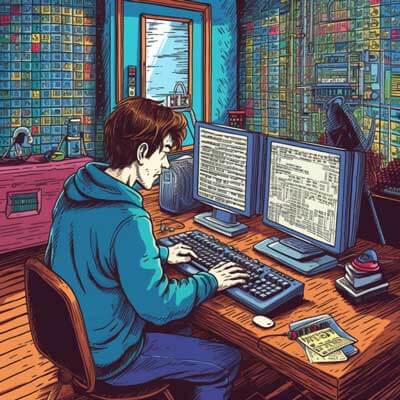
Table of Contents
Overview of Extract Text Plugin Usage
The Extract Text Webpack Plugin is a tool designed to extract text from your JavaScript files into separate files. This is especially useful for handling CSS and other text-based assets in a modular way. The plugin allows you to keep your stylesheets separate from your JavaScript, improving load times and enabling better caching strategies.
To get started, the first step is to install the plugin. You can do this using npm:
npm install --save-dev extract-text-webpack-plugin
Then, integrate the plugin into your Webpack configuration file:
const ExtractTextPlugin = require('extract-text-webpack-plugin'); module.exports = { // other configurations... module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] };
In this example, CSS files will be processed by the css-loader
and extracted into a single styles.css
file.
Related Article: How to Set Webpack Target to Node.js
Code Splitting with Extract Text Plugin
Code splitting is a technique that allows you to break your application into smaller chunks, which can be loaded on demand. This reduces the initial load time of your application. The Extract Text Plugin can be used in conjunction with code splitting to ensure that your CSS is also split accordingly.
To implement code splitting, you can define multiple entry points in your Webpack configuration. Here’s how you can achieve this:
module.exports = { entry: { app: './src/index.js', vendor: './src/vendor.js' }, output: { filename: '[name].bundle.js', path: path.resolve(__dirname, 'dist') }, module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new ExtractTextPlugin('[name].css') ] };
Each entry point will generate its own CSS file. For example, app
will produce app.css
and vendor
will produce vendor.css
.
Bundle Optimization Techniques
Bundle optimization refers to the strategies used to reduce the size of your final output files, improving load times. Using the Extract Text Plugin can aid in this process. You can implement techniques such as minification and compression of your extracted CSS.
To minify CSS, you can use css-minimizer-webpack-plugin
alongside the Extract Text Plugin. Here’s a simple setup:
npm install --save-dev css-minimizer-webpack-plugin
Then, modify your Webpack configuration:
const CssMinimizerPlugin = require('css-minimizer-webpack-plugin'); module.exports = { // other configurations... optimization: { minimize: true, minimizer: [ `...`, // This includes the default TerserPlugin new CssMinimizerPlugin(), ], }, plugins: [ new ExtractTextPlugin('styles.css') ] };
The resulting CSS will be minified, reducing file size and improving load times.
Asset Management Strategies
Managing assets effectively is crucial for the performance of a web application. The Extract Text Plugin helps in organizing CSS files, making it easier to manage versions and updates. Using the plugin, you can extract CSS into separate files, which can then be cached by the browser.
A common strategy is to create a dedicated folder for your assets. Here’s a sample configuration:
module.exports = { output: { filename: '[name].bundle.js', path: path.resolve(__dirname, 'dist/assets') }, plugins: [ new ExtractTextPlugin({ filename: 'styles/[name].css' }) ] };
With this setup, your styles will be placed in the dist/assets/styles
directory, making it more organized.
Related Article: How to Use the Webpack CLI Option -d
CSS Extraction Process
The process of extracting CSS using the Extract Text Plugin involves configuring Webpack to process your CSS files. This is done through the rules
array in your Webpack configuration file. When Webpack encounters a CSS file, it applies the configured loaders.
Here’s a step-by-step example:
1. Install the necessary loaders.
npm install --save-dev css-loader style-loader
2. Update your Webpack configuration:
module.exports = { module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] };
In this configuration, CSS files will be processed using css-loader
and extracted into styles.css
while being loaded by style-loader
.
Dynamic Imports and Extract Text Plugin Integration
Dynamic imports allow you to load modules at runtime rather than at build time. This can be combined with the Extract Text Plugin to load styles dynamically as needed.
