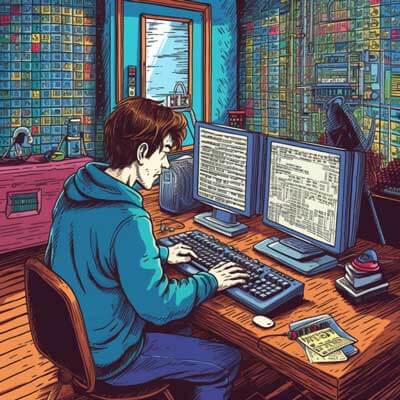
Table of Contents
Overview of Compression Plugins in Build Tools
Compression plugins play a vital role in the modern web development process. They are designed to reduce the size of files that are served to users, leading to faster load times and improved performance. In the context of build tools, these plugins automate the process of compressing assets during the build stage, making it easier for developers to optimize their applications without manual intervention.
Webpack, one of the most popular build tools, offers various compression plugins that help in minimizing file sizes. These plugins work by taking your JavaScript, CSS, and HTML files and compressing them to save bandwidth and improve the speed of serving these files to users.
Related Article: How to Bypass a Router with Webpack Proxy
What is Gzip Compression and Its Benefits
Gzip is a file format and software application used for file compression and decompression. It reduces the size of files by using a combination of Lempel-Ziv coding and Huffman coding. When a web server serves files compressed with Gzip, it can significantly reduce the amount of data transferred over the network.
Benefits of Gzip compression include:
1. Reduced File Size: Gzip can reduce file sizes by up to 70% in some cases, making downloads faster.
2. Improved Load Times: Smaller files mean quicker load times for users, which enhances user experience.
3. Lower Bandwidth Costs: With reduced file sizes, your server consumes less bandwidth, which can lead to cost savings.
4. Better SEO: Faster websites tend to rank better in search engines, contributing to better visibility.
Configuring Gzip Compression in Your Setup
To set up Gzip compression in a Webpack environment, you can use the compression-webpack-plugin. This plugin creates compressed versions of your files in the build output folder. Here’s how to configure it:
First, install the plugin:
npm install compression-webpack-plugin --save-dev
Next, add the plugin to your Webpack configuration file:
// webpack.config.js const CompressionPlugin = require('compression-webpack-plugin'); module.exports = { // other configurations... plugins: [ new CompressionPlugin({ filename: '[path].gz[query]', algorithm: 'gzip', test: /\.(js|css|html|svg)$/, threshold: 10240, minRatio: 0.8, }), ], };
In this setup:
- filename
: This determines the name of the compressed file.
- algorithm
: The compression algorithm to use, which is Gzip in this case.
- test
: This regex determines which files to compress.
- threshold
: Files below this size (in bytes) will not be compressed.
- minRatio
: The minimum compression ratio for the file to be considered compressed.
Exploring Brotli Compression and Its Advantages
Brotli is a newer compression algorithm developed by Google that provides better compression ratios than Gzip. It is especially effective for text-based content such as HTML, CSS, and JavaScript. Brotli uses a combination of LZ77, Huffman coding, and a dictionary approach to achieve better compression.
Advantages of Brotli compression include:
1. Higher Compression Rates: Brotli can yield smaller file sizes compared to Gzip, making it ideal for modern web applications.
2. Improved Performance: Smaller files lead to faster loading times, improving user experience.
3. Widely Supported: Most modern browsers support Brotli, making it a viable option for production environments.
Related Article: How To Exclude Test Files In Webpack With Esbuild
Setting Up Brotli Compression for Production
To implement Brotli compression in a Webpack setup, you can use the brotli-webpack-plugin. Begin by installing it:
npm install brotli-webpack-plugin --save-dev
Then, configure it in your Webpack configuration file:
// webpack.config.js const BrotliPlugin = require('brotli-webpack-plugin'); module.exports = { // other configurations... plugins: [ new BrotliPlugin({ asset: '[path].br[query]', test: /\.(js|css|html|svg)$/, threshold: 10240, minRatio: 0.8, }), ], };
In this configuration:
- asset
: Defines the naming convention for Brotli-compressed files.
