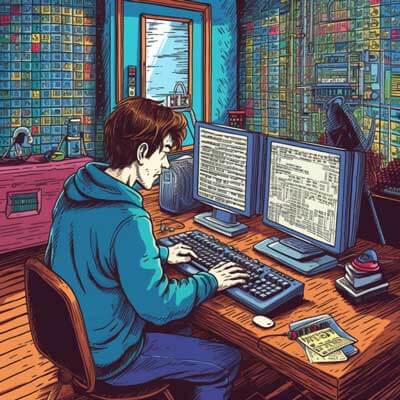
Table of Contents
In PHP, loops are used to execute a block of code repeatedly. They allow you to perform repetitive tasks without having to write the same code multiple times. There are several types of loops available in PHP, including the while loop, do-while loop, for loop, and foreach loop.
Syntax and Structure of Loops
The syntax and structure of loops in PHP are similar across different loop types. Here is the general structure of a loop:
The loop begins with an initialization step, where you set the initial value(s) of any variables used in the loop. The condition is then checked, and if it evaluates to true, the code inside the loop is executed. After each iteration, the loop update step is executed, which typically increments or updates the loop variable(s). The condition is checked again, and the loop continues until the condition evaluates to false.
Related Article: How to Use the Now Function in PHP
Using While Loops
The while loop is used when you want to execute a block of code repeatedly as long as a specified condition is true. The condition is checked before each iteration, and if it evaluates to true, the code inside the loop is executed.
Here's an example that uses a while loop to print numbers from 1 to 5:
<?php $number = 1; while ($number <= 5) { echo $number . "<br>"; $number++; } ?>
Output:
1 2 3 4 5
In this example, the loop starts with an initial value of $number = 1
. The condition $number <= 5
is checked, and as long as it evaluates to true, the code inside the loop is executed. The loop prints the value of $number
and then increments it by 1. This process continues until $number
becomes greater than 5.
Using Do-While Loops
The do-while loop is similar to the while loop, but the condition is checked after each iteration. This means that the code inside the loop will always be executed at least once, regardless of the condition.
Here's an example that uses a do-while loop to print numbers from 1 to 5:
<?php $number = 1; do { echo $number . "<br>"; $number++; } while ($number
Output:
1 2 3 4 5
In this example, the loop starts with an initial value of $number = 1
. The code inside the loop is executed, and then the condition $number <= 5
is checked. If the condition evaluates to true, the loop continues. The process repeats until $number
becomes greater than 5.
Using For Loops
The for loop is used when you know the number of iterations in advance. It consists of three parts: initialization, condition, and update.
Here's an example that uses a for loop to print numbers from 1 to 5:
<?php for ($number = 1; $number <= 5; $number++) { echo $number . "<br>"; } ?>
Output:
1 2 3 4 5
In this example, the loop starts with the initialization $number = 1
. The condition $number <= 5
is checked before each iteration, and if it evaluates to true, the code inside the loop is executed. After each iteration, the loop update $number++
increments the value of $number
by 1. The loop continues until $number
becomes greater than 5.
Related Article: How to Fix the 404 Not Found Error in Errordocument in PHP
The Foreach Loop
The foreach loop is used specifically for iterating over arrays or objects. It allows you to access each element in the array or each property in the object without the need for an explicit loop variable.
Here's an example that uses a foreach loop to iterate over an array of names:
<?php $names = ["John", "Jane", "Tom"]; foreach ($names as $name) { echo $name . "<br>"; } ?>
Output:
John Jane Tom
In this example, the loop iterates over each element in the $names
array. In each iteration, the value of the current element is assigned to the loop variable $name
, and the code inside the loop is executed.
Use Case: Iterating Through Arrays with Foreach
The foreach loop is commonly used to iterate through arrays and perform operations on each element. Here's an example that demonstrates how to use foreach to calculate the sum of an array of numbers:
Output:
The sum is: 15
In this example, the foreach loop iterates over each element in the $numbers
array. The value of each element is added to the $sum
variable using the +=
operator. After the loop finishes, the total sum is displayed.
Best Practice: Loop Optimization
When using loops, it's important to optimize your code for better performance. Here are a few best practices to consider:
1. Minimize unnecessary calculations inside the loop: Move any calculations that don't depend on the loop variable outside the loop to avoid redundant computations.
2. Use pre-increment or pre-decrement operators (++$x or --$x) instead of post-increment or post-decrement operators ($x++ or $x--) when possible. Pre-increment/decrement is slightly faster because it doesn't involve an additional assignment.
3. Avoid calling functions or methods inside the loop if their return value doesn't change during each iteration. Instead, calculate the value before the loop and store it in a variable.
4. Set the initial capacity of an array if you know the approximate number of elements it will contain. This can prevent unnecessary resizing operations during the loop.
