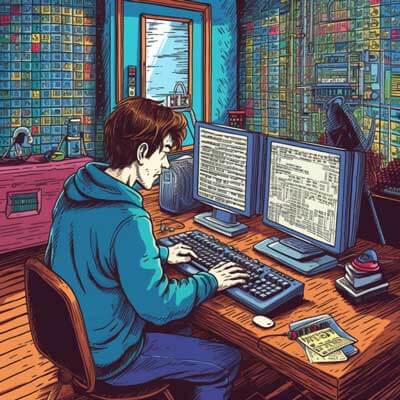
Table of Contents
Overview of npm
npm, which stands for Node Package Manager, is an essential tool for managing JavaScript packages. It serves as the default package manager for the JavaScript runtime environment Node.js. With npm, developers can easily install, share, and manage libraries and tools required for their applications. The npm registry hosts thousands of open-source packages, making it a vital resource for developers looking to enhance their projects.
This tool allows for the automation of the process of installing and updating packages. It also provides a way to manage dependencies, ensuring that an application can maintain the necessary libraries it requires to function correctly. In addition, npm simplifies the process of sharing code, as developers can publish their own packages for others to use.
Related Article: How to Fix Yarn v4 npm Publish 401 Error
Installing npm
To begin using npm, it is necessary to have Node.js installed, as npm comes bundled with it. The installation process varies depending on the operating system being used.
For Windows and MacOS, visiting the official Node.js website is the best approach:
1. Navigate to the Node.js download page: https://nodejs.org/.
2. Download the installer for your operating system.
3. Run the installer and follow the on-screen instructions.
For Linux, the installation can be done via package managers. For example, using apt for Ubuntu:
sudo apt update sudo apt install nodejs npm
After installation, verify that npm is installed correctly by checking the version:
npm -v
This command will return the version number of npm, confirming the installation was successful.
Creating package.json
The package.json file is the heart of any npm project. It holds metadata about the project and lists all the dependencies required. To create a package.json file, navigate to your project directory and run:
npm init
This command will prompt you to fill out information such as the project name, version, description, entry point, and author. If you wish to skip the prompts and create a default package.json file, you can run:
npm init -y
The generated package.json file will look something like this:
{ "name": "your-project-name", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "", "license": "ISC" }
This file serves as a blueprint for your project, detailing its structure and dependencies.
Setting Up a New Package
When starting a new project, it is essential to set up a dedicated directory. Create a new folder for your project and navigate into it:
mkdir my-new-project cd my-new-project
Next, initialize npm to create a package.json file:
npm init -y
With the package.json created, you can now begin adding packages that your project will use. The setup stage is crucial as it lays the foundation for the development work ahead.
Related Article: How to use npm install -i for package installation
Installing Packages with npm
To add packages to your project, npm provides a simple command. For example, to install the popular library 'express', run:
npm install express
This command will download the express package and add it to the dependencies in your package.json file. The package will also be placed in the node_modules directory, which npm creates to store all installed packages.
After installation, the dependencies section in your package.json might look like this:
"dependencies": { "express": "^4.17.1" }
The caret (^) symbol indicates that any minor version updates will be automatically installed.
Managing Dependencies in npm
Managing dependencies is a crucial part of using npm. It ensures that the correct versions of packages are used throughout the development process. Each dependency in the package.json file specifies a version range that npm will respect during installation.
In situations where multiple packages depend on the same library, npm uses a nested structure to avoid conflicts. This means that if two packages require different versions of the same dependency, npm will install both versions in separate directories under node_modules.
To check for outdated packages, use:
npm outdated
This command lists all packages that have newer versions available. It is essential to keep dependencies up to date to benefit from fixes and new features.
Updating Packages
To update packages in your project, the npm update command can be used. This command updates all the packages to their latest versions according to the version ranges specified in package.json:
npm update
If you want to update a specific package, provide its name:
npm update express
Additionally, if you want to install the latest version of a package regardless of the version range specified, you can use the following command:
npm install express@latest
This command fetches the most recent version of express from the npm registry and updates package.json accordingly.
Uninstalling Packages
If a package is no longer needed in your project, it can be uninstalled easily. To remove a package, use the npm uninstall command followed by the package name:
npm uninstall express
This command will remove the express package from the node_modules directory and also update the package.json file to reflect the change by removing express from the dependencies.
It is also possible to uninstall packages globally if they were installed that way. Use the -g flag for global uninstallation:
npm uninstall -g package-name
This will remove the specified package from the global scope.
Related Article: How To Set Npm Registry Configuration
Working with npm Scripts
npm allows for the automation of tasks through scripts defined in the package.json file. Scripts can include commands to run tests, start a server, or build your code.
In the scripts section of your package.json, you can define custom commands. For example:
"scripts": { "start": "node index.js", "test": "jest" }
To run a script, use the npm run command followed by the script name:
npm run start
This command will execute the start script, which in this case runs the index.js file using Node.js. Similarly, for testing, you can use:
npm run test
This approach helps streamline development workflows by allowing developers to define and execute common tasks with ease.
Package Version Management
Managing package versions is vital for maintaining stability in a project. The versioning system in npm follows semantic versioning (semver), which is structured as MAJOR.MINOR.PATCH.
- MAJOR version changes introduce breaking changes.
- MINOR version changes add functionality in a backward-compatible manner.
- PATCH version changes include backward-compatible bug fixes.
In the package.json file, you can specify version ranges to control which versions of a package can be installed. Common symbols include:
- ^
allows updates that do not change the leftmost non-zero digit.
- ~
allows updates for the last digit, meaning only patch-level changes.
- >
indicates that any version greater than the specified version can be installed.
This version management strategy helps ensure that projects remain stable while still allowing for updates and improvements to dependencies.