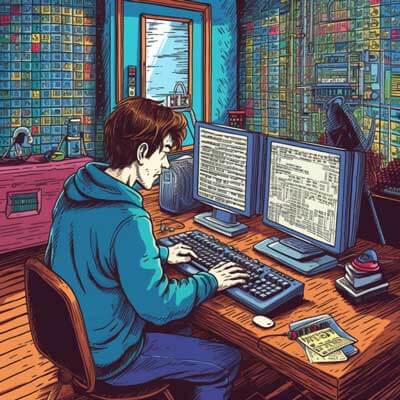
Table of Contents
Introduction to Getline in C++
Getline is a commonly used function in C++ that allows you to read a line of text from an input stream. It is part of the
library and provides a convenient way to handle input that spans multiple lines. Getline is particularly useful when reading user input from the console or reading from a file.
Here's a simple example that demonstrates the usage of Getline:
#include <iostream> using namespace std; int main() { string line; cout << "Enter a line of text: "; getline(cin, line); cout << "You entered: " << line << endl; return 0; }
In this example, the program prompts the user to enter a line of text, and then uses Getline to read the entire line, including any spaces or special characters. The line is then stored in the variable line
and displayed back to the user.
Related Article: How to Install, Configure and Use the JSON Server
How to Read a Line of Text in C++ Using Getline
To read a line of text using Getline, you need to provide two arguments: the input stream from which you want to read the line, and the string variable in which you want to store the line.
Here's the syntax for using Getline:
getline(input_stream, variable);
- input_stream
: This is the input stream from which you want to read the line. It can be a file stream (ifstream
), the standard input stream (cin
), or any other input stream object.
- variable
: This is the string variable in which you want to store the line of text. Make sure you declare the variable before calling Getline.
Let's take a look at an example that reads multiple lines of text from the user:
#include <iostream> using namespace std; int main() { string line; cout << "Enter multiple lines of text. Press Enter to stop." << endl; while (getline(cin, line)) { cout << "You entered: " << line << endl; } return 0; }
In this example, the program continuously reads lines of text from the user until the user presses Enter without typing anything. Each line is then displayed back to the user.
Getline Syntax and Parameters
The syntax of Getline is straightforward, as mentioned earlier. However, there are a few additional parameters that can be used to customize its behavior.
Here's the extended syntax for Getline:
getline(input_stream, variable, delimiter);
- input_stream
: This is the input stream from which you want to read the line.
- variable
: This is the string variable in which you want to store the line of text.
- delimiter
(optional): This is the character that marks the end of a line. By default, it is set to the newline character ('\n'
). You can specify a different delimiter if needed.
Let's see an example that uses a custom delimiter:
#include <iostream> using namespace std; int main() { string line; cout << "Enter multiple lines of text. Use '#' to end the input." << endl; while (getline(cin, line, '#')) { cout << "You entered: " << line << endl; } return 0; }
In this example, the program reads lines of text from the user until the user types the '#' character, which is used as the delimiter. The delimiter is not included in the stored line.
Best Practices of Using Getline in C++
When using Getline in C++, there are a few best practices that can help you write clean and error-free code. Let's explore some of these practices:
1. Always declare the string variable before using Getline.
2. Check if the Getline operation was successful before using the input.
3. Handle empty lines or lines with only whitespace appropriately.
4. Clear the input stream before using Getline to discard any extra characters or newline characters.
Let's see an example that demonstrates these best practices:
#include <iostream> using namespace std; int main() { string line; cout << "Enter a line of text: "; if (getline(cin, line)) { if (!line.empty()) { cout << "You entered: " << line << endl; } else { cout << "Input is empty." << endl; } } else { cout << "Failed to read input." << endl; } cin.ignore(numeric_limits<streamsize>::max(), '\n'); // clear input stream return 0; }
In this example, the program checks if the Getline operation was successful by using the result of the function call in an if statement. It then checks if the line is empty or not before displaying the input. Finally, it clears the input stream using cin.ignore()
to remove any remaining characters.
Related Article: How to Ignore Case Sensitivity with Regex (Case Insensitive)
Real World Use Case: User Input Validation with Getline
Getline can be used effectively for user input validation, ensuring that the input meets certain criteria before proceeding. Let's take the example of validating a user's age:
#include <iostream> #include <sstream> using namespace std; bool validateAge(const string& input) { stringstream ss(input); int age; if (ss >> age && age >= 18 && age <= 99) { return true; } return false; } int main() { string ageInput; cout << "Enter your age: "; while (getline(cin, ageInput)) { if (validateAge(ageInput)) { cout << "Valid age entered: " << ageInput << endl; break; } else { cout << "Invalid age. Please enter a valid age between 18 and 99: "; } } return 0; }
In this example, the program continuously prompts the user to enter their age using Getline. It then calls a validateAge()
function to check if the input is a valid age between 18 and 99. If the input is valid, it displays a success message and exits the loop. Otherwise, it prompts the user to enter a valid age again.
