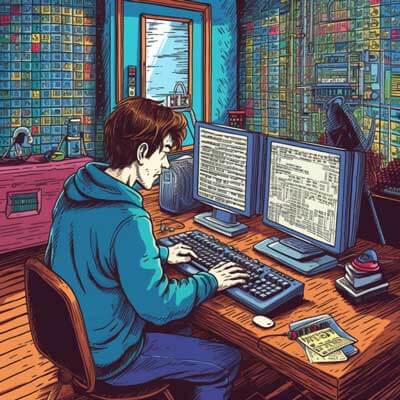
Table of Contents
Introduction
The forEach method is a built-in function in JavaScript that allows you to iterate over an array and perform a specific action for each element. It provides a simple and convenient way to loop through the elements of an array without the need for traditional for loops. In this answer, we will explore how to use the forEach method in JavaScript and discuss some best practices and alternative ideas.
Related Article: How to Get Query String Values in Javascript
Syntax
The syntax for the forEach method is as follows:
array.forEach(callback(currentValue, index, array), thisArg)
The forEach method takes in a callback function as an argument, which will be invoked for each element in the array. The callback function can take up to three parameters:
- currentValue: The value of the current element being processed.
- index (optional): The index of the current element being processed.
- array (optional): The array that the forEach method was called upon.
The thisArg parameter (optional) allows you to specify the value to be used as "this" when executing the callback function.
Iterating over an Array
To use the forEach method, you simply call it on an array and provide a callback function. The callback function will be executed for each element in the array in ascending order.
Here's an example that demonstrates how to use forEach to iterate over an array and print each element:
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
Output:
1 2 3 4 5
In the example above, the callback function takes a single parameter (number) which represents the current element in the array. The function then logs the value of each element to the console.
Breaking Out of a forEach Loop
The forEach method does not support breaking out of the loop like the traditional for loop or other looping constructs. Once the forEach loop starts, it will continue to iterate over all the elements in the array, regardless of any return statements or exceptions thrown within the callback function.
However, there are a couple of ways to achieve a similar effect. One approach is to use the Array.some method within the forEach loop to stop the iteration when a certain condition is met. The Array.some method returns true if at least one element in the array satisfies the provided testing function.
Here's an example that demonstrates how to break out of a forEach loop using the Array.some method:
const numbers = [1, 2, 3, 4, 5]; let shouldBreak = false; numbers.forEach(function(number) { if (number === 3) { shouldBreak = true; return; // No need to continue iterating } console.log(number); return !shouldBreak; // Stop the forEach loop if shouldBreak is true });
Output:
1 2
In the example above, the callback function checks if the current element is equal to 3. If it is, the shouldBreak
variable is set to true and the return statement immediately exits the callback function. The !shouldBreak
statement is used to stop the forEach loop if shouldBreak
is true, effectively breaking out of the loop.
Alternatively, you can use a traditional for loop or a for...of loop if you need more control over the looping process and the ability to break out of the loop.
Related Article: Top Algorithms Implemented in JavaScript
Best Practices
When using the forEach method in JavaScript, consider the following best practices:
1. Use arrow functions: Arrow functions provide a more concise syntax and lexical scoping of the "this" value. They are especially useful when the callback function is short and does not require its own "this" context.
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(number => console.log(number));
2. Avoid using forEach for asynchronous operations: The forEach method does not wait for asynchronous operations to complete, which can lead to unexpected behavior. Use other looping constructs, such as for...of or for loops, when dealing with asynchronous operations.
3. Consider using map or reduce: If you need to transform or aggregate the elements of an array, the map or reduce methods may be more appropriate than forEach. These methods provide functional programming paradigms that can lead to more readable and maintainable code.