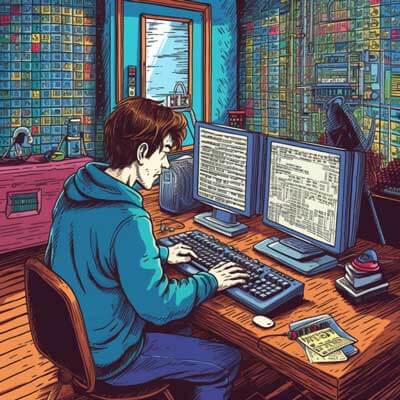
Table of Contents
Regular expressions (regex) are a useful tool for pattern matching and searching in strings. By default, regex patterns are case sensitive, meaning that they will only match characters that have the same case as specified in the pattern. However, in some cases, you may want to perform a case-insensitive match, where the pattern will match characters regardless of their case. In this guide, we will discuss how to use regex for case-insensitive matching in various programming languages.
1. Regex Case Insensitive Flag
One common approach to perform case-insensitive matching with regex is to use a flag or modifier that indicates case insensitivity. Different programming languages and regex engines have different ways of specifying this flag, but it is usually denoted by the letter "i". Here are examples of how to use the case-insensitive flag in different programming languages:
JavaScript:
In JavaScript, you can use the i
flag to perform a case-insensitive match. This flag can be added to the regex pattern by appending it at the end of the pattern:
const regex = /pattern/i;
Python:
In Python, you can use the re.IGNORECASE
flag to perform a case-insensitive match. This flag can be passed as the second argument to the re.compile()
function or directly to the re.search()
or re.match()
functions:
import re pattern = re.compile("pattern", re.IGNORECASE)
PHP:
In PHP, you can use the i
flag to perform a case-insensitive match. This flag can be added to the regex pattern by appending it at the end of the pattern, or by using the preg_match()
function with the PREG_CASELESS
flag:
$pattern = "/pattern/i";
Related Article: Visualizing Binary Search Trees: Deep Dive
2. Character Class
Another way to perform case-insensitive matching is by using character class. Character classes allow you to specify a set of characters that can match a single character in the input string. By including both uppercase and lowercase letters in the character class, you can achieve case-insensitive matching. Here's an example:
const regex = /[Pp]attern/;
In this example, the character class [Pp]
matches either "P" or "p", making the match case-insensitive.
Best Practices
Related Article: How To Use A Regex To Only Accept Numbers 0-9
When using regex for case-insensitive matching, it is important to consider the following best practices:
1. Use the case-insensitive flag or modifier provided by your programming language or regex engine whenever possible. This is the most straightforward and efficient way to achieve case-insensitive matching.
2. If your regex engine does not support a case-insensitive flag, consider using character classes to specify both uppercase and lowercase versions of the characters you want to match.
3. Be cautious when using case-insensitive matching in situations where the case of the matched string is significant. For example, if you are performing a case-insensitive search on a user's username, you should be careful to handle potential conflicts between usernames that only differ in case.
4. Test your regular expressions thoroughly to ensure that they are working as expected. Use sample input strings that cover different cases (both uppercase and lowercase) to verify the behavior of your regex pattern.