To implement dynamic imports for CSS, you can use the following configuration:
module.exports = { module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] }; // Dynamic import import('./styles.css').then(() => { console.log('Styles loaded'); });
When the dynamic import is executed, the styles will be extracted and applied.
Tree Shaking Benefits
Tree shaking is a technique used to eliminate dead code from your final bundle. The Extract Text Plugin can facilitate tree shaking by ensuring that only the necessary CSS is extracted. When combined with ES6 module syntax, unused styles can be automatically removed during the build process.
To leverage tree shaking, ensure your project uses ES6 modules and configure Webpack:
module.exports = { mode: 'production', module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] };
Unused CSS will be eliminated, leading to smaller and more efficient CSS files.
Hot Module Replacement
Hot Module Replacement (HMR) allows modules to be updated in the browser without a full reload. The Extract Text Plugin does not support HMR out-of-the-box, but you can integrate it with additional configurations.
To set up HMR with the Extract Text Plugin, follow these steps:
1. Install the necessary packages:
npm install --save-dev webpack-dev-server
2. Update your Webpack configuration:
const webpack = require('webpack'); module.exports = { devServer: { hot: true }, module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: 'css-loader' }) } ] }, plugins: [ new webpack.HotModuleReplacementPlugin(), new ExtractTextPlugin('styles.css') ] };
With this setup, changes to CSS files will update in the browser without a full page reload.
Related Article: How to Use Environment Variables in Webpack
Plugin Architecture Features of Extract Text Plugin
The Extract Text Plugin follows a specific architecture that allows it to interact seamlessly with Webpack. It uses a combination of loaders to process files and extract them into separate files. The plugin architecture is modular, enabling developers to customize its behavior.
Key features include:
- Customization of output file names.
- Support for multiple file types.
- Integration with other Webpack plugins for optimization and minification.
To customize the output, you can specify a filename pattern:
new ExtractTextPlugin({ filename: '[name].[contenthash].css' });
This feature allows better caching by generating unique file names based on content.
Module Federation
Module Federation enables sharing of code between different applications, allowing for better collaboration and reduced redundancy. The Extract Text Plugin can be integrated into a module federation setup, ensuring that CSS is handled correctly across different applications.
Here’s how you can set it up:
1. Install necessary packages:
npm install --save-dev @module-federation/webpack-module-federation
2. Configure your Webpack:
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin'); module.exports = { plugins: [ new ModuleFederationPlugin({ name: 'app', filename: 'remoteEntry.js', exposes: { './Component': './src/Component', } }), new ExtractTextPlugin('styles.css') ] };
This setup allows sharing of components while managing CSS extraction effectively.
Improving Build Performance with Extract Text Plugin
Improving build performance is a common goal in Webpack configurations. The Extract Text Plugin can contribute to this by reducing the size of the output files and streamlining the loading process.
To enhance performance, consider using the plugin in conjunction with caching:
module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, plugins: [ new ExtractTextPlugin({ filename: '[name].css', disable: process.env.NODE_ENV === 'development', }), ], };
This configuration ensures that CSS files are split and cached appropriately, leading to faster builds.
Configuring the Extract Text Plugin
Proper configuration of the Extract Text Plugin is crucial for its functionality. The plugin accepts various options that can be adjusted based on project requirements.
Here’s a basic configuration example:
new ExtractTextPlugin({ filename: 'styles/[name].css', allChunks: true, });
- filename
: Defines the output file name and location.
- allChunks
: When set to true, extracts CSS from all chunks, not just the initial ones.
Modify these options to fit the needs of your project.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Advantages of Using Extract Text Plugin
The Extract Text Plugin offers several advantages, including:
- Separation of CSS from JavaScript, improving load times.
- Better caching strategies due to separate file outputs.
- Support for minification and optimization processes.
These benefits contribute to a more organized and efficient build process.
Extracting CSS from Multiple Entry Points with Extract Text Plugin
When dealing with multiple entry points, extracting CSS for each entry point is essential. The Extract Text Plugin can handle this by defining separate output patterns.
Here’s an example configuration:
module.exports = { entry: { app: './src/app.js', admin: './src/admin.js' }, plugins: [ new ExtractTextPlugin({ filename: '[name].css' }) ] };
With this setup, app.js
generates app.css
, while admin.js
generates admin.css
.