- test
: Specifies which file types to compress.
- threshold
and minRatio
are similar to the Gzip configuration, ensuring only significant files are compressed.
Minification Techniques and Their Role in Compression
Minification is the process of removing all unnecessary characters from source code without changing its functionality. This includes removing whitespace, comments, and shortening variable names. Minification plays a crucial role in compression by reducing the overall size of the files before they are compressed with Gzip or Brotli.
Common tools for minification include:
- Terser for JavaScript
- cssnano for CSS
- HTMLMinifier for HTML
In Webpack, minification can be easily integrated using the TerserWebpackPlugin for JavaScript:
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
This configuration ensures that JavaScript files are minified during the build process, which helps further reduce file sizes before compression.
Differences Between Minification and Compression
Minification and compression serve different purposes in the optimization process. While both aim to reduce file sizes, the methods and results differ:
- Minification: This process alters the actual code by removing unnecessary characters and shortening names. It makes the code less readable but does not change its functionality. The output is still a valid file that can be executed by the browser.
- Compression: This process encodes the file in a way that reduces its size for transfer over the network. It does not alter the content of the file but compresses it into a specific format (like Gzip or Brotli) for more efficient transmission.
Combining both techniques can lead to optimal performance improvements, as minified files are smaller before compression is applied.
Tree Shaking and Its Impact on Compression Results
Tree shaking is a term used in the JavaScript ecosystem, particularly with Webpack, to describe the process of removing unused code from your final bundle. This is especially relevant when using ES6 modules, as it allows for more efficient code management.
This optimization leads to smaller file sizes, which in turn enhances the effectiveness of compression. When unused code is eliminated, the remaining code is more likely to be compressed effectively, resulting in smaller Gzip or Brotli outputs.
To enable tree shaking in Webpack, make sure you have the following in your configuration:
// webpack.config.js module.exports = { mode: 'production', // Enables tree shaking automatically // other configurations... };
When the mode is set to production, Webpack automatically removes unused exports, enhancing overall performance.
Related Article: How to Use Webpack in Your Projects
Code Splitting Strategies to Enhance Compression
Code splitting is a technique that allows you to split your code into smaller chunks, which can be loaded on demand. This strategy can significantly enhance compression and improve loading times by delivering only the necessary code to the user.
Webpack provides several methods for code splitting, including:
1. Entry Points: Define multiple entry points in your configuration, allowing for separate bundles.
2. Dynamic Imports: Use import()
syntax to load modules only when needed.
Here’s an example of dynamic imports:
// Example of dynamic imports import(/* webpackChunkName: "myChunk" */ './myModule').then(module => { // Use the module });
Asset Optimization for Reduced File Sizes
Asset optimization involves several techniques to ensure that images, fonts, and other assets are as small as possible. Common practices include:
1. Image Compression: Use tools like image-webpack-loader to compress images during the build process.
npm install image-webpack-loader --save-dev
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(png|jpe?g|gif|svg)$/, use: [ { loader: 'file-loader', options: { name: '[path][name].[ext]', }, }, { loader: 'image-webpack-loader', options: { mozjpeg: { progressive: true, quality: 65, }, pngquant: { quality: [0.65, 0.80], speed: 4, }, gifsicle: { interlaced: false, }, webp: { quality: 75, }, }, }, ], }, ], }, };
2. Font Optimization: Use only the necessary font weights and styles. Consider using font-display: swap
in your CSS to improve loading behavior.
3. Remove Unused Assets: Regularly audit your assets to remove unused images and other files that may contribute to larger bundle sizes.
Performance Tuning Through Compression Methods
Performance tuning involves adjusting various settings and configurations to achieve optimal loading speeds. By leveraging compression methods effectively, you can greatly enhance your application's performance.
