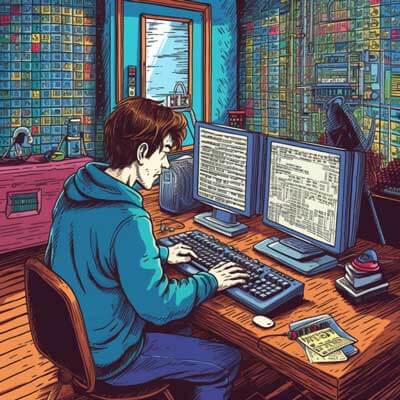
Table of Contents
The ngIf directive in AngularJS allows you to conditionally show or hide elements in your HTML based on a specific expression. However, unlike some other programming languages, AngularJS does not provide a built-in ngIf-else directive. In this answer, we'll explore two possible approaches to achieve the ngIf-else functionality in AngularJS.
Approach 1: Using ngIf with a Wrapper Element
One way to achieve the ngIf-else functionality is by using the ngIf directive in combination with a wrapper element. Here's how you can do it:
<div> <!-- Content to be displayed if the condition is true --> </div> <div> <!-- Content to be displayed if the condition is false --> </div>
In this approach, we use two separate div elements, each with its own ngIf directive. The first div will be displayed if the condition is true, and the second div will be displayed if the condition is false.
For example, let's say we have a variable named "showMessage" in our controller, which determines whether a message should be displayed or not. We can use this variable in the ngIf directive to show or hide the message:
<div> <p>Hello, world!</p> </div> <div> <p>Goodbye, world!</p> </div>
In the above example, if the "showMessage" variable is true, the message "Hello, world!" will be displayed. Otherwise, if the "showMessage" variable is false, the message "Goodbye, world!" will be displayed.
Related Article: How to Check for Null Values in Javascript
Approach 2: Using ngSwitch Directive
Another approach to achieve the ngIf-else functionality in AngularJS is by using the ngSwitch directive. The ngSwitch directive allows you to conditionally switch between multiple elements based on a specific expression. Here's how you can do it:
<div> <p>Content to be displayed if the condition is true</p> <p>Content to be displayed if the condition is false</p> </div>
In this approach, we use the ngSwitch directive on a wrapper element, and then use the ngSwitchWhen directive on the child elements to define the different cases.
For example, let's say we have a variable named "isLoggedIn" in our controller, which determines whether a user is logged in or not. We can use this variable in the ngSwitch directive to switch between different messages based on the user's login status:
<div> <p>Welcome, user!</p> <p>Please log in to continue.</p> </div>
In the above example, if the "isLoggedIn" variable is true, the message "Welcome, user!" will be displayed. Otherwise, if the "isLoggedIn" variable is false, the message "Please log in to continue." will be displayed.
Best Practices
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
When using ngIf or ngSwitch directives with ngIf-else functionality in AngularJS, it's important to keep the following best practices in mind:
1. Use meaningful variable names: Choose variable names that accurately describe the condition you are checking. This improves code readability and makes it easier for other developers to understand your code.
2. Avoid complex expressions: Keep the expressions used in ngIf or ngSwitch directives simple and easy to understand. If the expression becomes too complex, consider moving the logic to a controller function instead.
3. Minimize DOM manipulation: Be mindful of the number of elements and their complexity inside ngIf or ngSwitch directives. Excessive DOM manipulation can have performance implications, especially if the condition changes frequently.
4. Consider using ng-show and ng-hide: If you only need to show or hide elements based on a condition without removing them from the DOM, you can use the ng-show and ng-hide directives instead of ngIf. This can be more efficient in some scenarios.