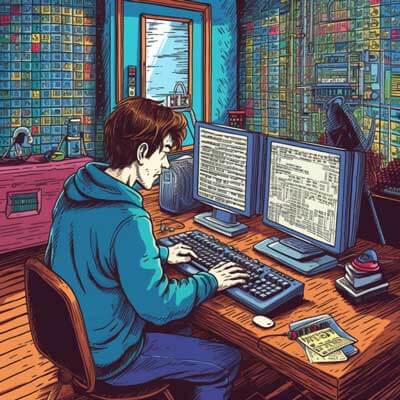
Table of Contents
Overview
Clean Webpack Plugin is a useful tool for managing the output directory in a Webpack project. It ensures that old files are removed before each build, which prevents stale assets from persisting in your build directory. This feature is particularly beneficial in projects with frequent updates, as it keeps the output clean and organized. The plugin interacts with the file system to delete unnecessary files, making it easier to maintain the integrity of your assets.
Related Article: How to Set LibraryTarget in the Webpack Configuration
Installation Steps
To install Clean Webpack Plugin, you will need to have Node.js and npm (Node Package Manager) installed. The installation process involves a few simple steps:
1. Open your terminal.
2. Navigate to your project directory.
3. Run the following command to install the plugin:
npm install clean-webpack-plugin --save-dev
This command installs the Clean Webpack Plugin as a development dependency in your project.
Configuration Options
Configuring the Clean Webpack Plugin involves adding it to your Webpack configuration file. The plugin accepts various options that allow customization of its behavior. Below is an example of how to set it up in a Webpack configuration file:
// webpack.config.js const { CleanWebpackPlugin } = require('clean-webpack-plugin'); module.exports = { // other configuration options... plugins: [ new CleanWebpackPlugin({ cleanOnceBeforeBuildPatterns: ['**/*', '!static-files*'], verbose: true, dry: false }), ], };
In this example:
- cleanOnceBeforeBuildPatterns
specifies which files and directories to clean. The pattern ['**/*', '!static-files*']
means to clean everything except for files in the static-files
directory.
- verbose
enables logging, providing information on which files are being removed.
- dry
when set to true, simulates the cleaning process without actually deleting any files.
Using Clean Webpack Plugin in Production Builds
When preparing for production builds, Clean Webpack Plugin plays a crucial role in ensuring that only the necessary files are delivered. By integrating the plugin into your production Webpack configuration, you can prevent old assets from being served. This is vital for maintaining performance and reliability. To use it in production, ensure that it’s included in the plugins array of your Webpack configuration:
// webpack.prod.js const { CleanWebpackPlugin } = require('clean-webpack-plugin'); module.exports = { mode: 'production', plugins: [ new CleanWebpackPlugin(), // other production plugins... ], // other configuration options... };
This setup ensures that every time you run a production build, the output directory is cleaned.
Related Article: How to Use Webpack Manifest Plugin
Managing Multiple Output Directories
In projects where multiple output directories are involved, Clean Webpack Plugin can be configured to handle them effectively. By specifying multiple paths in the configuration, you can ensure that each directory is cleaned as needed. Here’s how you can achieve this:
// webpack.config.js const { CleanWebpackPlugin } = require('clean-webpack-plugin'); module.exports = { // other configuration options... plugins: [ new CleanWebpackPlugin({ cleanOnceBeforeBuildPatterns: ['dist/<strong>', 'build/</strong>'], }), ], };
In this example, both dist
and build
directories will be cleaned before a new build is generated, preventing any old files from remaining.
File System Manipulation
The Clean Webpack Plugin interacts directly with the file system to remove files. It uses Node.js’ fs module under the hood to perform deletions. Understanding how this manipulation works can help in customizing the plugin further. For instance, you can create custom scripts that execute file operations before Webpack runs. Here’s a simple example of how to perform an operation before Webpack builds:
const fs = require('fs'); fs.unlinkSync('./dist/some-old-file.js'); // Deletes specific file // Then run Webpack build...
This manipulation allows for more granular control over what gets deleted, complementing the functionality of Clean Webpack Plugin.
