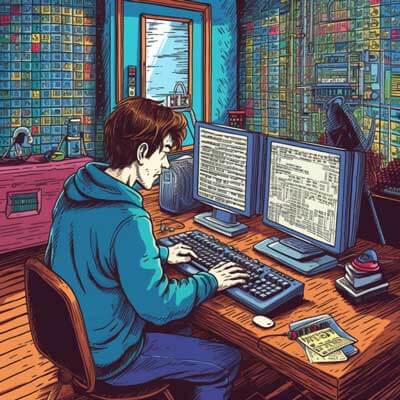
Table of Contents
Overview
Integer division refers to the division of two numbers where the result is an integer. This means that the division operation will produce the quotient as a whole number, without any decimal places. Python provides the floor division operator (//) to perform integer division.
Related Article: How to Use Pandas Groupby for Group Statistics in Python
The Concept of Floor Division
Floor division is a mathematical operation that rounds the quotient of a division down to the nearest whole number. It is denoted by the double forward slash (//) operator in Python. The floor division operator always returns an integer result, regardless of the operands.
Let's take an example to understand floor division better. Consider the following code snippet:
result = 10 // 3 print(result)
The output of the above code will be 3. Here, the floor division operation divides 10 by 3 and rounds down the result to the nearest whole number, which is 3.
The Quotient in Integer Division
In integer division, the quotient refers to the result of the division operation. It is the whole number part of the division. The floor division operator in Python returns the quotient as the result.
To calculate the quotient using integer division, you can use the floor division operator (//) as shown in the previous example.
Truncation in Integer Division
Truncation is the process of discarding the decimal part of a number, resulting in a whole number. In Python's integer division, truncation occurs automatically as the result is always an integer.
Let's consider an example to illustrate truncation in integer division:
result = 7 // 2 print(result)
The output of the above code will be 3. Here, the division operation 7 // 2 truncates the decimal part of the result, giving us the whole number 3.
Related Article: How To List All Files Of A Directory In Python
Handling the Divisor in Integer Division
In integer division, the divisor refers to the number by which another number is divided. When performing integer division in Python, it is important to consider the divisor and its implications on the result.
If the divisor is zero, Python will raise a ZeroDivisionError. This is because dividing any number by zero is undefined in mathematics.
To handle the divisor in integer division, you can add conditional statements to check if the divisor is zero before performing the division operation. Here's an example:
divisor = 0 if divisor == 0: print("Error: Division by zero") else: result = 10 // divisor print(result)
This code snippet checks if the divisor is zero before performing the division operation. If the divisor is zero, it prints an error message. Otherwise, it performs the division operation and prints the result.
Dealing with the Remainder in Integer Division
In integer division, the remainder refers to the decimal part of the division operation. Since integer division truncates the decimal part, the remainder is discarded.
To calculate the remainder in integer division, you can use the modulo operator (%) in Python. The modulo operator returns the remainder of the division operation.
Let's consider an example to illustrate dealing with the remainder in integer division:
result = 10 % 3 print(result)
The output of the above code will be 1. Here, the modulo operation 10 % 3 calculates the remainder of the division, which is 1.
Comparison of Integer Division and Regular Division in Python
In Python, regular division (/) returns the quotient as a floating-point number, with decimal places if necessary. On the other hand, integer division (//) returns the quotient as an integer, rounding down to the nearest whole number.
Let's compare regular division and integer division with an example:
result1 = 10 / 3 result2 = 10 // 3 print(result1) print(result2)
The output of the above code will be:
3.3333333333333335 3
Here, regular division (10 / 3) returns a floating-point number with decimal places, while integer division (10 // 3) returns the whole number part of the division.
Result of Integer Division in Python
The result of integer division in Python is always an integer, rounded down to the nearest whole number. This means that any decimal places in the division result are discarded.
To obtain the result of integer division, you can use the floor division operator (//) in Python.
Related Article: How to Create and Fill an Empty Pandas DataFrame in Python
Strategies for Handling Remainder in Integer Division
When dealing with the remainder in integer division, there are several strategies you can employ based on your specific requirements.
One common strategy is to use the modulo operator (%) to calculate the remainder explicitly. This allows you to perform additional operations or make decisions based on the remainder value.
Another strategy is to ignore the remainder altogether if it is not needed for your calculations. Since integer division discards the remainder, you can simply focus on the quotient and disregard the decimal part.
Additionally, you can use the divmod() function in Python to obtain both the quotient and the remainder of an integer division in a single operation. The divmod() function returns a tuple containing the quotient and the remainder.
Here's an example of using the divmod() function:
result = divmod(10, 3) print(result)
The output of the above code will be (3, 1). Here, divmod(10, 3) calculates the quotient and remainder of the division, returning them as a tuple.
Additional Resources
- Understanding Division in Python