Real World Use Case: File Reading with Getline
Getline is also widely used for reading text from files in C++. It provides a convenient way to read entire lines of text, making file reading operations more manageable. Let's see an example of reading a text file using Getline:
#include <iostream> #include <fstream> using namespace std; int main() { string line; ifstream file("example.txt"); if (file.is_open()) { while (getline(file, line)) { cout << line << endl; } file.close(); } else { cout << "Failed to open file." << endl; } return 0; }
In this example, the program opens a file called "example.txt" using the ifstream
class. It then uses Getline in a loop to read each line of text from the file and display it to the console. Finally, it closes the file when done.
Performance Considerations Using Getline in C++
While Getline provides a convenient way to read lines of text, it's worth considering its performance implications when dealing with large files or high-speed input streams. Here are a few performance considerations when using Getline in C++:
1. Getline reads characters one by one until it encounters the specified delimiter or reaches the end of the input. This can be slow compared to reading a fixed number of characters at a time.
2. If you know the maximum length of the input line in advance, you can pre-allocate the string variable to avoid unnecessary reallocations and improve performance.
3. If performance is critical, consider using lower-level file reading functions, such as fread()
or fgets()
, instead of Getline.
It's important to note that the performance impact of using Getline will vary depending on the specific use case and the size and complexity of the input. If you're working with small files or the performance requirements are not demanding, Getline should suffice.
Advanced Technique: Manipulating Strings Post Getline
After reading a line of text using Getline, you can manipulate the resulting string using various string manipulation functions and techniques in C++. Let's explore a few advanced techniques:
1. Tokenizing the string: You can split the string into tokens using delimiters and process each token individually. This can be useful for parsing input that has a specific format.
2. Removing leading and trailing whitespace: You can use the trim()
function to remove leading and trailing whitespace from the string, ensuring clean input.
3. Converting the string to uppercase or lowercase: You can use the toupper()
or tolower()
functions to convert the string to uppercase or lowercase, respectively.
4. Replacing substrings: You can use the replace()
function to replace specific substrings within the string with other substrings.
Here's an example that demonstrates some of these techniques:
#include <iostream> #include <sstream> #include <algorithm> #include <cctype> using namespace std; void trim(string& str) { str.erase(str.begin(), find_if(str.begin(), str.end(), [](int ch) { return !isspace(ch); })); str.erase(find_if(str.rbegin(), str.rend(), [](int ch) { return !isspace(ch); }).base(), str.end()); } int main() { string line; cout << "Enter a sentence: "; getline(cin, line); // Tokenizing the string stringstream ss(line); string word; while (ss >> word) { cout << "Token: " << word << endl; } // Removing leading and trailing whitespace trim(line); cout << "Trimmed sentence: " << line << endl; // Converting the string to uppercase transform(line.begin(), line.end(), line.begin(), [](unsigned char c) { return toupper(c); }); cout << "Uppercase sentence: " << line << endl; // Replacing substrings string search = "SENTENCE"; string replace = "STRING"; size_t index = line.find(search); if (index != string::npos) { line.replace(index, search.length(), replace); } cout << "Replaced sentence: " << line << endl; return 0; }
In this example, the program reads a sentence from the user using Getline and then demonstrates various string manipulation techniques. It tokenizes the sentence, trims leading and trailing whitespace, converts the sentence to uppercase, and replaces a specific substring.
Related Article: The issue with Monorepos
Error Handling in C++ with Getline
When using Getline in C++, it's important to handle errors that may occur during input operations. Getline can fail for various reasons, such as reaching the end of the input stream or encountering an error condition. Here's an example that demonstrates error handling with Getline:
#include <iostream> using namespace std; int main() { string line; cout << "Enter a line of text: "; if (!getline(cin, line)) { if (cin.eof()) { cout << "End of input reached." << endl; } else { cout << "Failed to read input." << endl; } } else { cout << "You entered: " << line << endl; } return 0; }
In this example, the program checks if the Getline operation was successful by using the result of the function call in an if statement. If Getline fails, it checks if the end of the input stream was reached or if an error occurred. It then displays an appropriate error message based on the result.
Code Snippet Idea: Creating a Text-Based Quiz Using Getline
Getline can be used to create interactive text-based quizzes in C++. By reading user input using Getline, you can prompt the user with questions and validate their answers. Here's a simple code snippet that demonstrates this idea:
#include <iostream> using namespace std; bool validateAnswer(const string& input, const string& correctAnswer) { return input == correctAnswer; } int main() { string answer; cout << "Welcome to the Quiz!" << endl; cout << "Question 1: What is the capital of France?" << endl; cout << "Your answer: "; getline(cin, answer); if (validateAnswer(answer, "Paris")) { cout << "Correct answer!" << endl; } else { cout << "Wrong answer." << endl; } return 0; }
In this example, the program prompts the user with a question and reads their answer using Getline. It then calls a validateAnswer()
function to check if the answer matches the correct answer. Based on the validation result, it displays an appropriate message to the user.