Real World Example: Data Processing with Loops
Loops are often used in real-world scenarios for processing large amounts of data. Let's consider an example where we have an array of student scores, and we need to calculate the average score:
<?php $scores = [85, 92, 78, 90, 88]; $total = 0; $count = count($scores); for ($i = 0; $i
Output:
The average score is: 86.6
In this example, we use a for loop to iterate over the elements of the $scores
array. The value of each element is added to the $total
variable, and at the end of the loop, we calculate the average score by dividing the total by the number of scores.
Related Article: How to Delete An Element From An Array In PHP
Performance Consideration: Avoiding Infinite Loops
An infinite loop occurs when the loop condition always evaluates to true, resulting in an endless loop that continues indefinitely. Infinite loops can cause your program to hang or crash, so it's important to avoid them.
Here's an example of an infinite loop:
To prevent infinite loops, ensure that the loop condition eventually evaluates to false. You can use control structures like if
statements and loop control statements like break
or return
to exit the loop when a specific condition is met.
Advanced Technique: Nested Loops
Nested loops are loops within loops. They are useful when you need to perform iterations in multiple dimensions or iterate over multidimensional arrays.
Here's an example of a nested loop that prints a multiplication table:
<?php for ($i = 1; $i <= 10; $i++) { for ($j = 1; $j <= 10; $j++) { echo $i * $j . " "; } echo "<br>"; } ?>
Output:
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100
In this example, the outer loop iterates over the numbers from 1 to 10, representing the rows of the multiplication table. The inner loop iterates over the same range, representing the columns. The product of the row and column values is printed for each combination.
Code Snippet: Looping Through Multi-Dimensional Arrays
To loop through multi-dimensional arrays, you can use nested foreach loops. Here's an example that demonstrates how to loop through a 2D array:
<?php $matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; foreach ($matrix as $row) { foreach ($row as $value) { echo $value . " "; } echo "<br>"; } ?>
Output:
1 2 3 4 5 6 7 8 9
In this example, the outer foreach loop iterates over each row of the $matrix
array. The inner foreach loop iterates over the elements of each row. The value of each element is printed, followed by a space. After each row, a line break is added to separate the rows.
Code Snippet: Using Break and Continue in Loops
The break
and continue
statements are used to control the flow of loops.
The break
statement is used to exit a loop prematurely. It is commonly used to terminate a loop when a specific condition is met. Here's an example that uses the break
statement to exit a loop when a number is found:
Output:
1 2 3 4
In this example, the loop iterates over the elements of the $numbers
array. When the value of $number
is equal to 5, the break
statement is executed, and the loop is terminated.
The continue
statement is used to skip the remaining code inside a loop and move on to the next iteration. It is commonly used to skip certain values or conditions. Here's an example that uses the continue
statement to skip odd numbers:
Output:
2 4 6 8 10
In this example, the loop iterates over the elements of the $numbers
array. When the value of $number
is odd (not divisible by 2), the continue
statement is executed, and the remaining code inside the loop is skipped. The loop then moves on to the next iteration.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Code Snippet: Using Loops with Conditional Statements
Loops can be combined with conditional statements to perform more complex operations. Here's an example that uses a loop with an if statement to find and display even numbers:
Output:
2 4 6 8 10
In this example, the loop iterates over the elements of the $numbers
array. For each element, the if statement checks if the number is even (divisible by 2). If the condition is true, the number is displayed.
Code Snippet: Using Foreach with Key-Value Pairs
In addition to the value, the foreach loop can also provide access to the key (index) of each element in an array. This is useful when you need to perform operations based on both the key and value.
Here's an example that demonstrates how to use foreach with key-value pairs:
"red", "banana" => "yellow", "orange" => "orange" ]; foreach ($fruits as $fruit => $color) { echo "The color of the $fruit is $color.<br>"; } ?>
Output:
The color of the apple is red. The color of the banana is yellow. The color of the orange is orange.
In this example, the loop iterates over the $fruits
array. The key of each element is assigned to the variable $fruit
, and the value is assigned to the variable $color
. The code inside the loop uses these variables to display the fruit name and its corresponding color.
Code Snippet: Foreach with List to Destructure Arrays
The list
language construct can be used with foreach to destructure arrays into individual variables. This allows you to easily access and work with the values of an array.
Here's an example that demonstrates how to use foreach with list:
<?php $person = ["John", "Doe", 30]; list($firstName, $lastName, $age) = $person; echo "First Name: $firstName<br>"; echo "Last Name: $lastName<br>"; echo "Age: $age<br>"; ?>
Output:
First Name: John Last Name: Doe Age: 30
In this example, the list
construct is used to assign the values of the $person
array to the variables $firstName
, $lastName
, and $age
. The code then displays the values of these variables.