Impact of Clean Webpack Plugin on Build Times
Incorporating Clean Webpack Plugin can have an impact on build times, particularly during large builds with many assets. The plugin adds an initial overhead as it scans the output directory and deletes unnecessary files. However, this time can be offset by the improved performance during subsequent builds, as only relevant files will be processed. To measure the impact on build times, consider running builds with and without the plugin and comparing the results.
Caching Strategies
Caching is essential for optimizing build performance. Clean Webpack Plugin can influence caching strategies by ensuring that only the latest files are cached. When old files are removed, the cache can be more effectively utilized, leading to faster rebuilds. To implement caching effectively, configure your Webpack output settings alongside the Clean Webpack Plugin:
output: { filename: '[name].[contenthash].js', path: path.resolve(__dirname, 'dist'), clean: true, // Automatically remove old files },
This configuration ensures that output files are hashed based on their content, enhancing caching mechanisms.
Related Article: How to Choose Between Gulp and Webpack
Performance Enhancement through Clean Webpack Plugin
Performance enhancement is a significant benefit of using Clean Webpack Plugin. By keeping the output directory clean, the plugin reduces the chances of serving outdated or unnecessary files, which can slow down loading times in production. Additionally, when combined with other Webpack optimizations, such as code splitting and asset compression, Clean Webpack Plugin can help achieve a more responsive application.
Asset Management
Managing assets in a Webpack project is crucial for maintaining a smooth workflow. Clean Webpack Plugin contributes to asset management by ensuring that only the latest assets are present after a build. This reduces the risk of serving outdated files. To effectively manage assets, pair Clean Webpack Plugin with asset-related plugins, such as MiniCssExtractPlugin for CSS files:
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [ new CleanWebpackPlugin(), new MiniCssExtractPlugin({ filename: '[name].[contenthash].css', }), ], };
This combination helps keep both JavaScript and CSS files organized.
Source Maps and Clean Webpack Plugin Integration
Source maps are essential for debugging, allowing developers to trace errors back to the original source code. Clean Webpack Plugin works seamlessly with source maps. When cleaning the output directory, it does not interfere with the generation of source maps. To enable source maps in your Webpack configuration, you can set:
module.exports = { devtool: 'source-map', // Generates source maps plugins: [ new CleanWebpackPlugin(), ], };
This setup ensures that your output remains clean while still providing the necessary source maps for debugging.
Hot Module Replacement
Hot Module Replacement (HMR) allows developers to update modules in a running application without a full reload. Clean Webpack Plugin does not directly impact HMR, but it is still important to ensure that old modules do not linger around during development. You can configure HMR alongside Clean Webpack Plugin for smoother development experiences:
const webpack = require('webpack'); module.exports = { devServer: { hot: true, }, plugins: [ new CleanWebpackPlugin(), new webpack.HotModuleReplacementPlugin(), ], };
This configuration keeps your development environment clean and responsive.
Related Article: How to Configure SVGR with Webpack
Compatibility of Clean Webpack Plugin with Webpack 5
Clean Webpack Plugin is fully compatible with Webpack 5. It has been updated to leverage the features of the latest Webpack version, such as improved caching and performance optimizations. When working with Webpack 5, ensure that you are using the latest version of Clean Webpack Plugin to take advantage of these enhancements.
npm install clean-webpack-plugin@latest --save-dev
This command installs the latest version, ensuring compatibility.
Combining Clean Webpack Plugin with Other Plugins
Combining Clean Webpack Plugin with other Webpack plugins can create a robust build process. For instance, integrating it with TerserWebpackPlugin for minification can streamline your builds:
const TerserWebpackPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserWebpackPlugin()], }, plugins: [ new CleanWebpackPlugin(), ], };
This combination cleans the output directory and minimizes your code in one go.
Best Practices
To ensure optimal use of Clean Webpack Plugin, follow these best practices:
1. Always specify patterns in cleanOnceBeforeBuildPatterns
to avoid accidental deletions.
2. Use verbose
logging during development to track what files are being removed.
3. Test the plugin in a staging environment to confirm its behavior before deploying to production.
4. Combine it with other plugins that enhance your build process, such as asset management and minification plugins.
5. Regularly update the Clean Webpack Plugin to benefit from the latest features and fixes.
Additional Resources
- Clean Webpack Plugin Documentation