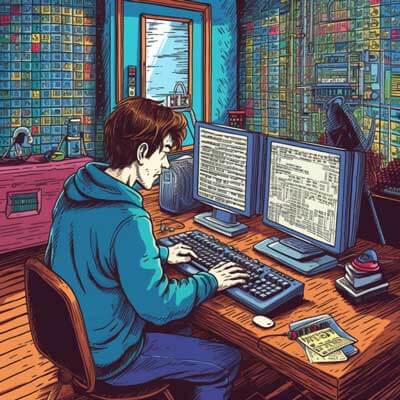
Table of Contents
Using PHP cURL for HTTP POST
Related Article: Optimizing Laravel Apps: Deployment and Scaling Techniques
Introduction
In PHP, cURL is a library that allows you to make HTTP requests. It supports various protocols, including HTTP, HTTPS, FTP, and more. With cURL, you can easily send data to a server using the POST method. This can be useful for submitting forms, uploading files, or interacting with APIs.
Step 1: Installing cURL
Before using cURL in PHP, you need to ensure that the cURL extension is installed and enabled in your PHP environment. Most PHP installations come with cURL pre-installed, but if it's missing, you can install it by following these steps:
1. For Linux-based systems, open a terminal and run the following command:
sudo apt-get install php-curl
2. For Windows, open your php.ini file (located in the PHP installation directory) and uncomment the line ;extension=curl
by removing the semicolon at the beginning of the line. Then, save the file and restart your web server.
Step 2: Making a Basic POST Request
To make a POST request using cURL in PHP, you need to follow these steps:
1. Initialize a cURL session using the curl_init()
function:
$ch = curl_init();
2. Set the URL to which you want to send the POST request using the curl_setopt()
function:
curl_setopt($ch, CURLOPT_URL, 'https://example.com/api');
3. Set the request method to POST using the curl_setopt()
function:
curl_setopt($ch, CURLOPT_POST, true);
4. Set the data to be sent in the POST request using the curl_setopt()
function:
$data = array( 'name' => 'John Doe', 'email' => 'john.doe@example.com', ); curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
5. Execute the POST request using the curl_exec()
function:
$result = curl_exec($ch);
6. Close the cURL session using the curl_close()
function:
curl_close($ch);
Related Article: How To Fix the “Array to string conversion” Error in PHP
Step 3: Handling Response and Error Checking
After executing the POST request, you can handle the response and check for any errors. Here's an example of how you can do it:
if ($result === false) { // An error occurred $error = curl_error($ch); // Handle the error } else { // Request was successful // Handle the response }
You can also retrieve additional information about the request and response, such as the HTTP status code and headers. Here's an example:
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE); $responseHeaders = curl_getinfo($ch, CURLINFO_HEADER_OUT);
Step 4: Best Practices and Additional Options
When using cURL for POST requests in PHP, consider the following best practices and additional options:
1. Use HTTPS for secure communication whenever possible. This can be done by setting the URL with the https://
prefix.
2. Handle errors gracefully by checking for errors using curl_error()
and curl_errno()
functions.
3. Set the CURLOPT_RETURNTRANSFER
option to true
to return the response as a string instead of outputting it directly. This allows you to process the response further.
4. Set the CURLOPT_FOLLOWLOCATION
option to true
to automatically follow redirects.
5. If you need to send JSON data in the POST request, you can use the json_encode()
function to encode the data before setting it with CURLOPT_POSTFIELDS
.
6. Use the CURLOPT_HTTPHEADER
option to set custom headers in the request. For example:
$headers = array( 'Content-Type: application/json', 'Authorization: Bearer your_token', ); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
7. If you need to upload a file in the POST request, use the CURLOPT_POSTFIELDS
option with the @
symbol followed by the file path. For example:
$data = array( 'file' => '@path/to/file.jpg', ); curl_setopt($ch, CURLOPT_POSTFIELDS, $data);