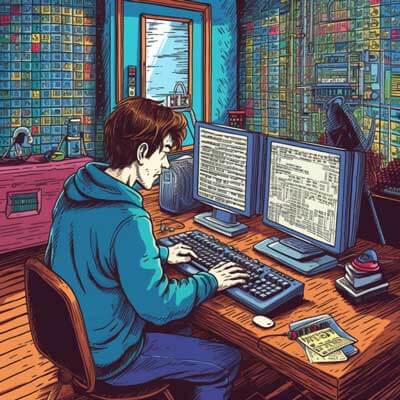
Table of Contents
Overview of npm run dev
npm run dev is a command used in Node.js projects to initiate development mode. It typically sets up a local environment that allows developers to test and run their applications while making changes in real-time. This command is defined in the scripts section of a project's package.json file, which is a central place to manage project dependencies and scripts.
When running npm run dev, developers can expect features such as live reloading and hot module replacement. These features can significantly streamline the development process by allowing changes to be reflected instantly in the browser without needing to refresh the page manually.
Related Article: How to Fix Yarn v4 npm Publish 401 Error
What npm run dev Does
The npm run dev command executes a script defined in the package.json file under the scripts section. This script is often tailored to start a development server, compile code, and watch for file changes. For example, it might use a tool such as Webpack, Vite, or another bundler to serve files and enable live reloading.
Here is an example of what this might look like in a package.json file:
{ "scripts": { "dev": "webpack serve --mode development" }}
When you run npm run dev
, the script executed here will start a Webpack development server in development mode, preparing your application for testing and development.
Setting Up npm run dev in Your Project
To set up npm run dev in your project, you'll first need a Node.js environment, including npm (Node Package Manager). Begin by initializing a new Node.js project using the following command:
npm init -y
This command creates a package.json file with default settings. Next, you will need to install the necessary dependencies for your project. If you are using Webpack, for instance, you can install it using:
npm install webpack webpack-cli --save-dev
Then, in the package.json file, add a dev script under the scripts section:
{ "scripts": { "dev": "webpack serve --mode development" }}
After setting this up, running the command npm run dev
in your terminal will execute the script, starting your development server.
Differences Between npm start and npm run dev
The commands npm start and npm run dev serve different purposes in a Node.js application. While both commands can be used to run scripts defined in the package.json file, they usually handle different environments.
npm start is generally used to start a production server. This command is often set up to run a script that prepares the application for production deployment. For example, it might compile the code, optimize assets, and serve the application.
In contrast, npm run dev is intended for development purposes. It is configured to run a development server, enabling features like hot reloading and error reporting to facilitate active development. The difference can be summarized as follows:
- npm start: Runs the application in production mode.
- npm run dev: Runs the application in development mode.
Related Article: How to manually install a PrimeVue component npm
Customizing the dev Script in package.json
Customizing the dev script allows developers to tailor their development experience according to project needs. Depending on the project's requirements, the dev script can include additional options and flags.
For example, if you want to specify a particular port and enable hot reloading, you can modify the script as follows:
{ "scripts": { "dev": "webpack serve --mode development --hot --port 3000" }}
In this case, --hot
enables hot module replacement, allowing modules to be updated in place without a full reload, and --port 3000
sets the server to run on port 3000.
Moreover, if you have multiple environments or configurations, you can define separate scripts for each environment. For instance:
{ "scripts": { "dev": "webpack serve --mode development", "dev:staging": "webpack serve --mode staging" }}
This setup allows you to run npm run dev
for local development and npm run dev:staging
for a staging environment.
Common Issues with npm run dev
Several common issues may arise when running npm run dev. These issues can stem from configuration errors, missing dependencies, or environmental problems.
1. Missing Dependencies: If you encounter an error stating that a module cannot be found, it may indicate that the necessary packages are not installed. Ensure you've installed all required dependencies by running:
npm install
2. Port Conflicts: If the server fails to start, there might be another service using the same port. You can change the port in your dev script or identify and stop the conflicting service.
3. Syntax Errors: JavaScript syntax issues can cause the server to crash. Check the console output for error messages indicating where the problem lies, and fix the code accordingly.
4. Configuration Errors: Issues can arise from incorrect configurations in Webpack or other tools. Review your configuration files to ensure that they are set up correctly.