Source Map Handling
Source maps are essential for debugging, allowing you to trace errors back to the original source code. The Extract Text Plugin can be configured to generate source maps for your CSS.
To enable source maps, configure the plugin as follows:
new ExtractTextPlugin({ filename: 'styles.css', disable: process.env.NODE_ENV === 'development', sourceMap: true, });
This configuration generates source maps, making it easier to debug your CSS styles.
Deprecation Status of Extract Text Plugin
The Extract Text Plugin is considered deprecated in favor of the MiniCssExtractPlugin. While it is still functional, new projects are encouraged to use the MiniCssExtractPlugin for better performance and support.
The MiniCssExtractPlugin has similar functionality but is optimized for use with the latest versions of Webpack.
Related Article: How to Bypass a Router with Webpack Proxy
Alternatives to Extract Text Plugin
While the Extract Text Plugin has been widely used, there are alternatives that offer similar functionality. The most notable alternative is the MiniCssExtractPlugin.
To use MiniCssExtractPlugin, follow these steps:
1. Install the plugin:
npm install --save-dev mini-css-extract-plugin
2. Update your Webpack configuration:
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { module: { rules: [ { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'] } ] }, plugins: [ new MiniCssExtractPlugin({ filename: 'styles.css' }) ] };
This setup provides similar functionality with improved performance and modern features.
Integrating Extract Text Plugin with PostCSS
PostCSS is a tool used to transform styles with JavaScript plugins. The Extract Text Plugin can be integrated with PostCSS to enhance your CSS processing capabilities.
Here’s how to set it up:
1. Install PostCSS and the necessary plugins:
npm install --save-dev postcss postcss-loader
2. Update your Webpack configuration:
module.exports = { module: { rules: [ { test: /\.css$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: [ 'css-loader', 'postcss-loader' ] }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] };
This configuration will process CSS files with PostCSS after they have been extracted.
Extract Text Plugin vs MiniCssExtractPlugin
The Extract Text Plugin and MiniCssExtractPlugin serve similar purposes, but they differ in usability and performance. The MiniCssExtractPlugin is the recommended choice for modern Webpack projects due to its optimizations and better integration.
For example, MiniCssExtractPlugin allows for hot reloading of CSS files:
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { module: { rules: [ { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'] } ] }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css' }) ] };
MiniCssExtractPlugin should be preferred for new projects or when upgrading existing projects.
Using Extract Text Plugin with SCSS
Extracting styles from SCSS files is similar to handling CSS files. The Extract Text Plugin can be used to process SCSS files using the appropriate loaders.
Here’s how to configure it:
1. Install the necessary loaders:
npm install --save-dev sass-loader node-sass
2. Update your Webpack configuration:
module.exports = { module: { rules: [ { test: /\.scss$/, use: ExtractTextPlugin.extract({ fallback: 'style-loader', use: [ 'css-loader', 'sass-loader' ] }) } ] }, plugins: [ new ExtractTextPlugin('styles.css') ] };
This configuration enables the processing of SCSS files, extracting them into a CSS file.
Related Article: How to Use ESLint Webpack Plugin for Code Quality
Troubleshooting Extract Text Plugin Issues
Common issues with the Extract Text Plugin can arise during configuration or while processing files. Here are some troubleshooting steps:
1. Ensure all dependencies are installed: Missing loaders or plugins can cause issues. Verify that all required packages are installed.
2. Check Webpack version compatibility: Ensure that the version of the Extract Text Plugin is compatible with your Webpack version.
3. Review Webpack configuration: Errors in the configuration file can lead to unexpected behavior. Double-check the syntax and options used.
4. Use console logs for debugging: Adding console logs in your configuration can help identify where the process is failing.
5. Refer to the official documentation: Consult the Extract Text Plugin documentation for specific issues and solutions.
Following these steps can help resolve common problems encountered when using the Extract Text Plugin.
Additional Resources
- Extracting CSS from Multiple Entry Points
- Alternatives to Extract Text Webpack Plugin
- Difference between Extract Text Webpack Plugin and MiniCssExtractPlugin