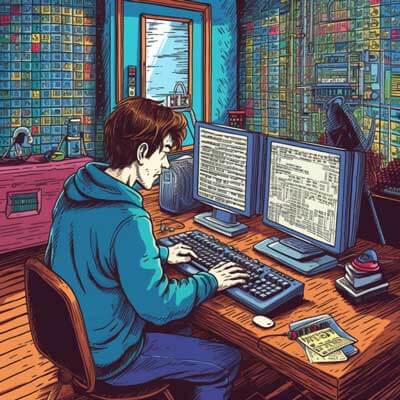
Table of Contents
Overview of Numpy Percentile Functionality
The Numpy library in Python provides a wide range of mathematical functions for efficient numerical computations. One such function is numpy.percentile(), which allows you to calculate the value below which a given percentage of data falls.
The numpy.percentile() function takes in an array and a percentile value as input and returns the value at that percentile. It is a useful tool in data analysis and can be used to understand the distribution and spread of data.
In this article, we will explore the functionality of numpy.percentile() and learn how to use it in Python.
Related Article: How To Use Ternary Operator In Python
Working with Arrays in Numpy
Before diving into the details of numpy.percentile(), let's first understand how to work with arrays in Numpy. Numpy provides a multidimensional array object called ndarray, which is a useful data structure for efficient storage and manipulation of large datasets.
To create a Numpy array, you can use the np.array() function and pass in a list or tuple of values. Here's an example:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) print(arr)
Output:
[1 2 3 4 5]
Numpy arrays can be of any dimension, from one-dimensional arrays to multi-dimensional arrays. You can access and manipulate the elements of a Numpy array using indexing and slicing.
Calculating the Mean of a Numpy Array
The mean of a set of numbers is the sum of all the numbers divided by the total count. In Numpy, you can calculate the mean of a Numpy array using the np.mean() function.
Here's an example that demonstrates how to calculate the mean of a Numpy array:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) # Calculate the mean mean = np.mean(arr) print(mean)
Output:
3.0
In this example, we created a Numpy array called arr
with values [1, 2, 3, 4, 5]
. We then used the np.mean() function to calculate the mean of the array, which is 3.0.
Exploring the Median in Numpy
The median is the middle value of a dataset when it is sorted in ascending order. In Numpy, you can calculate the median of a Numpy array using the np.median() function.
Let's see an example of how to calculate the median of a Numpy array:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) # Calculate the median median = np.median(arr) print(median)
Output:
3.0
In this example, we created a Numpy array called arr
with values [1, 2, 3, 4, 5]
. We then used the np.median() function to calculate the median of the array, which is also 3.0.
It is important to note that if the dataset has an odd number of elements, the median will be the middle value. However, if the dataset has an even number of elements, the median will be the average of the two middle values.
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
Standard Deviation Calculation in Numpy
The standard deviation is a measure of the spread or dispersion of a dataset. It indicates how much the values deviate from the mean. In Numpy, you can calculate the standard deviation of a Numpy array using the np.std() function.
Here's an example that demonstrates how to calculate the standard deviation of a Numpy array:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) # Calculate the standard deviation std_dev = np.std(arr) print(std_dev)
Output:
1.4142135623730951
In this example, we created a Numpy array called arr
with values [1, 2, 3, 4, 5]
. We then used the np.std() function to calculate the standard deviation of the array, which is approximately 1.4142135623730951.
The standard deviation provides valuable insights into the spread of the data. A higher standard deviation indicates a greater spread, while a lower standard deviation indicates a narrower distribution.
Code Snippet: How to Calculate the Mean of a Numpy Array
To calculate the mean of a Numpy array, you can use the np.mean() function. Here's a code snippet that demonstrates how to do it:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) # Calculate the mean mean = np.mean(arr) print(mean)
Output:
3.0
In this code snippet, we created a Numpy array called arr
with values [1, 2, 3, 4, 5]
. We then used the np.mean() function to calculate the mean of the array, which is 3.0.
Key Differences Between Mean and Median in Numpy
While both the mean and median provide insights into the central tendency of a dataset, they represent different aspects of the data.
The mean is the average of all the values in the dataset and is affected by outliers. It gives equal weight to all the values. On the other hand, the median is the middle value of the dataset, and it is not affected by outliers. It gives more weight to the central values.
Here's an example that demonstrates the difference between the mean and median:
import numpy as np # Create a Numpy array with outliers arr = np.array([1, 2, 3, 4, 1000]) # Calculate the mean and median mean = np.mean(arr) median = np.median(arr) print("Mean:", mean) print("Median:", median)
Output:
Mean: 202.0 Median: 3.0
In this example, we created a Numpy array called arr
with values [1, 2, 3, 4, 1000]
. The mean of the array is significantly influenced by the outlier value of 1000, resulting in a mean of 202.0. However, the median remains unaffected by the outlier and remains 3.0.
Code Snippet: How to Calculate the Standard Deviation of a Numpy Array
To calculate the standard deviation of a Numpy array, you can use the np.std() function. Here's a code snippet that demonstrates how to do it:
import numpy as np # Create a Numpy array arr = np.array([1, 2, 3, 4, 5]) # Calculate the standard deviation std_dev = np.std(arr) print(std_dev)
Output:
1.4142135623730951
In this code snippet, we created a Numpy array called arr
with values [1, 2, 3, 4, 5]
. We then used the np.std() function to calculate the standard deviation of the array, which is approximately 1.4142135623730951.
The standard deviation provides valuable information about the spread of the data. A higher standard deviation indicates a greater spread, while a lower standard deviation indicates a narrower distribution.
Related Article: Calculating Averages with Numpy in Python
Additional Resources
- Calculating the mean of a numpy array