Error Handling: Dealing with Loop Errors
When working with loops, it's important to handle any potential errors that may occur. Here are a few common errors that can happen in loops and how to deal with them:
1. Infinite loops: As mentioned earlier, infinite loops can cause your program to hang or crash. To prevent infinite loops, ensure that the loop condition eventually evaluates to false. Use control structures like if
statements and loop control statements like break
or return
to exit the loop when necessary.
2. Off-by-one errors: Off-by-one errors occur when you incorrectly set the loop condition or the loop update step. This can lead to unexpected results or even infinite loops. Carefully review your loop conditions and ensure that they are correct.
3. Null or undefined values: When working with arrays or objects in loops, it's important to check for null or undefined values before accessing them. This can help prevent errors like "Undefined index" or "Undefined property" from occurring. Use control structures like isset()
or empty()
to validate the existence of values before using them.
Related Article: How to Use Laravel Folio: Tutorial with Advanced Examples
Performance Consideration: Reducing Loop Overhead
Loop overhead refers to the additional time and resources consumed by a loop structure itself, rather than the code inside the loop. Minimizing loop overhead can lead to improved performance. Here are a few tips to reduce loop overhead:
1. Minimize the number of iterations: If possible, try to reduce the number of iterations by optimizing your code or using more efficient algorithms. Consider using built-in functions or libraries that can perform operations on entire arrays or collections instead of individual elements.
2. Use efficient loop constructs: Choose the appropriate loop construct for your specific needs. For example, use a foreach loop when iterating over arrays, as it is optimized for this purpose. Avoid using a foreach loop when you need to modify the array itself, as it creates a copy of the array.
3. Move expensive operations outside the loop: If you have expensive calculations or function calls that don't depend on the loop variable, move them outside the loop to avoid redundant computations.
4. Cache loop values: If you need to access the same value multiple times within a loop, consider storing it in a variable outside the loop to avoid repeated lookups.
Advanced Technique: Using Recursive Loops
Recursive loops, also known as nested loops with recursion, are a powerful technique that allows a loop to call itself within its own body. This can be useful in scenarios where you need to perform repetitive actions on nested or hierarchical data structures.
Here's an example that demonstrates how to use a recursive loop to calculate the factorial of a number:
Output:
Factorial of 5: 120
In this example, the factorial()
function uses recursion to calculate the factorial of a number. The base case is when $n
reaches 0, in which case the function returns 1. Otherwise, the function calls itself with the argument $n - 1
and multiplies the result by $n
. This process continues until the base case is reached.
Recursive loops can be a powerful tool, but they require careful design to prevent infinite recursion and manage memory usage.
Use Case: Iterating Over Object Properties with Foreach
The foreach loop can also be used to iterate over the properties of an object. This can be useful when you need to perform operations on each property dynamically.
Here's an example that demonstrates how to use foreach to iterate over an object's properties:
$value) { echo "$property: $value<br>"; } ?>
Output:
name: John age: 30 city: New York
In this example, the foreach loop iterates over the properties of the $person
object. The name of each property is assigned to the variable $property
, and the value of each property is assigned to the variable $value
. The code inside the loop displays the name and value of each property.
Real World Example: Building a Pagination System with Loops
Loops are often used in the development of pagination systems, which allow users to navigate through a large dataset or list of items. Let's consider an example where we have an array of items and we need to display them in pages of a certain size:
<?php $items = ["Item 1", "Item 2", "Item 3", "Item 4", "Item 5", "Item 6", "Item 7", "Item 8", "Item 9", "Item 10"]; $itemsPerPage = 3; $currentPage = 2; $startIndex = ($currentPage - 1) * $itemsPerPage; $endIndex = $startIndex + $itemsPerPage; for ($i = $startIndex; $i < $endIndex; $i++) { if (isset($items[$i])) { echo $items[$i] . "<br>"; } } ?>
Output:
Item 4 Item 5 Item 6
In this example, we have an array of items and we want to display them in pages of 3 items each. We specify the current page and calculate the start and end indices for that page. The loop iterates over the specified range of indices and displays the corresponding items. The isset()
function is used to check if an item exists at a given index before displaying it.
Related Article: How To Fix the “Array to string conversion” Error in PHP
Best Practice: Avoiding Off-By-One Errors in Loops
Off-by-one errors are common mistakes that occur when the loop condition or the loop update step is not set correctly, leading to unexpected behavior or infinite loops. Here are a few best practices to avoid off-by-one errors:
1. Start the loop variable from the correct initial value: Depending on the problem or data structure, the loop variable should start from the appropriate initial value. For example, when iterating over an array, the loop variable should start from 0 if the array uses zero-based indexing.
2. Set the loop condition correctly: Ensure that the loop condition checks for the correct termination condition. If the condition is not set correctly, the loop may not execute the expected number of times.
3. Update the loop variable correctly: The loop update step should increment or update the loop variable in the correct way to ensure that the loop progresses as expected. If the update step is not set correctly, the loop may not terminate or may skip certain values.