5. Environment Variables: Sometimes, environment variables may not be set correctly, leading to unexpected behavior. Verify that any required environment variables are defined.
Adding Environment Variables for npm run dev
Adding environment variables to the npm run dev command can help manage different settings for various environments. Environment variables can be defined directly in the command or through a .env file.
To define environment variables directly in the npm script, you can modify the dev script as follows:
{ "scripts": { "dev": "NODE_ENV=development webpack serve --mode development" }}
This command sets the NODE_ENV variable to development while running the script. However, keep in mind that this method may not work in all operating systems, particularly Windows. For cross-platform compatibility, consider using the cross-env package:
1. Install cross-env:
npm install cross-env --save-dev
2. Modify the dev script:
{ "scripts": { "dev": "cross-env NODE_ENV=development webpack serve --mode development" }}
With this setup, you can safely set environment variables for your development server.
Tools Used with npm run dev
Several tools can enhance the development experience when using npm run dev. These tools often integrate with the development server and provide additional functionalities:
- Webpack: A popular module bundler that allows developers to bundle JavaScript modules and assets. It supports various loaders and plugins to optimize the development process.
- Babel: A JavaScript compiler that allows developers to use the latest JavaScript features while ensuring compatibility with older browsers. Learn more at Babel.
- ESLint: A linting tool that helps identify and fix problems in JavaScript code. Integrating ESLint into the dev script can ensure code quality during development.
- Prettier: A code formatter that enforces a consistent style throughout the codebase. Running Prettier automatically can improve readability and maintainability.
- Live Server: A lightweight development server that provides live reloading capabilities for static and dynamic web pages.
Integrating these tools into your development process can lead to a more productive and streamlined workflow.
Related Article: How to Fix npm Unsupported Engine Issues
Suitability of npm run dev for Production
Using npm run dev in a production environment is not recommended. The dev script is designed for development purposes and includes features like hot reloading, which are not suitable for production. Production environments require optimized and minified code without development dependencies or features that could slow down performance.
For production deployment, it is advisable to use npm start or a similar command that is specifically configured for production. This command typically runs a build step to prepare the application for deployment, ensuring that it is optimized for performance, security, and reliability.
When preparing for production, ensure that you run the appropriate build commands to create a production-ready version of your application. For example:
npm run build
This command will often compile and bundle your application, making it suitable for deployment.
Debugging Issues with npm run dev
Debugging issues when running npm run dev can involve several strategies to identify and resolve problems effectively. Here are some common approaches:
1. Console Output: Pay attention to the console output when running npm run dev. Errors and warnings are typically logged to the console, providing insights into what might be going wrong.
2. Source Maps: Enabling source maps can make debugging easier by allowing you to see the original source code in the browser's developer tools. Ensure that your build configuration includes source maps for development.
3. Browser Developer Tools: Utilize browser developer tools to inspect elements, check console logs, and debug JavaScript code. The tools provide useful features that can help identify issues in the front-end code.
4. Verbose Logging: Some tools allow enabling verbose logging to get more detailed output. This can help track down issues that might not be apparent with standard logging.
5. Debugging Tools: Many IDEs and editors have built-in debugging tools or extensions that allow you to set breakpoints and step through your code. Familiarize yourself with these tools to streamline the debugging process.
6. Reproducing the Issue: Attempt to reproduce the issue consistently. This can help narrow down the causes and lead to a solution more quickly.
Components of the dev Script
The dev script can include several components that control its behavior and functionality. Understanding these components is essential for customizing and effectively using npm run dev.
1. Command: The command specified in the dev script determines what happens when you run npm run dev. This could be a command to start a server, compile code, or run a specific tool.
2. Options: Various options can be passed to the command, such as --mode
, --port
, and --hot
. These options customize the behavior of the command, allowing developers to tailor the development experience.
3. Environment Variables: Environment variables can be passed into the script to configure different aspects of the application. They can define settings like API endpoints, feature flags, or other configuration settings.
4. Dependencies: The dev script often relies on other packages or tools installed in the project. These dependencies should be properly defined in package.json to ensure that the script runs smoothly.
5. Hooks: Some tools allow for hooks that run additional scripts before or after the main command. For example, you might want to run a linting script before starting the development server.