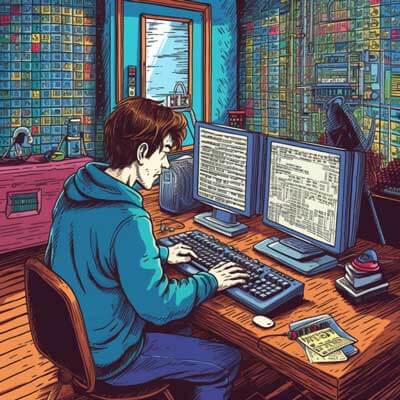
Table of Contents
To remove specific characters from a string in Python, you can use the strip()
method. This method allows you to remove leading and trailing characters from a string. However, if you want to remove characters from anywhere within the string, you can use a combination of other methods and functions.
Using the strip() Method
The strip()
method is a built-in function in Python that can remove leading and trailing characters from a string. By default, it removes whitespace characters such as spaces, tabs, and newlines. However, you can specify the characters you want to remove by passing them as an argument to the strip()
method.
Here's an example that demonstrates how to use the strip()
method to remove specific characters from a string:
string = "Hello, World!" new_string = string.strip("H!") print(new_string) # Output: "ello, World"
In the example above, we have a string "Hello, World!"
. By calling the strip()
method with the argument "H!"
, we are instructing Python to remove any leading or trailing occurrences of the characters 'H'
and '!'
from the string. The resulting string is "ello, World"
.
Related Article: How to Append to Strings in Python
Using the replace() Method
If you want to remove specific characters from anywhere within a string, you can use the replace()
method. The replace() method allows you to replace occurrences of a substring with another substring. By specifying an empty string as the replacement substring, you can effectively remove the specified characters.
Here's an example that demonstrates how to use the replace()
method to remove specific characters from a string:
string = "Hello, World!" new_string = string.replace("H", "").replace("!", "") print(new_string) # Output: "ello, World"
In the example above, we have a string "Hello, World!"
. By calling the replace()
method twice, we are replacing occurrences of the characters 'H'
and '!'
with an empty string. This effectively removes these characters from the string, resulting in "ello, World"
.
Using Regular Expressions
If you need more advanced pattern matching capabilities to remove specific characters from a string, you can use regular expressions. The re module in Python provides functions for working with regular expressions.
Here's an example that demonstrates how to use regular expressions to remove specific characters from a string:
import re string = "Hello, World!" pattern = r"[H!]" new_string = re.sub(pattern, "", string) print(new_string) # Output: "ello, World"
In the example above, we import the re
module and define a regular expression pattern [H!]
using the r
prefix. This pattern matches either the character 'H'
or '!'
. We then use the re.sub()
function to substitute occurrences of this pattern with an empty string in the original string. The resulting string is "ello, World"
.
Additional Considerations
- If you want to remove multiple different characters from a string, you can chain multiple replace() or strip()
method calls.
- When using the strip()
method, be aware that it removes characters only from the beginning and end of the string. If you want to remove characters from anywhere within the string, you should use other methods or functions.
- If you are working with large strings or need to perform complex string manipulations, consider using the re
module and regular expressions for more flexible pattern matching and substitution.
- Remember to assign the modified string to a new variable or the same variable if you want to overwrite the original string.