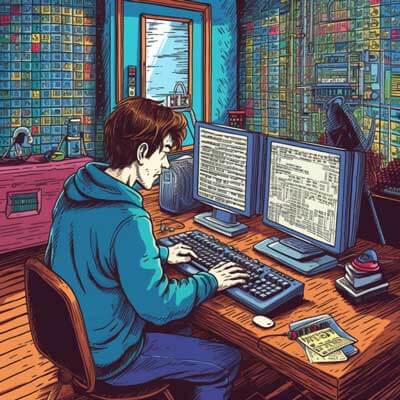
Table of Contents
Environment variables allow developers to store configuration settings outside of their application code. This approach enhances security and flexibility when deploying applications across different environments like development, testing, and production. Webpack, a popular module bundler, supports environment variables to customize the build process. By using environment variables, developers can control various aspects of their builds, such as API endpoints, feature flags, and other settings that may change based on the environment.
Setting Environment Variables in Webpack Configuration
Environment variables can be set directly in your Webpack configuration file. You can access these variables in your configuration and use them to alter settings based on the environment.
For example, you can set an environment variable before running your Webpack build:
export NODE_ENV=production
Then, within your webpack.config.js
, you can access this variable:
// webpack.config.jsconst path = require('path');module.exports = { mode: process.env.NODE_ENV || 'development', entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }};
In this case, Webpack will use 'production' as the mode if the NODE_ENV
variable is set.
Related Article: How to Configure SVGR with Webpack
Using DefinePlugin for Environment Variables
DefinePlugin is a built-in Webpack plugin that allows you to create global constants which can be configured at compile time. This is useful for defining environment variables.
To use DefinePlugin, first, import it at the top of your Webpack configuration file:
// webpack.config.jsconst webpack = require('webpack');module.exports = { // ... other configurations plugins: [ new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify(process.env.NODE_ENV || 'development'), 'process.env.API_URL': JSON.stringify(process.env.API_URL || 'http://localhost:3000') }) ]};
Here, process.env.NODE_ENV
and process.env.API_URL
will be replaced with their respective values during the build process.
Integrating dotenv with Webpack for Environment Variables
Dotenv is a popular library that loads environment variables from a .env
file into process.env
. This is particularly useful for managing sensitive information like API keys. To use dotenv with Webpack, follow these steps:
1. Install the dotenv package:
npm install dotenv --save
2. Create a .env
file in your project root:
NODE_ENV=productionAPI_URL=https://api.example.com
3. Load the variables from the .env
file in your Webpack configuration:
// webpack.config.jsconst path = require('path');const webpack = require('webpack');require('dotenv').config();module.exports = { // ... other configurations plugins: [ new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify(process.env.NODE_ENV), 'process.env.API_URL': JSON.stringify(process.env.API_URL) }) ]};
Now, the environment variables defined in your .env
file will be accessible during the build.
Accessing Environment Variables in Your Code
To access the defined environment variables in your application code, simply refer to them using process.env
. For example:
// src/index.jsconsole.log('Environment:', process.env.NODE_ENV);console.log('API URL:', process.env.API_URL);
When your application runs, it will output the current environment and the API URL based on the values defined in your Webpack configuration.
Related Article: How to Set Webpack Target to Node.js
Defining Custom Environment Variables in Webpack
Custom environment variables can be defined similarly to standard ones. You can use DefinePlugin to set any variable you need. For example:
// webpack.config.jsconst webpack = require('webpack');module.exports = { // ... other configurations plugins: [ new webpack.DefinePlugin({ 'process.env.CUSTOM_VAR': JSON.stringify('my-custom-value') }) ]};
This allows you to create variables that can be used in your application code:
// src/index.jsconsole.log('Custom Variable:', process.env.CUSTOM_VAR);
This outputs "my-custom-value" when the application is built.
Using NODE_ENV for Environment Configuration
NODE_ENV is a convention used in many Node.js applications to determine the environment in which the application is running. It typically has values such as 'development', 'production', or 'test'. Webpack can use this variable to optimize builds for different environments.
