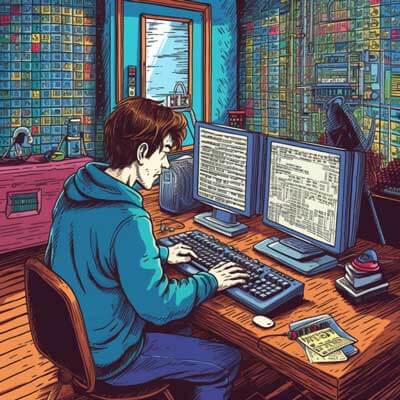
Table of Contents
The 'in' keyword in Python is used to check if a value is present in a sequence or collection. It is commonly used in if statements to perform conditional execution based on the presence or absence of a value. In this answer, we will explore how to use 'in' in a Python if statement with different types of sequences and collections.
Using 'in' with Lists
To check if a value is present in a list, you can use the 'in' keyword followed by the list name. Here's an example:
fruits = ['apple', 'banana', 'orange'] if 'apple' in fruits: print('Apple is present in the list')
In this example, the if statement checks if the value 'apple' is present in the list 'fruits'. If it is, the statement inside the if block is executed, which prints 'Apple is present in the list'.
You can also use the 'in' keyword with the 'not' keyword to check if a value is not present in a list. Here's an example:
fruits = ['apple', 'banana', 'orange'] if 'grape' not in fruits: print('Grape is not present in the list')
In this example, the if statement checks if the value 'grape' is not present in the list 'fruits'. If it is not, the statement inside the if block is executed, which prints 'Grape is not present in the list'.
Related Article: How to Use and Import Python Modules
Using 'in' with Strings
The 'in' keyword can also be used to check if a substring is present in a string. Here's an example:
message = 'Hello, world!' if 'world' in message: print('The word "world" is present in the message')
In this example, the if statement checks if the substring 'world' is present in the string 'message'. If it is, the statement inside the if block is executed, which prints 'The word "world" is present in the message'.
You can also use the 'in' keyword with the 'not' keyword to check if a substring is not present in a string. Here's an example:
message = 'Hello, world!' if 'python' not in message: print('The word "python" is not present in the message')
In this example, the if statement checks if the substring 'python' is not present in the string 'message'. If it is not, the statement inside the if block is executed, which prints 'The word "python" is not present in the message'.
Using 'in' with Tuples
The 'in' keyword can also be used to check if a value is present in a tuple. Here's an example:
numbers = (1, 2, 3, 4, 5) if 3 in numbers: print('The number 3 is present in the tuple')
In this example, the if statement checks if the value 3 is present in the tuple 'numbers'. If it is, the statement inside the if block is executed, which prints 'The number 3 is present in the tuple'.
Using 'in' with Sets
The 'in' keyword can also be used to check if a value is present in a set. Here's an example:
numbers = {1, 2, 3, 4, 5} if 3 in numbers: print('The number 3 is present in the set')
In this example, the if statement checks if the value 3 is present in the set 'numbers'. If it is, the statement inside the if block is executed, which prints 'The number 3 is present in the set'.
Related Article: How to Download a File Over HTTP in Python
Using 'in' with Dictionaries
The 'in' keyword can also be used to check if a key is present in a dictionary. Here's an example:
person = {'name': 'John', 'age': 30, 'city': 'New York'} if 'name' in person: print('The key "name" is present in the dictionary')
In this example, the if statement checks if the key 'name' is present in the dictionary 'person'. If it is, the statement inside the if block is executed, which prints 'The key "name" is present in the dictionary'.
You can also use the 'in' keyword with the 'not' keyword to check if a key is not present in a dictionary. Here's an example:
person = {'name': 'John', 'age': 30, 'city': 'New York'} if 'email' not in person: print('The key "email" is not present in the dictionary')
In this example, the if statement checks if the key 'email' is not present in the dictionary 'person'. If it is not, the statement inside the if block is executed, which prints 'The key "email" is not present in the dictionary'.