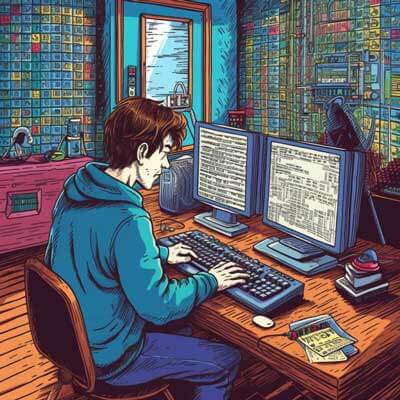
Introduction
The assert
statement in Python is a useful tool for debugging and testing code. It allows you to check if a given condition is true and raises an exception if it is not. This can be particularly helpful in identifying and fixing issues in your code. In this guide, we will explore how to use the assert
statement effectively in Python.
Related Article: How to Use Infinity in Python Math
Syntax
The syntax of the assert
statement is as follows:
assert condition, message
The condition
is the expression to be evaluated. If the condition evaluates to False
, the assert
statement raises an AssertionError
exception. The optional message
parameter can be used to provide additional information about the assertion failure.
Using Assert in Python
To use assert
in Python, follow these steps:
1. Identify the condition that you want to check. This can be any expression that evaluates to either True
or False
.
2. Write an assert
statement that includes the condition and an optional message.
Here’s an example that demonstrates the usage of assert
:
def divide(a, b): assert b != 0, "Cannot divide by zero!" return a / b result = divide(10, 2) print(result) # Output: 5.0 result = divide(10, 0) print(result) # Raises an AssertionError with the message "Cannot divide by zero!"
In this example, the assert
statement checks if the divisor b
is not equal to zero before performing the division operation. If the condition is False
, an AssertionError
is raised with the specified message.
Best Practices
To make the most out of the assert
statement, consider the following best practices:
1. Use assert
for debugging and testing purposes. It is not intended to handle runtime errors or exceptions that are expected to occur during normal program execution.
2. Keep the condition simple and concise. Complex conditions can make the code harder to read and understand.
3. Include a meaningful message when using assert
to provide clear information about the assertion failure.
4. Use assert
sparingly and only when necessary. Overusing assert
can clutter your code and make it harder to maintain.
Related Article: How to Execute a Program or System Command in Python
Alternatives to Assert
While the assert
statement can be useful in certain situations, there are alternative approaches that you can consider:
1. Exception Handling: Instead of using assert
to check conditions, you can use traditional exception handling techniques. This gives you more control over how errors are handled and allows you to provide custom error messages.
2. Unit Testing: For more comprehensive testing, consider using a unit testing framework such as unittest
or pytest
. These frameworks provide a structured way to write tests and assert conditions.
Here’s an example that demonstrates exception handling as an alternative to using assert
:
def divide(a, b): if b == 0: raise ZeroDivisionError("Cannot divide by zero!") return a / b try: result = divide(10, 0) print(result) except ZeroDivisionError as e: print(e) # Output: Cannot divide by zero!
In this example, a ZeroDivisionError
exception is raised when the divisor b
is equal to zero. The exception is then caught using a try-except
block, allowing you to handle the error gracefully.