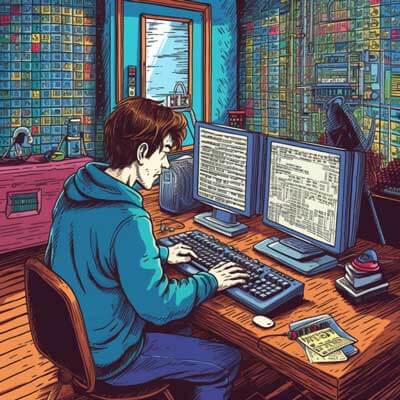
Table of Contents
To utilize the MySQL query string contains functionality, you can use the LIKE operator in combination with the % wildcard character. This allows you to search for a specific string within a column of a table. Here are two possible approaches to using the MySQL query string contains:
Approach 1: Using the LIKE Operator
To perform a basic string contains search using the LIKE operator, you can construct a query like this:
SELECT * FROM table_name WHERE column_name LIKE '%search_string%';
In this query, replace table_name
with the name of your table and column_name
with the name of the column you want to search in. Replace search_string
with the specific string you are looking for. The % wildcard character is used before and after the search string to indicate that the string can appear anywhere within the column value.
For example, if you have a table called employees
with a column named name
, and you want to search for all employees whose name contains the string "John", you can use the following query:
SELECT * FROM employees WHERE name LIKE '%John%';
This query will return all rows where the name
column contains the string "John".
Related Article: Tutorial: Full Outer Join versus Join in SQL
Approach 2: Using REGEXP
If you need more advanced pattern matching capabilities, you can use the REGEXP operator. This allows you to use regular expressions to define the search pattern. Here's an example:
SELECT * FROM table_name WHERE column_name REGEXP 'regex_pattern';
Replace table_name
with the name of your table and column_name
with the name of the column you want to search in. Replace regex_pattern
with the regular expression pattern you want to match against.
For instance, if you want to search for all rows where the name
column starts with "J" and is followed by any two characters, you can use the following query:
SELECT * FROM employees WHERE name REGEXP '^J..';
This query will return all rows where the name
column starts with "J" and is followed by any two characters.
Best Practices
When using the MySQL query string contains functionality, keep the following best practices in mind:
1. Use appropriate indexes: To optimize the performance of your queries, consider adding indexes to the columns you frequently search for string contains. This can significantly speed up the search process.
2. Be cautious with leading wildcards: Using leading wildcards (% wildcard at the beginning of the search string) can make the query slower since it cannot leverage indexes effectively. Try to avoid this if possible.
3. Consider case sensitivity: By default, the LIKE operator is case-insensitive in MySQL. However, if you need case-sensitive searching, you can use the BINARY operator before the column name, like column_name BINARY LIKE '%search_string%'
.
Alternative Ideas
While the LIKE operator and REGEXP are commonly used for string contains searches in MySQL, there are alternative ideas to consider:
1. Full-text search: If you require more advanced search capabilities, including relevance ranking and word stemming, you can explore MySQL's full-text search feature. It provides a useful way to search for text within large datasets.
2. External search engines: For even more advanced search functionality, you might consider using external search engines such as Elasticsearch or Solr. These engines are specifically designed for efficient and accurate search operations.