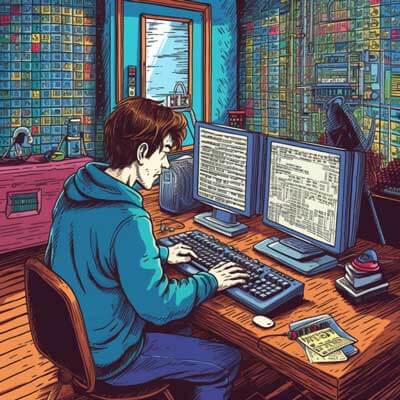
Table of Contents
Introduction to Selenium WebDriver
Selenium WebDriver is a powerful open-source tool for automating web browsers. It allows software engineers to write automated tests in various programming languages, such as Java, Python, C#, etc., to verify the functionality of web applications. WebDriver provides a user-friendly API that enables developers to interact with web elements, navigate through web pages, and perform actions like clicking buttons, filling out forms, and submitting data.
Related Article: What is Test-Driven Development? (And How To Get It Right)
Example 1: Basic WebDriver Test
import org.openqa.selenium.WebDriver;import org.openqa.selenium.chrome.ChromeDriver;public class BasicWebDriverTest { public static void main(String[] args) { // Set the path to the ChromeDriver executable System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); // Create a new instance of ChromeDriver WebDriver driver = new ChromeDriver(); // Open a web page driver.get("https://www.example.com"); // Verify the page title String pageTitle = driver.getTitle(); System.out.println("Page Title: " + pageTitle); // Close the browser driver.quit(); }}
Example 2: Basic WebDriver Test with Firefox
from selenium import webdriver# Create a new instance of Firefox WebDriverdriver = webdriver.Firefox()# Open a web pagedriver.get("https://www.example.com")# Verify the page titlepage_title = driver.titleprint("Page Title:", page_title)# Close the browserdriver.quit()
Setting up Selenium WebDriver
Before using Selenium WebDriver, you need to set up the necessary dependencies and drivers for the specific browser you want to automate. Here, we'll cover the setup process for Chrome and Firefox.
Related Article: Smoke Testing Best Practices: How to Catch Critical Issues Early
Setting up WebDriver for Chrome
To use WebDriver with Chrome, you need to download and configure the ChromeDriver executable. Follow these steps:
1. Download the ChromeDriver executable from the official website: ChromeDriver Downloads.
2. Extract the downloaded archive.
3. Set the system property "webdriver.chrome.driver" to the path of the ChromeDriver executable.
Example: Setting up ChromeDriver in Java
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");WebDriver driver = new ChromeDriver();
Example: Setting up ChromeDriver in Python
from selenium import webdriverdriver = webdriver.Chrome(executable_path="path/to/chromedriver")
Setting up WebDriver for Firefox
To use WebDriver with Firefox, you need to download and configure the GeckoDriver executable. Follow these steps:
1. Download the GeckoDriver executable from the official website: GeckoDriver Releases.
2. Extract the downloaded archive.
3. Set the system property "webdriver.gecko.driver" to the path of the GeckoDriver executable.
Example: Setting up GeckoDriver in Java
System.setProperty("webdriver.gecko.driver", "path/to/geckodriver");WebDriver driver = new FirefoxDriver();
Example: Setting up GeckoDriver in Python
from selenium import webdriverdriver = webdriver.Firefox(executable_path="path/to/geckodriver")
Elements of Selenium WebDriver
WebDriver provides a rich set of methods to interact with various web elements on a web page. Here are some of the commonly used methods:
- findElement(By by)
: Locates a single web element using the specified locator strategy.
- findElements(By by)
: Locates multiple web elements using the specified locator strategy.
- click()
: Clicks on the web element.
- sendKeys(CharSequence... keysToSend)
: Sends keys to the web element (e.g., for text input fields).
- getText()
: Retrieves the visible text of the web element.
- getAttribute(String name)
: Retrieves the value of the specified attribute of the web element.
- isEnabled()
: Checks if the web element is enabled.
- isSelected()
: Checks if the web element is selected (e.g., for checkboxes or radio buttons).
- submit()
: Submits a form containing the web element.
Example 1: Finding and Clicking a Button
import org.openqa.selenium.By;import org.openqa.selenium.WebDriver;import org.openqa.selenium.WebElement;import org.openqa.selenium.chrome.ChromeDriver;public class ButtonClickExample { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); driver.get("https://www.example.com"); // Find the button element and click it WebElement button = driver.findElement(By.cssSelector("button")); button.click(); driver.quit(); }}
Example 2: Filling out a Form
from selenium import webdriverfrom selenium.webdriver.common.keys import Keysdriver = webdriver.Chrome(executable_path="path/to/chromedriver")driver.get("https://www.example.com")# Find the input field and enter textinput_field = driver.find_element_by_css_selector("input[type='text']")input_field.send_keys("Hello, World!")# Find the submit button and press Enter keysubmit_button = driver.find_element_by_css_selector("input[type='submit']")submit_button.send_keys(Keys.ENTER)driver.quit()