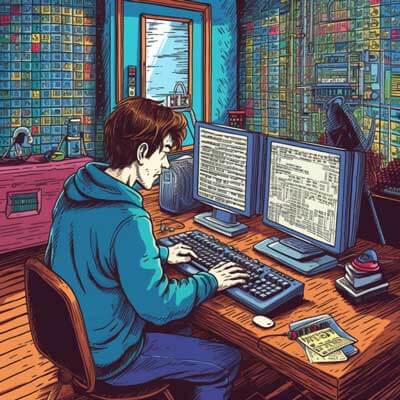
Table of Contents
Named tuples in Python provide a convenient way to define and work with lightweight, immutable data structures. They are similar to regular tuples, but with the added benefit of being able to access elements by name instead of just by index. This makes named tuples more readable and self-explanatory, especially when dealing with complex data.
Creating a Named Tuple
To use named tuples in Python, you first need to import the namedtuple
function from the collections module:
from collections import namedtuple
Then, you can define a named tuple by calling the namedtuple
function and passing in the name of the tuple and a sequence of field names as arguments. Here's an example:
Person = namedtuple('Person', ['name', 'age', 'location'])
In this example, we defined a named tuple called Person
with three fields: name
, age
, and location
. Each field is represented by a string.
Related Article: How to Save and Load Objects Using pickle.load in Python
Creating Instances of a Named Tuple
Once you have defined a named tuple, you can create instances of it by calling the named tuple as if it were a function. You pass in the values for each field as arguments. Here's an example:
person1 = Person('Alice', 25, 'New York') person2 = Person('Bob', 30, 'London')
In this example, we created two instances of the Person
named tuple: person1
and person2
. Each instance has values assigned to the name
, age
, and location
fields.
Accessing Fields in a Named Tuple
You can access the fields in a named tuple using dot notation. Each field acts as an attribute of the named tuple instance. Here's an example:
print(person1.name) print(person2.age)
In this example, we accessed the name
field of person1
and the age
field of person2
.
Immutable Nature of Named Tuples
Named tuples are immutable, which means that their values cannot be changed after they are created. If you try to assign a new value to a field, you will get an error. Here's an example:
person1.age = 30 # This will raise an error
To modify a field in a named tuple, you need to create a new named tuple instance with the updated value.
Related Article: Python Deleter Tutorial
Named Tuple Methods
Named tuples come with several useful methods that you can use to manipulate and work with the data. Some of the commonly used methods include:
- _asdict()
: Returns the named tuple as an ordered dictionary.
- _replace()
: Creates a new named tuple with some fields replaced by new values.
- _fields
: Returns a tuple of field names.
- _make(iterable)
: Creates a new named tuple from an iterable.
Here's an example that demonstrates the usage of these methods:
print(person1._asdict()) person3 = person1._replace(age=30) print(person3) print(person1._fields) person4 = Person._make(['Charlie', 35, 'Paris']) print(person4)
In this example, we used the _asdict()
method to convert the named tuple to an ordered dictionary, the _replace()
method to create a new named tuple with the age field replaced, the _fields
attribute to get the field names, and the _make()
method to create a new named tuple from an iterable.
Benefits of Using Named Tuples
Named tuples offer several benefits over regular tuples and other data structures:
- Readability: Named tuples provide self-descriptive field names that make the code more readable and understandable.
- Immutable: Named tuples are immutable, which means that their values cannot be accidentally modified. This can help prevent bugs and make the code more robust.
- Memory Efficiency: Named tuples are more memory-efficient compared to regular dictionaries or objects because they don't store field names with each instance.
- Compatibility: Named tuples can be used in places where regular tuples are expected, making them compatible with existing code and libraries.
- Serialization: Named tuples can be easily serialized and deserialized using standard serialization libraries in Python, such as pickle.