Code Snippet Idea: Implementing a Simple Text Editor Using Getline
Getline can be used to implement a simple text editor in C++, allowing users to type and modify text. By continuously reading lines of text using Getline, you can provide basic text editing functionality. Here's a code snippet that demonstrates this idea:
#include <iostream> using namespace std; int main() { string document; cout << "Welcome to the Text Editor!" << endl; cout << "Enter your text. Press Enter twice to exit." << endl; string line; while (getline(cin, line) && !line.empty()) { document += line + "\n"; } cout << "Document content:" << endl; cout << document << endl; return 0; }
In this example, the program continuously reads lines of text using Getline until the user presses Enter without typing anything. It appends each line to a document
string, separating lines with newline characters. Finally, it displays the content of the document to the user.
Code Snippet Idea: Building a Command-Line Interface with Getline
Getline can be used to create a command-line interface (CLI) in C++, allowing users to interact with a program using text-based commands. By reading user input using Getline and processing it accordingly, you can build powerful CLI applications. Here's a code snippet that demonstrates this idea:
#include <iostream> using namespace std; void processCommand(const string& command) { if (command == "help") { cout << "Available commands: help, info, exit" << endl; } else if (command == "info") { cout << "This is a command-line interface program." << endl; } else if (command == "exit") { cout << "Exiting the program." << endl; } else { cout << "Invalid command. Type 'help' for available commands." << endl; } } int main() { string command; cout << "Welcome to the Command-Line Interface!" << endl; cout << "Type 'help' to see available commands." << endl; while (getline(cin, command)) { processCommand(command); if (command == "exit") { break; } } return 0; }
In this example, the program continuously reads user input using Getline and passes it to a processCommand()
function for processing. The function checks the command and executes the corresponding action or displays an error message for invalid commands. The program continues to accept commands until the user types "exit".
Related Article: Agile Shortfalls and What They Mean for Developers
Code Snippet Idea: Developing a File Converter Using Getline
Getline can be used to develop a file converter in C++, allowing users to convert the content of one file into another format. By reading lines of text from an input file using Getline and processing them accordingly, you can build a versatile file conversion tool. Here's a code snippet that demonstrates this idea:
#include <iostream> #include <fstream> using namespace std; void convertFile(const string& inputFile, const string& outputFile) { ifstream inFile(inputFile); ofstream outFile(outputFile); if (inFile.is_open() && outFile.is_open()) { string line; while (getline(inFile, line)) { // Perform conversion or processing on each line // Example: Convert each line to uppercase transform(line.begin(), line.end(), line.begin(), [](unsigned char c) { return toupper(c); }); outFile << line << endl; } inFile.close(); outFile.close(); cout << "File converted successfully." << endl; } else { cout << "Failed to open file." << endl; } } int main() { string inputFile, outputFile; cout << "Welcome to the File Converter!" << endl; cout << "Enter the input file name: "; getline(cin, inputFile); cout << "Enter the output file name: "; getline(cin, outputFile); convertFile(inputFile, outputFile); return 0; }
In this example, the program prompts the user to enter the names of the input and output files using Getline. It then calls a convertFile()
function, which opens the input and output files using ifstream
and ofstream
, respectively. It then reads each line from the input file using Getline, performs the desired conversion or processing, and writes the result to the output file. Finally, it displays a success message if the conversion was successful.
Code Snippet Idea: Making a Contact Information Parser with Getline
Getline can be used to parse contact information from a file or user input in C++. By reading lines of text using Getline and extracting relevant information using string manipulation techniques, you can build a contact information parser. Here's a code snippet that demonstrates this idea:
#include <iostream> #include <sstream> #include <vector> using namespace std; struct Contact { string name; string phone; string email; }; Contact parseContact(const string& line) { stringstream ss(line); string name, phone, email; ss >> name >> phone >> email; return {name, phone, email}; } int main() { string input; cout << "Enter contact information (name phone email): "; getline(cin, input); Contact contact = parseContact(input); cout << "Parsed contact information:" << endl; cout << "Name: " << contact.name << endl; cout << "Phone: " << contact.phone << endl; cout << "Email: " << contact.email << endl; return 0; }
In this example, the program prompts the user to enter contact information in the format "name phone email" using Getline. It then calls a parseContact()
function, which uses a stringstream to extract the name, phone, and email from the input line. The function returns a Contact
structure containing the parsed information, which is then displayed to the user.