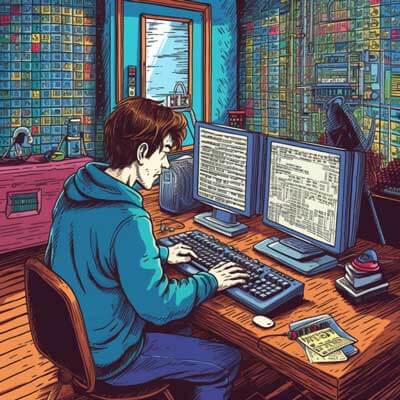
Table of Contents
Using JavaScript to Refresh or Reload a Page with jQuery
To refresh or reload a webpage using JavaScript and jQuery, you can utilize the location.reload()
method, which forces the current page to reload. In this answer, we will discuss how to achieve this using different approaches.
Related Article: How to Compare Two Dates in Javascript
Approach 1: Using the location.reload() Method
One way to refresh a page using JavaScript and jQuery is by calling the location.reload()
method. This method will reload the current page, including all resources such as stylesheets, JavaScript files, and images. Here's an example:
location.reload();
location.reload(true);
Approach 2: Using the location.href Property
Another approach to refresh a page using JavaScript and jQuery is by modifying the location.href
property. This property represents the URL of the current page and can be used to redirect the browser to a new URL. By assigning the current URL to location.href
, you can trigger a page refresh. Here's an example:
location.href = location.href;
This line of code assigns the current URL to location.href
, effectively reloading the page.
Best Practices and Considerations
When using JavaScript and jQuery to refresh a page, it's important to consider best practices and potential pitfalls. Here are some tips to keep in mind:
1. Avoid excessive page refreshes: Frequent page refreshes can hinder the user experience and impact performance. Only refresh the page when necessary, such as after submitting a form or when data needs to be updated.
2. Use appropriate event handlers: To trigger a page refresh at the right moment, bind the refresh code to the appropriate event handlers. For example, you might want to refresh the page after a button click or when a specific condition is met.
3. Consider alternative approaches: While JavaScript and jQuery can be used to refresh a page, it's worth exploring alternative approaches when applicable. For example, in some cases, you might be able to update specific elements on the page dynamically without requiring a full refresh.
4. Test across different browsers: Ensure that your page refresh code works correctly across different browsers and devices. Test your implementation thoroughly to avoid compatibility issues.
5. Communicate the refresh to the user: If a refresh is triggered as a result of a user action, consider providing a visual indicator or a message to inform the user about the refresh. This helps prevent confusion and provides a better user experience.