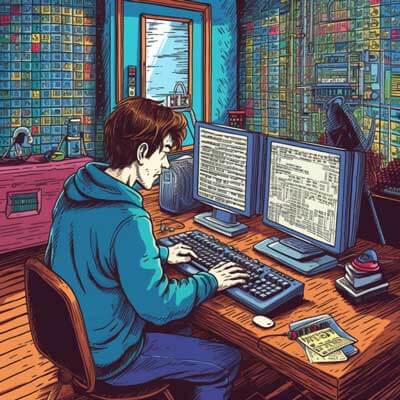
Table of Contents
Overview of the Django Webpack Loader
Django Webpack Loader is a Django app that helps manage your front-end assets, allowing you to integrate Webpack into your Django projects seamlessly. This tool is important because it bridges the gap between Django's static file handling and Webpack's useful module bundling capabilities. By using Django Webpack Loader, you can take advantage of Webpack's features, such as code splitting, tree shaking, and hot module replacement, while still leveraging Django's built-in static file management.
When combined, Django and Webpack create a robust environment for developing modern web applications. The loader simplifies the process of linking your bundled assets to your Django templates, ensuring that your application remains organized and maintainable.
Related Article: How To Define A Plugin In Webpack
Setting Up Your Django Project for Asset Management
To get started with Django Webpack Loader, you need to set up your Django project to handle asset management. This process involves installing the necessary packages and configuring your settings.
1. First, create a new Django project if you haven’t already:
django-admin startproject myproject cd myproject
2. Install Django Webpack Loader and Webpack:
pip install django-webpack-loader npm install --save-dev webpack webpack-cli
3. Add webpack_loader
to your INSTALLED_APPS
in settings.py
:
# settings.py INSTALLED_APPS = [ ... 'webpack_loader', ]
4. Create a new directory for your Webpack configuration files and assets:
mkdir assets cd assets
5. Create a webpack.config.js
file for your Webpack setup:
// webpack.config.js const path = require('path'); module.exports = { entry: './assets/js/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, mode: 'development', };
6. Update your Django settings to configure Webpack Loader:
# settings.py WEBPACK_LOADER = { 'DEFAULT': { 'BUNDLE_DIR_NAME': 'assets/dist/', # path to your output directory 'STATS_FILE': os.path.join(BASE_DIR, 'webpack-stats.json'), } }
7. Create a webpack-stats.json
file in your project root to manage bundle stats:
{ "status": "done", "chunks": { "main": { "files": ["bundle.js"] } } }
Now, your Django project is set up to manage assets with Webpack.
Configuring the Development Server for Optimal Performance
To improve your development experience, configure Webpack to run a development server that serves your assets. This setup enables features like hot module replacement and faster rebuild times.
1. Install the Webpack Dev Server:
npm install --save-dev webpack-dev-server
2. Update your webpack.config.js
file to include the Dev Server configuration:
// webpack.config.js const path = require('path'); module.exports = { entry: './assets/js/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', publicPath: '/assets/dist/', }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, }, mode: 'development', };
3. Add a script to your package.json
to run the Webpack Dev Server:
{ "scripts": { "start": "webpack serve --open" } }
4. Run the development server:
npm start
The server will open in your browser at http://localhost:9000
, where you can see your application running with live updates.
Integrating JavaScript Bundling with Django
Integrating JavaScript bundling with Django involves linking your bundled assets in your templates. Use the Webpack Loader to include the generated JavaScript files.
1. In your Django template (e.g., index.html
), load the bundle:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Django App</title> {% load webpack_loader %} {% render_bundle 'main' %} </head> <body> <h1>Hello, Django with Webpack!</h1> </body> </html>
2. Ensure that your JavaScript entry file exists at assets/js/index.js
:
// assets/js/index.js console.log("JavaScript is bundled and running!");
When you navigate to your Django app in the browser, the bundled JavaScript should load correctly.
