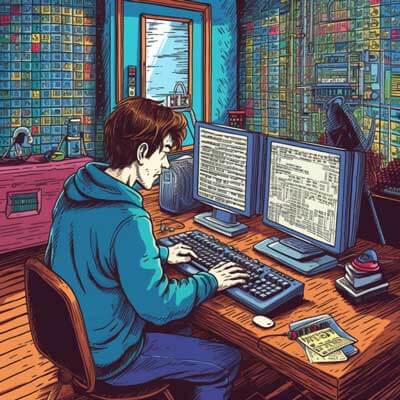
Table of Contents
A .sh file, also known as a shell script, is a text file containing a series of commands that are executed in a sequence. These scripts are commonly used in Linux environments to automate tasks or execute a set of commands. In this guide, we will walk you through the steps of using a .sh file in Linux.
1. Create a .sh File
To use a .sh file, you first need to create one. Open a text editor of your choice and create a new file. Give it a descriptive name and use the .sh extension at the end. For example, you can name it "myscript.sh".
Related Article: How to Pass Parameters to Scripts in Bash
2. Add Commands to the .sh File
Once you have created the .sh file, you can start adding commands to it. Each command should be written on a new line. You can include any valid Linux command or a series of commands in your script.
For example, let's say you want to create a script that displays the current date and time, as well as the list of files in a specific directory. You can add the following commands to your script:
#!/bin/bash echo "Current date and time:" date echo "Files in the directory:" ls /path/to/directory
In the above example, the first line #!/bin/bash
is called the shebang. It specifies the interpreter to use (in this case, bash) to run the script.
3. Make the .sh File Executable
Before you can execute a .sh file, you need to make it executable. To do this, you can use the chmod
command. Open a terminal and navigate to the directory where your .sh file is located. Then, run the following command:
chmod +x myscript.sh
This command grants execute permissions to the file, allowing you to run it as a script.
4. Execute the .sh File
Once you have made the .sh file executable, you can run it using the terminal. Navigate to the directory where the .sh file is located and run the following command:
./myscript.sh
The ./
before the file name tells the terminal to execute the script in the current directory.
Related Article: How to Run Bash Scripts in the Zsh Shell
5. Understanding Exit Codes
When a .sh file is executed, it can return an exit code indicating the success or failure of the script. An exit code of 0 typically indicates success, while a non-zero exit code indicates an error or failure.
You can access the exit code of the last executed command or script by using the special variable $?
. For example, you can modify your script to display the exit code as follows:
#!/bin/bash echo "Current date and time:" date echo "Files in the directory:" ls /path/to/directory echo "Exit code: $?"
6. Passing Arguments to the .sh File
You can also pass arguments to a .sh file when executing it. These arguments can be accessed within the script using special variables such as $1
, $2
, and so on.
For example, let's modify our script to accept a directory path as an argument and display the files in that directory:
#!/bin/bash echo "Current date and time:" date echo "Files in the directory:" ls "$1" echo "Exit code: $?"
In the above script, the directory path is passed as the first argument ($1
). You can execute the script and pass the directory path as follows:
./myscript.sh /path/to/directory
7. Best Practices
When working with .sh files, it is important to follow best practices to ensure readability and maintainability. Here are some recommendations:
- Use meaningful names for your .sh files to describe their purpose.
- Include comments in your script to provide explanations for complex commands or to document the script's functionality.
- Use indentation and proper spacing to improve readability.
- Test your script thoroughly before deploying it to ensure it functions as expected.
- Regularly backup your .sh files to avoid losing important scripts.