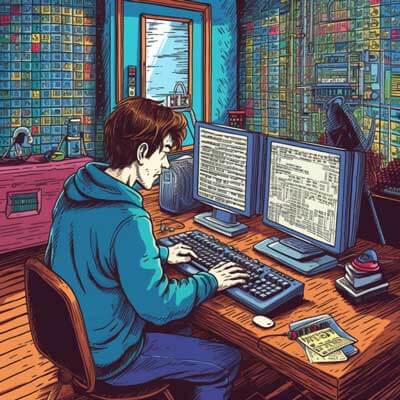
Table of Contents
Overview of Math GCD
The term GCD stands for "Greatest Common Divisor." It refers to the largest positive integer that divides two or more integers without leaving a remainder. For example, the GCD of 8 and 12 is 4, as 4 is the highest number that divides both 8 and 12 evenly. Calculating the GCD is important in various applications, including simplifying fractions, number theory, and algorithms such as those used in cryptography.
Related Article: How to Convert a String to Dictionary in Python
Importing the Math Module in Python
To use the GCD function in Python, the first step is to import the math module, which contains various mathematical functions, including GCD. This can be accomplished by writing a simple import statement at the beginning of your Python script.
import math
After executing this import statement, all functions available in the math module, including GCD, can be accessed using the syntax math.function_name
.
Purpose of Math.gcd
The function math.gcd
serves to compute the GCD of two integers. This function is particularly useful when working with mathematical calculations that require simplification of ratios or when analyzing relationships between numbers. It provides a built-in and optimized way to obtain the GCD, which can be more efficient than implementing a custom solution.
Calculating GCD Using Math Module
To calculate the GCD using the math
module, you can call the gcd
function with two integer arguments. The following example demonstrates how to compute the GCD of two numbers using Python.
import math num1 = 48 num2 = 18 gcd_value = math.gcd(num1, num2) print(f"The GCD of {num1} and {num2} is {gcd_value}.")
The output will display the GCD of the specified numbers.
Related Article: How to Use the IsAlpha Function in Python
Parameters of Math.gcd
The math.gcd
function accepts two parameters, both of which must be integers. If you pass non-integer values, Python will raise a TypeError. Here is a breakdown of the parameters:
- a: The first integer.
- b: The second integer.
Both parameters can be positive or negative integers. The function calculates the GCD based on their absolute values.
Handling Negative Numbers with Math.gcd
When working with negative numbers, the math.gcd
function still produces a valid output. It calculates the GCD based on the absolute values of the provided integers. For instance:
import math num1 = -48 num2 = 18 gcd_value = math.gcd(num1, num2) print(f"The GCD of {num1} and {num2} is {gcd_value}.")
In this case, the output will still show the GCD as 6, ignoring the negative sign.
Return Type of Math.gcd
The return type of the math.gcd
function is always an integer. It returns the greatest common divisor between the two integer inputs. If both inputs are zero, the behavior is defined, and the function returns 0, as mathematically, the GCD of zero is considered to be zero.
Comparison with Custom GCD Implementation
Implementing a custom GCD function can be done using various algorithms such as the Euclidean algorithm. Here is a simple custom implementation:
def custom_gcd(a, b): while b: a, b = b, a % b return abs(a) num1 = 48 num2 = 18 print(f"The GCD calculated by custom function is {custom_gcd(num1, num2)}.")
While this custom function works effectively, using math.gcd
is generally preferred due to its optimization and built-in nature, which saves time and reduces the potential for errors.
Related Article: How to Add Multilingual Support to Django Apps
Using Math.gcd with Multiple Numbers
The math.gcd
function is designed to compute the GCD of two integers at a time. However, if you need to find the GCD of more than two numbers, you can apply the function iteratively with the help of the functools.reduce method. Here’s an example:
from functools import reduce import math numbers = [48, 64, 16] gcd_value = reduce(math.gcd, numbers) print(f"The GCD of {numbers} is {gcd_value}.")
This method effectively reduces the list of numbers by applying the GCD function repeatedly until a single GCD value remains.
Time Complexity of Math.gcd
The time complexity of the math.gcd
function is O(log(min(a, b))), where a
and b
are the two numbers for which the GCD is being computed. This logarithmic time complexity arises from the Euclidean algorithm used internally by the math.gcd
function, making it efficient for large numbers.
Alternatives to Math.gcd in Python
While math.gcd
is a convenient and efficient choice for computing the GCD, there are other alternatives available in Python. For instance, you can implement the GCD using the built-in fractions module, which can also simplify fractions. Here’s how:
from fractions import Fraction fraction = Fraction(48, 18) gcd_value = fraction.denominator print(f"The GCD of 48 and 18 using fractions is {gcd_value}.")
This method is not as direct as using math.gcd
, but it shows that Python offers various ways to achieve similar results, depending on your specific requirements.