Related Article: How to Use the Copy Webpack Plugin
Handling Static Files in Django Using Asset Management Tools
Django's static file system can be extended with asset management tools like Webpack. This setup allows for better organization and optimization of static assets.
1. Use Webpack to manage CSS and images along with JavaScript. Update your webpack.config.js
to handle styles:
// webpack.config.js const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { ... module: { rules: [ { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'], }, ], }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ], };
2. Create a CSS file for your styles at assets/css/styles.css
:
/* assets/css/styles.css */ body { background-color: #f0f0f0; font-family: Arial, sans-serif; }
3. Import the CSS file in your JavaScript entry file:
// assets/js/index.js import '../css/styles.css'; console.log("JavaScript and CSS are bundled and running!");
4. Update your webpack-stats.json
to include the CSS file:
{ "status": "done", "chunks": { "main": { "files": ["bundle.js", "styles.css"] } } }
Now, both JavaScript and CSS are managed through Webpack, making it easier to maintain your static files.
Managing CSS and JavaScript Together
Combining CSS and JavaScript management under Webpack allows for a unified approach to handling your assets. This setup promotes modularity and code organization.
1. Create separate directories for JavaScript and CSS assets:
mkdir -p assets/js assets/css
2. Update your webpack.config.js
to manage both file types:
// webpack.config.js const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { entry: { main: ['./assets/js/index.js', './assets/css/styles.css'], }, output: { ... }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'], }, ], }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ], };
3. Ensure your HTML template includes both the JavaScript and CSS bundles:
<head> ... {% render_bundle 'main' %} </head>
Implementing Hot Module Replacement
Hot Module Replacement (HMR) enables live updates of modules without a full browser refresh. This feature significantly improves the development experience.
1. Ensure your webpack.config.js
has the HMR plugin configured:
// webpack.config.js const webpack = require('webpack'); module.exports = { ... plugins: [ new webpack.HotModuleReplacementPlugin(), new MiniCssExtractPlugin({ filename: '[name].css', }), ], };
2. Update your devServer
configuration to enable HMR:
// webpack.config.js devServer: { ... hot: true, },
3. Modify your entry point in index.js
to enable HMR:
if (module.hot) { module.hot.accept(); }
4. Run your development server:
npm start
Now, when you make changes to your JavaScript or CSS files, the updates will reflect in the browser without a full page reload.
Code Splitting Techniques
Code splitting allows you to break your JavaScript bundles into smaller chunks, loading only what's necessary for the initial render. This technique improves load times and overall performance.
1. Update your webpack.config.js
to enable code splitting:
// webpack.config.js module.exports = { ... optimization: { splitChunks: { chunks: 'all', }, }, };
2. Use dynamic imports in your JavaScript files to split code:
// assets/js/index.js function loadModule() { import('./module.js').then(module => { module.default(); }); } loadModule();
3. Create the module.js
file:
// assets/js/module.js export default function() { console.log("Dynamically loaded module!"); }
When the loadModule
function is called, the module will be loaded on demand, reducing the initial bundle size.
Related Article: How to Choose Between Gulp and Webpack
Optimizing Builds for Production
To prepare your application for production, you need to optimize your Webpack build. This process includes minifying your JavaScript and CSS, removing unused code, and more.
1. Update your webpack.config.js
for production mode:
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { mode: 'production', optimization: { minimize: true, minimizer: [new TerserPlugin()], splitChunks: { chunks: 'all', }, }, };
2. Run the production build:
npx webpack --config webpack.config.js --mode production
This command generates an optimized build in the dist
directory, ready for deployment.
Using Tree Shaking
Tree shaking is a technique to eliminate dead code from your bundles. By using ES6 module syntax, Webpack can identify and remove unused code.