Using Gzip or Brotli, combined with minification and tree shaking, creates a robust strategy. Additionally, consider the following:
- Server Configuration: Ensure that your server is configured to serve compressed files. For instance, in an Nginx server, you can enable Gzip compression like this:
gzip on; gzip_types text/plain application/javascript text/css application/json; gzip_min_length 1000;
- HTTP/2: If possible, use HTTP/2, which allows multiplexing of requests, enabling faster loading of compressed assets.
File Size Reduction Techniques for Web Assets
Reducing file sizes for web assets involves various approaches:
1. Use SVGs for Graphics: SVGs are vector graphics that can be scaled without losing quality. They are usually smaller than bitmap images.
2. Optimize CSS: Use tools like PostCSS to remove unused CSS rules and minify your stylesheets.
3. Limit External Libraries: Assess the necessity of external libraries and frameworks. If you only need a small part, consider using alternatives or writing custom code.
4. Load Assets Conditionally: Use lazy loading for images and components that are not immediately necessary, which can improve initial load times.
Related Article: How to Compare Vite and Webpack for Your Project
Caching Strategies to Improve Load Times
Caching is essential for improving load times. It allows browsers to store files locally, reducing the need to fetch them on repeated visits. Implement caching strategies such as:
- Cache-Control Headers: Set appropriate headers for static assets to control how long they are cached by browsers.
Cache-Control: public, max-age=31536000, immutable
- Service Workers: Consider using service workers to cache assets programmatically, providing offline access and faster load times.
- Versioning: Use file versioning for your assets so that when changes are made, new versions are loaded, avoiding cached versions.
Configuring Multiple Compression Plugins Together
Combining multiple compression plugins can lead to optimized performance. For example, both Gzip and Brotli can be configured in Webpack. Here’s how to set them up together:
// webpack.config.js const CompressionPlugin = require('compression-webpack-plugin'); const BrotliPlugin = require('brotli-webpack-plugin'); module.exports = { plugins: [ new CompressionPlugin({ filename: '[path].gz[query]', algorithm: 'gzip', test: /\.(js|css|html|svg)$/, threshold: 10240, minRatio: 0.8, }), new BrotliPlugin({ asset: '[path].br[query]', test: /\.(js|css|html|svg)$/, threshold: 10240, minRatio: 0.8, }), ], };
This configuration allows you to serve both Gzip and Brotli compressed files, with the browser typically selecting the best option based on its capabilities.
Common Issues with Compression Plugins
When using compression plugins, you may encounter several issues:
1. Plugin Conflicts: Sometimes, different plugins may conflict with each other, leading to unexpected behavior. Ensure to test configurations thoroughly.
2. File Serving Issues: If your server is not configured to serve compressed files, users may experience problems accessing them. Verify server settings.
3. Increased Build Times: Adding multiple compression plugins can increase build times. Optimize your build setup to balance speed and output quality.
4. Browser Compatibility: Ensure that the compression formats being used are supported by the target browsers.
Enabling Compression for Production Builds
When preparing your application for production, it is crucial to enable compression. This ensures that all assets are served in their compressed forms for optimal performance. In Webpack, this can be set up in the production configuration:
// webpack.config.js const CompressionPlugin = require('compression-webpack-plugin'); module.exports = { mode: 'production', plugins: [ new CompressionPlugin({ algorithm: 'gzip', test: /\.(js|css|html|svg)$/, threshold: 10240, deleteOriginalAssets: false, }), ], };
This setup compresses files during the production build process, ensuring that optimized assets are deployed.
Related Article: How to Fix webpack Build Cannot Find Name ‘vi’ Vitest
Impact of Compression on Loading Time
Compression has a direct impact on loading times. By significantly reducing the size of files served over the network, compression allows for faster data transfer, which leads to quicker page loads.
In many cases, users may see load times decrease from several seconds to fractions of a second, especially on slower connections. The overall performance improvement not only enhances user experience but can also lead to better retention rates and lower bounce rates.
Additional Resources
- Best Compression Plugins for Webpack