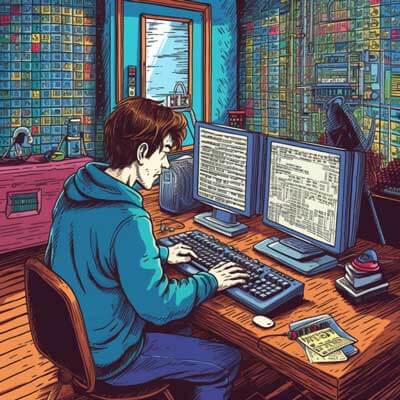
Table of Contents
Overview of Matplotlib with Chinese Text
Matplotlib is a popular plotting library in Python that allows users to create a wide array of static, animated, and interactive visualizations. For users working with Chinese text, Matplotlib can handle this requirement, but it requires additional configuration to display the characters correctly. Understanding how to set up and use Chinese fonts in Matplotlib is crucial for creating plots that are both visually appealing and informative.
The challenge lies in the fact that Matplotlib does not include Chinese fonts by default. Therefore, when creating plots with Chinese characters, it is necessary to install the required fonts and configure Matplotlib accordingly. This ensures that Chinese text appears correctly on plots, allowing for clear communication of data.
Related Article: How to Use Numpy Percentile in Python
Setting Up Chinese Fonts in Matplotlib
Installing Chinese fonts on your system is the first step towards using them in Matplotlib. Commonly used Chinese fonts include SimHei, Microsoft YaHei, and Noto Sans CJK. Depending on your operating system, the installation process may vary.
For Windows users, you can download a font file (TTF) and install it by double-clicking the file and selecting "Install." On macOS, you can use the Font Book application to add new fonts. Linux users can typically install fonts via their package manager. For example, on Ubuntu, the following command installs the Noto Sans CJK font package:
sudo apt install fonts-noto-cjk
Once the fonts are installed, ensure they are recognized by Matplotlib. You can check the available fonts in your Matplotlib environment using the following code:
import matplotlib.font_manager as fm for font in fm.findSystemFonts(fontpaths=None, fontext='ttf'): print(font)
This will list all the TrueType fonts available to Matplotlib, including the newly installed Chinese fonts.
Configuring Matplotlib for Chinese Support in Python
After installing the necessary fonts, configuration is required to ensure Matplotlib can find and utilize them. This involves setting the default font properties. One way to do this is by modifying the matplotlibrc
configuration file. This file contains various settings for Matplotlib, allowing for customization of font family, font size, line width, and more.
Locate the matplotlibrc
file. If you do not have one, you can create it in your working directory. Add the following lines to set the default font properties:
font.family : sans-serif font.sans-serif : SimHei # or any other installed Chinese font
An alternative approach is to set the font properties directly in your script using the following code:
import matplotlib.pyplot as plt plt.rcParams['font.sans-serif'] = ['SimHei'] # Specify your Chinese font plt.rcParams['axes.unicode_minus'] = False # To display negative signs correctly
This ensures that every plot generated in the script will use the specified Chinese font.
Creating Chinese Labels for Plots
Creating labels in Chinese for your plots is a straightforward process once the fonts are configured. Labels can be added to various elements of the plot, such as the x-axis, y-axis, and titles. Here’s an example demonstrating how to create a simple plot with Chinese labels:
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6]) plt.title('简单的折线图') # Title in Chinese plt.xlabel('横轴标签') # X-axis label in Chinese plt.ylabel('纵轴标签') # Y-axis label in Chinese plt.show()
This code generates a simple line plot with Chinese text for the title and labels. The characters should appear correctly on the plot, provided that the configuration has been set up accurately.
Related Article: How to Convert String to Bytes in Python 3
Customizing Chinese Titles in Plots
Customizing titles in plots enhances the visual appeal and provides clarity about the data being presented. In addition to using Chinese text, you can adjust font size, weight, and color for better emphasis. The following example illustrates how to customize the title of a plot:
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6]) plt.title('自定义标题', fontsize=16, fontweight='bold', color='blue') # Customized title plt.show()
In this example, the title "自定义标题" appears in blue, bold, and at a font size of 16. Adjusting these parameters allows more control over the presentation, making the plot more engaging for the audience.