1. Ensure you are using ES6 imports and exports in your modules:
// assets/js/module.js export const usedFunction = () => { console.log("This function is used."); }; export const unusedFunction = () => { console.log("This function is unused."); };
2. Import only the necessary functions in your entry file:
// assets/js/index.js import { usedFunction } from './module'; usedFunction();
3. Set the mode to production in webpack.config.js
to enable tree shaking:
// webpack.config.js module.exports = { mode: 'production', ... };
When you build your application, Webpack will remove the unusedFunction
, reducing the bundle size.
CSS Preprocessing Methods
CSS preprocessing allows you to write styles in a more maintainable and modular way. Tools like SASS or LESS can be integrated into your Webpack setup.
1. Install SASS and the necessary loaders:
npm install --save-dev sass sass-loader
2. Update your webpack.config.js
to handle SASS files:
// webpack.config.js module.exports = { ... module: { rules: [ { test: /\.scss$/, use: [MiniCssExtractPlugin.loader, 'css-loader', 'sass-loader'], }, ], }, };
3. Create a SASS file at assets/scss/styles.scss
:
/* assets/scss/styles.scss */ $primary-color: #3498db; body { background-color: $primary-color; font-family: Arial, sans-serif; }
4. Import the SASS file into your JavaScript entry file:
// assets/js/index.js import '../scss/styles.scss'; console.log("SASS is compiled and applied!");
Now, your Django project uses SASS for styling, which enhances your CSS workflow.
Module Federation
Module Federation allows you to share code between multiple applications dynamically. This feature is beneficial for micro-frontend architectures.
1. Update your webpack.config.js
to set up module federation:
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin'); module.exports = { ... plugins: [ new ModuleFederationPlugin({ name: 'app1', filename: 'remoteEntry.js', exposes: { './Button': './src/Button', }, shared: ['react', 'react-dom'], }), ], };
2. Use the Button
component in another application:
import('app1/Button').then(Button => { // Use the Button component });
This approach enables code sharing across different applications, promoting reusability.
Related Article: How to Use Webpack CopyPlugin for Asset Management
Common Issues When Using Asset Management Tools
Several issues can arise when integrating asset management tools with Django. Here are some common problems and their solutions:
1. Webpack Not Finding Assets: Ensure your BUNDLE_DIR_NAME
in the Webpack Loader settings points to the correct output directory.
2. Static Files Not Updating: If you’re not seeing updates in your assets, check your browser cache or try a hard refresh.
3. Hot Module Replacement Not Working: Ensure the HMR plugin is correctly configured in your Webpack config, and that your server is running.
4. Build Errors: Check the console for errors during the Webpack build process. Ensure all required loaders and plugins are installed.
Best Practices for Asset Management in Django Projects
Follow these best practices to maintain a clean and efficient asset management workflow:
1. Organize Files: Keep your JavaScript, CSS, and image files in separate directories to enhance clarity.
2. Use ES6 Modules: Leverage ES6 module syntax to take advantage of tree shaking and modular code.
3. Optimize for Production: Always configure Webpack for production builds to minimize file sizes and improve load times.
4. Regularly Update Dependencies: Keep your Webpack and related dependencies up to date to benefit from the latest features and fixes.
5. Document Your Setup: Clearly document your asset management setup to assist future developers working on the project.
Advanced Configuration Settings for Asset Management in Django
Advanced configurations can enhance your asset management setup further. Consider the following options:
1. Custom Output Filenames: Change the output filenames in webpack.config.js
to include hashes for cache busting:
output: { filename: '[name].[contenthash].js', }
2. Environment Variables: Use environment variables to differentiate between development and production environments:
const isProduction = process.env.NODE_ENV === 'production'; module.exports = { mode: isProduction ? 'production' : 'development', };
3. Linting and Formatting: Integrate tools like ESLint and Prettier to maintain code quality:
npm install --save-dev eslint prettier
4. Source Maps: Enable source maps for easier debugging during development:
devtool: 'source-map',
Additional Resources
- Managing CSS and JavaScript with Webpack