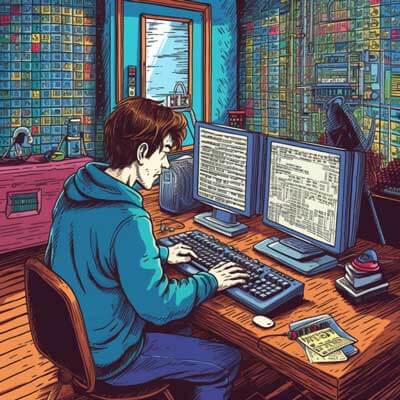
Table of Contents
Overview of numpy linspace function
The numpy linspace function is a useful tool in Python for creating arrays with evenly spaced values. It allows you to specify the start and end points of the array, as well as the number of values you want in between. This function is particularly useful when you need to generate a set of values that are evenly distributed over a specified range.
Related Article: How to Use and Import Python Modules
Creating linearly spaced array using numpy
To create a linearly spaced array using numpy, you can use the linspace function. The syntax for this function is as follows:
numpy.linspace(start, stop, num=50, endpoint=True, retstep=False, dtype=None, axis=0)
Let's break down each parameter:
- start: This is the starting value of the sequence.
- stop: This is the end value of the sequence.
- num: This is the number of equally spaced samples to generate. By default, it is set to 50.
- endpoint: This parameter determines whether to include the endpoint in the sequence. If set to True, the endpoint is included. If set to False, the endpoint is not included. By default, it is set to True.
- retstep: This parameter determines whether to return the step size between the samples. If set to True, the step size is returned. If set to False, only the array of samples is returned. By default, it is set to False.
- dtype: This parameter specifies the data type of the output array. If not specified, it is determined automatically based on the input values.
- axis: This parameter specifies the axis along which the array is created. By default, it is set to 0.
Let's look at a few examples to better understand how to use the linspace function.
Example 1: Generate an array with 10 equally spaced values between 0 and 1.
import numpy as np arr = np.linspace(0, 1, num=10) print(arr)
Output:
[0. 0.11111111 0.22222222 0.33333333 0.44444444 0.55555556 0.66666667 0.77777778 0.88888889 1. ]
In this example, the linspace function generates an array with 10 equally spaced values between 0 and 1, including the endpoint.
Example 2: Generate an array with 5 equally spaced values between -1 and 1, excluding the endpoint.
import numpy as np arr = np.linspace(-1, 1, num=5, endpoint=False) print(arr)
Output:
[-1. -0.5 0. 0.5 1. ]
In this example, the linspace function generates an array with 5 equally spaced values between -1 and 1, excluding the endpoint.
The endpoint parameter in numpy linspace
The endpoint parameter in the numpy linspace function determines whether to include the endpoint in the generated sequence. By default, it is set to True, which means the endpoint is included. However, if you set it to False, the endpoint will not be included in the sequence.
Including the endpoint can be useful in some scenarios, such as when you want to generate a set of values that span a specific range and include the maximum value. On the other hand, excluding the endpoint can be useful when you want to generate a set of values that span a range but exclude the maximum value.
Let's look at an example to illustrate the purpose of the endpoint parameter.
Example: Generate an array with 7 equally spaced values between 0 and 1, including the endpoint.
import numpy as np arr = np.linspace(0, 1, num=7, endpoint=True) print(arr)
Output:
[0. 0.16666667 0.33333333 0.5 0.66666667 0.83333333 1. ]
In this example, the linspace function generates an array with 7 equally spaced values between 0 and 1, including the endpoint. The endpoint value of 1 is included in the sequence.
Now, let's modify the example to exclude the endpoint.
Example: Generate an array with 7 equally spaced values between 0 and 1, excluding the endpoint.
import numpy as np arr = np.linspace(0, 1, num=7, endpoint=False) print(arr)
Output:
[0. 0.14285714 0.28571429 0.42857143 0.57142857 0.71428571 0.85714286]
In this modified example, the linspace function generates an array with 7 equally spaced values between 0 and 1, excluding the endpoint. The endpoint value of 1 is not included in the sequence.
The concept of step in numpy linspace
The concept of step in the numpy linspace function refers to the difference between consecutive values in the generated sequence. It represents the increment or decrement between each value in the array.
Let's look at an example to better understand the concept of step in numpy linspace.
Example: Generate an array with 5 equally spaced values between 0 and 10 and return the step size.
import numpy as np arr, step_size = np.linspace(0, 10, num=5, retstep=True) print(arr) print(step_size)
Output:
[ 0. 2.5 5. 7.5 10. ] 2.5
In this example, the linspace function generates an array with 5 equally spaced values between 0 and 10. The step size between each value is 2.5, which is returned by the function.
Understanding the concept of step in numpy linspace can be helpful when you need to perform calculations or operations that depend on the increment or decrement between values in the array.
Related Article: How to use Python's Integer Division
Additional Resources
- NumPy linspace