alphanumeric characters, hyphens, dots, slashes, question marks, percentage signs, and ampersands. The path starts with a slash (“/”).
– $
asserts the end of the string.
Here’s an example of how you can use this regular expression to match a URL in JavaScript:
const url = "https://www.example.com/path/?param=value"; const isUrlValid = urlPattern.test(url); console.log(isUrlValid); // Output: true
This regular expression can be used to validate URLs that start with either “http://” or “https://”, followed by a valid domain name and an optional path.
Related Article: How to Compare Arrays in Javascript
Extracting Components from a URL
In addition to matching a URL, you may also want to extract specific components from the URL string. Regular expressions can be used to achieve this as well.
For example, to extract the domain name from a URL, you can use the following regular expression:
const url = "https://www.example.com/path/?param=value"; const domainName = url.match(/^(?:https?:\/\/)?([\w-]+(\.[\w-]+)+)/)[1]; console.log(domainName); // Output: www.example.com
In this example, the regular expression captures the domain name portion of the URL string and extracts it using the match()
method. The domain name is captured by the capturing group ([\w-]+(\.[\w-]+)+)
, and match()[1]
retrieves the captured value.
Similarly, you can extract other components such as the protocol, path, query parameters, and more using regular expressions and the appropriate capturing groups.
Best Practices
When using regular expressions to match URLs in JavaScript, consider the following best practices:
1. Use specific regular expressions: Depending on your use case, you may need to adapt the regular expression to match specific URL formats. Avoid using overly generic regular expressions that may match invalid URLs.
2. Handle edge cases: URLs can have various formats and can include special characters. Ensure that your regular expression accounts for edge cases such as internationalized domain names, special characters in the path, and query parameters.
3. Test your regular expressions: Regular expressions can be complex, and it’s important to test them thoroughly. Use test cases that cover different URL formats and edge cases to ensure that your regular expression behaves as expected.
4. Consider using a URL parsing library: While regular expressions can handle basic URL matching and extraction, for more complex scenarios, consider using a dedicated URL parsing library like the URL
object in JavaScript or third-party libraries like url-parse
or url-regex
.
Alternative Approach
Another approach to matching URLs in JavaScript is to use the URL
object, which provides a built-in way to parse and manipulate URLs. This approach is often more straightforward and less error-prone than using regular expressions.
Here’s an example of how you can use the URL
object to match a URL in JavaScript:
const url = "https://www.example.com/path/?param=value"; let parsedUrl; try { parsedUrl = new URL(url); console.log(parsedUrl.href); // Output: https://www.example.com/path/?param=value } catch (error) { console.log("Invalid URL"); }
In this example, the URL
object is used to parse the URL string. If the URL is valid, the URL
object will be created, and you can access its properties such as href
, protocol
, host
, pathname
, search
, and more.
Using the URL
object provides a more robust and standardized approach to working with URLs in JavaScript. It automatically handles URL encoding, validation, and extraction of various components.
However, note that the URL
object is not available in older versions of JavaScript or in some environments, so make sure to check the compatibility before relying on it.
Related Article: How to Create a Countdown Timer with Javascript