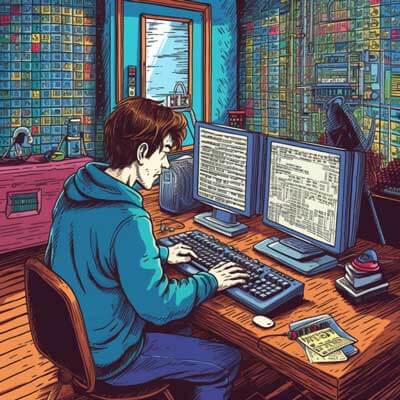
Table of Contents
The setTimeout function in JavaScript is a useful tool for delaying actions, and when combined with jQuery, it becomes even more versatile. In this article, we will explore how to use setTimeout for delaying jQuery actions in different scenarios.
1. Delaying a Single JQuery Action
To delay a single jQuery action, you can use the setTimeout function to set a delay before executing the action. Here is an example:
setTimeout(function() { // Your jQuery action here $('element').fadeIn(); }, 2000); // Delay in milliseconds
In this example, the jQuery action to fade in the element will be executed after a delay of 2000 milliseconds (or 2 seconds). You can adjust the delay according to your requirements.
Related Article: Invoking Angular Component Functions via JavaScript
2. Delaying Multiple JQuery Actions
If you need to delay multiple jQuery actions, you can use the setTimeout function in combination with a loop or a callback function. Here are two examples:
Using a Loop:
var actions = [ function() { $('element1').fadeIn(); }, function() { $('element2').fadeOut(); }, // Add more actions here ]; for (var i = 0; i < actions.length; i++) { setTimeout(actions[i], i * 1000); // Delay each action by 1 second }
In this example, each action is stored as a function in an array. The setTimeout function is called within a loop to execute each action with a delay of 1 second between them. You can adjust the delay time by modifying the value of i * 1000
.
Using a Callback Function:
function delayAction(action, delay) { setTimeout(action, delay); } function action1() { $('element1').fadeIn(); } function action2() { $('element2').fadeOut(); } // Delay action1 by 2 seconds and action2 by 4 seconds delayAction(action1, 2000); delayAction(action2, 4000);
In this example, the delayAction
function is defined to accept an action (a jQuery function) and a delay time. The setTimeout function is called within delayAction
to execute the action with the provided delay.
Best Practices and Suggestions
- When using setTimeout to delay jQuery actions, it is important to consider the overall performance and user experience. Avoid using long delays that may make the website or application appear unresponsive.
- If you need to cancel a delayed action, you can use the clearTimeout function to clear the timer. For example:
var timer = setTimeout(function() { // Your code here }, 2000); clearTimeout(timer); // Cancel the delayed action
- To ensure smoother animations and transitions, consider using CSS transitions or animations instead of relying solely on setTimeout. CSS transitions and animations are hardware-accelerated and can provide better performance.
- If you are working with asynchronous operations, such as AJAX requests or data fetching, you may need to handle the delays and actions within the callback functions of those operations. Be mindful of the order and timing of your actions to avoid unexpected behavior.
- It is important to test and debug your code when using setTimeout for delaying jQuery actions. Use browser developer tools to inspect the timing and behavior of your actions.
Alternative Approach: jQuery Delay Method
In addition to using setTimeout, jQuery provides a built-in delay method that can be used to delay jQuery actions. Here is an example:
$('element').delay(2000).fadeIn();
In this example, the delay method is called on the jQuery object, specifying the delay time in milliseconds. The fadeIn method is then called after the specified delay.
The jQuery delay method can be useful for simple delay scenarios, but it may not be as flexible as using setTimeout directly. Consider your specific requirements and choose the approach that best suits your needs.