Adjusting Chinese Legends in Matplotlib
Legends play a vital role in comprehending what different lines or markers represent in a plot. Including Chinese text in legends follows a similar process to titles and labels. Here’s how you can add a legend with Chinese text:
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6], label='数据一') # Legend label in Chinese plt.plot([1, 2, 3], [6, 5, 4], label='数据二') # Another legend label in Chinese plt.legend() # Display the legend plt.show()
The legend will display the labels "数据一" and "数据二" in Chinese, helping viewers understand the different data series being represented. This feature becomes crucial when presenting complex datasets with multiple lines or markers.
Setting Chinese Axis Labels
Axis labels provide context to the data being visualized. Setting Chinese labels for axes can help communicate the significance of the data more effectively. Here is an example of how to set Chinese labels for both axes:
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('时间 (秒)') # X-axis label in Chinese plt.ylabel('值') # Y-axis label in Chinese plt.show()
This plot features axis labels that clearly indicate the context of the data. The x-axis is labeled "时间 (秒)" meaning "Time (seconds)," while the y-axis is labeled simply "值" meaning "Value." These labels make the plot more informative for Chinese-speaking audiences.
Saving Plots with Chinese Characters
When saving plots that contain Chinese characters, it's crucial to ensure that the saved file retains the proper encoding. Matplotlib supports saving plots in various formats such as PNG, PDF, and SVG. Use the following code to save a plot with Chinese characters:
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6]) plt.title('保存带有中文字符的图') plt.savefig('plot_with_chinese.png', dpi=300, bbox_inches='tight') # Saving the plot
Related Article: PHP vs Python: How to Choose the Right Language
Handling Chinese Characters in Graphs
Handling Chinese characters in graphs is about ensuring that all text elements are rendered correctly and are legible. This includes titles, labels, and legends. If the fonts are not set properly, the characters may not display or may appear as boxes or question marks.
To troubleshoot issues with Chinese characters, check the following points:
1. Ensure that the correct font is installed and configured in Matplotlib.
2. Verify that the font supports the specific Chinese characters being used.
3. Test the plot with a simple example to confirm that the setup works before implementing it in more complex visualizations.
import matplotlib.pyplot as plt plt.figure(figsize=(8, 5)) plt.plot([1, 2, 3], [4, 5, 6]) plt.title('测试中文字符') # Test title in Chinese plt.show()
This code snippet can be used for quick verification to ensure that the configuration is correct and that Chinese characters display as intended.
Using Subplots with Chinese Text
Creating subplots allows for the comparison of multiple datasets within a single figure. Matplotlib makes it easy to add Chinese text to subplots. The following example demonstrates how to set up subplots with Chinese titles and labels:
import matplotlib.pyplot as plt fig, axs = plt.subplots(2, 1, figsize=(8, 10)) axs[0].plot([1, 2, 3], [4, 5, 6]) axs[0].set_title('第一幅图') # Title in Chinese for the first subplot axs[0].set_xlabel('横轴') # X-axis label in Chinese axs[0].set_ylabel('纵轴') # Y-axis label in Chinese axs[1].plot([1, 2, 3], [6, 5, 4]) axs[1].set_title('第二幅图') # Title in Chinese for the second subplot axs[1].set_xlabel('横轴') # X-axis label in Chinese axs[1].set_ylabel('纵轴') # Y-axis label in Chinese plt.tight_layout() # Adjust the layout plt.show()
Applying Color Maps to Chinese Data
Color maps enhance the visual appeal of plots and can provide additional insights into the data being presented. When working with Chinese data, applying color maps in Matplotlib remains the same as with English data. Here’s an example of how to create a heatmap with Chinese labels:
import matplotlib.pyplot as plt import numpy as np data = np.random.rand(10, 10) # Generate random data plt.imshow(data, cmap='hot', interpolation='nearest') plt.title('热图示例') # Title in Chinese plt.colorbar(label='值') # Colorbar label in Chinese plt.xticks(ticks=np.arange(10), labels=[f'列{i}' for i in range(10)]) # X-ticks in Chinese plt.yticks(ticks=np.arange(10), labels=[f'行{i}' for i in range(10)]) # Y-ticks in Chinese plt.show()