When set to 'production', Webpack enables various optimizations by default, such as minimizing the output code. You can set NODE_ENV in your terminal before running Webpack:
export NODE_ENV=production
In your Webpack configuration, you can use NODE_ENV to conditionally apply settings:
// webpack.config.jsmodule.exports = { mode: process.env.NODE_ENV || 'development', optimization: { minimize: process.env.NODE_ENV === 'production' }};
This ensures that the application is built optimally based on the environment.
Differences Between Development and Production Modes
Development mode is focused on providing a fast feedback loop for developers. It includes features like source maps, detailed error messages, and no code minification. This mode allows developers to debug their code more easily.
Production mode, on the other hand, is optimized for performance. It minimizes the output bundle size, removes unused code, and enhances performance by optimizing the code for execution. Webpack's default behavior changes based on the mode:
// webpack.config.jsmodule.exports = { mode: 'development', // or 'production' // Development-specific configurations devtool: process.env.NODE_ENV === 'production' ? false : 'source-map'};
This example disables source maps in production mode for better performance.
Changing Configuration Based on Environment Variables
Different configurations can be applied based on environment variables to tailor the build process. By checking the value of an environment variable, you can load different settings:
// webpack.config.jsconst isProduction = process.env.NODE_ENV === 'production';module.exports = { // Common configuration output: { filename: isProduction ? 'bundle.min.js' : 'bundle.js', path: path.resolve(__dirname, 'dist') }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: isProduction ? ['@babel/preset-env'] : ['@babel/preset-env', 'babel-plugin-transform-remove-console'] } } } ] }};
In this case, the output filename and Babel presets are adjusted based on whether the build is for production or development.
Related Article: How to Use Django Webpack Loader with Webpack
Using Webpack CLI to Set Environment Variables
Webpack CLI allows setting environment variables directly from the command line. This is useful for one-off builds or when you want to override environment variables without modifying the configuration files.
You can set environment variables inline when running Webpack, like this:
NODE_ENV=production API_URL=https://api.example.com npx webpack --config webpack.config.js
This command sets the NODE_ENV
and API_URL
environment variables for that specific run, allowing you to customize the build without changing the configuration file.
Working with Multiple Environment Variables in Webpack
Managing multiple environment variables can be done efficiently by defining them in your .env
file or directly in your Webpack config. Using the dotenv
package helps to keep the configuration organized.
Here's an example of a .env
file with multiple variables:
NODE_ENV=productionAPI_URL=https://api.example.comANOTHER_VAR=some_value
You can load these variables similarly in your Webpack configuration:
// webpack.config.jsrequire('dotenv').config();module.exports = { plugins: [ new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify(process.env.NODE_ENV), 'process.env.API_URL': JSON.stringify(process.env.API_URL), 'process.env.ANOTHER_VAR': JSON.stringify(process.env.ANOTHER_VAR) }) ]};
Accessing these variables in your code is the same as before:
console.log('Another Variable:', process.env.ANOTHER_VAR);
This enables you to control various aspects of your application through environment variables easily.
A Practical Example of Environment Variables in Webpack
Consider a simple web application that requires an API URL that changes based on the environment. You can set this up as follows:
1. Create a .env
file:
NODE_ENV=developmentAPI_URL=http://localhost:3000
2. In your webpack.config.js
, load and define the environment variables:
// webpack.config.jsconst webpack = require('webpack');require('dotenv').config();module.exports = { mode: process.env.NODE_ENV || 'development', plugins: [ new webpack.DefinePlugin({ 'process.env.API_URL': JSON.stringify(process.env.API_URL) }) ]};
3. Access the API URL in your application code:
// src/index.jsfetch(process.env.API_URL + '/data') .then(response => response.json()) .then(data => console.log(data));
4. Run your build:
NODE_ENV=development npx webpack
Additional Resources
- Setting Up Environment Variables in Webpack