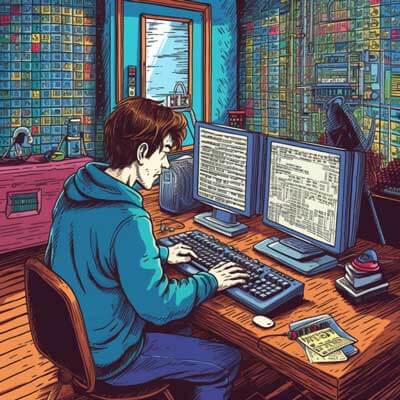
Table of Contents
The map
function in JavaScript is primarily used to iterate over an array and transform its elements by applying a provided function to each element. However, when it comes to objects, JavaScript does not have a built-in map
function. In this case, you can achieve similar functionality by using a combination of other array methods and object manipulation techniques. Here are two possible approaches to using a map-like function on objects in JavaScript:
Approach 1: Converting the Object to an Array and Applying Map
One way to use a map-like function on an object is by converting the object to an array of key-value pairs and then applying the map
function. Here's how you can do it:
const obj = { key1: 'value1', key2: 'value2', key3: 'value3' }; const result = Object.entries(obj).map(([key, value]) => { // Apply your transformation logic here return { key, transformedValue: value.toUpperCase() }; }); console.log(result);
In this approach, we use Object.entries(obj)
to convert the object into an array of key-value pairs. Then, we can apply the map
function on this array, where each element is an array [key, value]
. Inside the map callback function, you can apply your desired transformation logic to each key-value pair. Finally, the transformed key-value pairs are collected in the result
array.
Related Article: How To Convert A String To An Integer In Javascript
Approach 2: Iterating Over Object Properties and Creating a New Object
Another approach is to iterate over the properties of the object using a for...in
loop and create a new object containing the transformed values. Here's an example:
const obj = { key1: 'value1', key2: 'value2', key3: 'value3' }; const result = {}; for (const key in obj) { if (obj.hasOwnProperty(key)) { // Apply your transformation logic here result[key] = obj[key].toUpperCase(); } } console.log(result);
In this approach, we use a for...in
loop to iterate over the properties of the object. We check if the property belongs to the object itself (not inherited from its prototype) using hasOwnProperty
. Inside the loop, you can apply your desired transformation logic to each property and assign the transformed value to the corresponding property in the result
object.
Best Practices and Considerations
Related Article: How to Check If a String is a Valid Number in JavaScript
- It's important to note that objects in JavaScript do not have a guaranteed order for their properties. Therefore, the order of the transformed key-value pairs in the resulting array or object may not match the original order of the object properties.
- When using the map
function or iterating over object properties, be mindful of the potential performance implications, especially if you are working with large objects. Consider whether other object manipulation techniques, such as reduce
, might be more suitable for your specific use case.
- If you are working with nested objects, you can adapt the approaches mentioned above to handle the nested properties. You may need to use recursion or additional looping to traverse and transform the nested structures.
- For more advanced object manipulation and transformation tasks, you might consider using libraries like Lodash or Ramda, which provide a rich set of utility functions for working with objects and arrays in JavaScript.