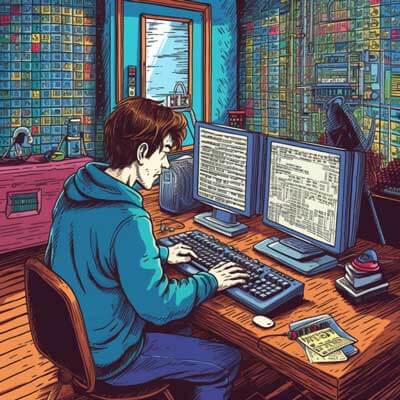
Table of Contents
To create a directory in Linux using the mkdir
command only if the directory doesn't already exist, you can use the -p
option along with a conditional statement. Here are two possible ways to achieve this:
Method 1: Using Conditional Statement
You can use a conditional statement to check if the directory exists before running the mkdir
command. Here's an example:
if [ ! -d "/path/to/directory" ]; then mkdir "/path/to/directory" fi
In this example, the command if [ ! -d "/path/to/directory" ]
checks if the directory /path/to/directory
does not exist. If it doesn't exist, the mkdir "/path/to/directory"
command is executed to create the directory.
You can replace /path/to/directory
with the actual path of the directory you want to create.
Related Article: How to Handle Quotes with Md5sum in Bash Scripts
Method 2: Using the -p Option
The mkdir
command in Linux has a -p
option that allows you to create parent directories as needed. When using this option, the command will create the directory only if it doesn't already exist. Here's an example:
mkdir -p "/path/to/directory"
In this example, the command mkdir -p "/path/to/directory"
creates the directory /path/to/directory
if it doesn't already exist. If the directory already exists, the command will do nothing.
You can replace /path/to/directory
with the actual path of the directory you want to create.
Best Practices and Suggestions
Related Article: How to Loop Through an Array of Strings in Bash
- When using the conditional statement approach, make sure to enclose the path in quotes if it contains spaces or special characters. For example, if [ ! -d "/path/with spaces" ]
.
- It's a good practice to use absolute paths when creating directories to avoid any ambiguity.
- If you need to create multiple directories at once, you can provide multiple paths to the mkdir
command, separated by spaces. For example, mkdir -p "/path/to/dir1" "/path/to/dir2"
.
- If you are writing a script that requires creating directories, consider adding error handling to gracefully handle any failures that may occur during the directory creation process.
- If you want to create directories with specific permissions, you can use the -m
option followed by the desired permissions. For example, mkdir -p -m 755 "/path/to/directory"
.
- If you want to create directories with different ownership, you can use the -m
option followed by the desired ownership. For example, mkdir -p -m 755 -o username "/path/to/directory"
.