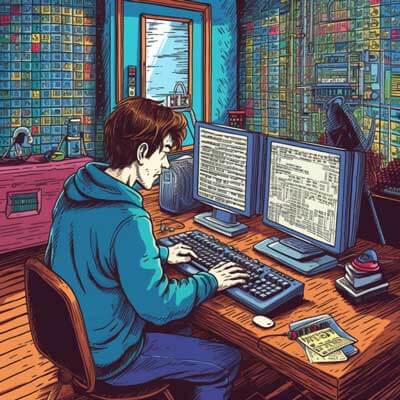
Table of Contents
The Terser Webpack Plugin is a tool designed for minimizing JavaScript code during the build process of applications using Webpack. It leverages Terser, a JavaScript parser and mangler/compressor toolkit, to reduce the file size of JavaScript bundles. This process is crucial for optimizing web applications, as smaller file sizes lead to faster load times and improved performance.
Terser performs several tasks such as removing whitespace, shortening variable names, and eliminating dead code. By integrating Terser with Webpack, developers can automate the minification process, ensuring that their code is always optimized for production environments.
What is the Terser Webpack Plugin Used For
The primary purpose of the Terser Webpack Plugin is to minimize JavaScript files. This is particularly important for production builds where performance is critical. Minification reduces the overall size of the JavaScript files, which is beneficial for several reasons:
1. Faster Load Times: Smaller files transfer faster over the internet, leading to quicker page loads.
2. Reduced Bandwidth Usage: Minimizing file sizes can significantly reduce the amount of data that needs to be transferred, which is especially beneficial for users with limited bandwidth.
3. Improved Performance: Smaller files mean less processing time for browsers, which can lead to smoother user experiences.
The plugin is often used in conjunction with other Webpack features like code splitting and tree shaking to maximize optimization.
Related Article: How to Use the Fork TS Checker Webpack Plugin
Benefits of Using Terser for JavaScript Optimization
Terser offers several advantages for JavaScript code optimization:
1. Advanced Minification: Terser uses advanced algorithms to reduce code size while maintaining functionality. It can perform variable renaming, dead code elimination, and more.
2. Support for ES6+: Unlike some older minifiers, Terser supports modern JavaScript syntax, making it suitable for applications that use ES6 and beyond.
3. Customizable Options: Terser provides a wide range of options for developers to configure the minification process according to their needs.
4. Source Map Support: Terser can generate source maps, allowing developers to debug minified code easily.
5. Active Maintenance: Terser is actively maintained and updated, ensuring compatibility with the latest JavaScript features and performance improvements.
Configuration of the Terser Webpack Plugin
To set up the Terser Webpack Plugin, you need to install it and then configure it within your Webpack configuration file. First, install the plugin via npm:
npm install terser-webpack-plugin --save-dev
Next, you will need to modify your Webpack configuration file, typically named webpack.config.js
. Here’s a basic example:
// webpack.config.jsconst TerserPlugin = require('terser-webpack-plugin');module.exports = { mode: 'production', optimization: { minimize: true, minimizer: [new TerserPlugin()], }, // other configurations...};
In this configuration, setting mode
to 'production'
automatically enables optimizations, including minification, but specifying the TerserPlugin allows for more control.
Available Options for Terser Webpack Plugin
Terser Webpack Plugin provides various options to customize the minification process. Here are some key options:
1. parallel
: Enables parallel processing for faster builds. Set it to true
to use multiple CPU cores.
2. sourceMap
: If set to true
, source maps will be generated for debugging purposes.
3. terserOptions
: This allows you to pass specific options to Terser, such as:
- compress
: Enables compression options.
- mangle
: Controls variable name mangling.
- output
: Controls the output format.
Example of configuring options:
// webpack.config.jsconst TerserPlugin = require('terser-webpack-plugin');module.exports = { mode: 'production', optimization: { minimize: true, minimizer: [ new TerserPlugin({ parallel: true, terserOptions: { compress: { drop_console: true, // Removes console logs }, mangle: true, output: { comments: false, // Removes comments }, }, sourceMap: true, }), ], },};
Related Article: How to Use Webpack Node Externals
Enabling Source Maps with Terser
Enabling source maps is essential for debugging minified code. Terser can generate source maps that map the minified code back to the original source code, which can be invaluable when troubleshooting issues in production.
To enable source maps, set the sourceMap
option to true
in the Terser configuration. Ensure that your Webpack configuration also supports source maps by including the appropriate devtool
option:
// webpack.config.jsmodule.exports = { mode: 'production', devtool: 'source-map', // Enables source maps for the build optimization: { minimize: true, minimizer: [ new TerserPlugin({ sourceMap: true, }), ], },};
With this setup, you will be able to view the original code in your browser's developer tools, even after minification.
Tree Shaking Techniques with Terser
Tree shaking is a method of optimizing code by removing unused exports from the final bundle. Terser works well with tree shaking, especially when used in a Webpack production build.
To enable tree shaking, ensure that your project uses ES6 module syntax (import/export) and that the mode
is set to 'production'
. Webpack automatically performs tree shaking in production mode.
Here is how to make sure your Webpack configuration is set up correctly:
// webpack.config.jsmodule.exports = { mode: 'production', optimization: { usedExports: true, // Enables tree shaking minimize: true, minimizer: [new TerserPlugin()], },};
With this setup, Webpack will analyze the dependency graph of your modules and eliminate any code that is not referenced in the final build.
Code Splitting Strategies for Bundle Size Reduction
Code splitting is a technique that allows you to split your code into smaller chunks, which can be loaded on demand. This can significantly reduce the initial loading time of your application.
Webpack provides several ways to implement code splitting:
1. Entry Points: You can define multiple entry points in your Webpack configuration.
// webpack.config.js module.exports = { entry: { app: './src/app.js', vendor: './src/vendor.js', }, // other configurations... };
2. Dynamic Imports: Use import()
syntax to load modules asynchronously.
// example.js import('./moduleA').then(module => { // Use moduleA });
3. SplitChunksPlugin: Webpack includes a built-in plugin for code splitting that can be configured as follows:
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', // Split all chunks }, }, };
These strategies combined with Terser can lead to significant reductions in bundle size, as only the necessary code is loaded initially.
Reducing Bundle Size Using Terser
To effectively reduce bundle size with Terser, consider the following strategies:
1. Minification: Ensure that the Terser plugin is properly configured to minify your JavaScript code.
2. Dead Code Elimination: Use tree shaking to remove unused code. This is automatically handled in production mode with Webpack.
3. Code Splitting: Implement code splitting to load only the necessary code for the initial load.
4. Remove Console Logs: Configure Terser to drop console statements using the drop_console
option in the compress
settings.
Example of a configuration that combines these strategies:
// webpack.config.jsconst TerserPlugin = require('terser-webpack-plugin');module.exports = { mode: 'production', optimization: { minimize: true, minimizer: [ new TerserPlugin({ terserOptions: { compress: { drop_console: true, }, output: { comments: false, }, }, }), ], },};
Related Article: How to Use the Webpack CLI Option -d
Gzip Compression and Terser
Gzip compression is a method of compressing files for faster transfer over the network. While Terser reduces the size of JavaScript files, Gzip can further minimize the size of the resulting files when they are served.
To enable Gzip compression for your Webpack build, you can use the compression-webpack-plugin
. Install it via npm:
npm install compression-webpack-plugin --save-dev
Next, configure it in your Webpack setup:
// webpack.config.jsconst CompressionPlugin = require('compression-webpack-plugin');module.exports = { plugins: [ new CompressionPlugin({ algorithm: 'gzip', test: /\.js$/, // Only compress JavaScript files threshold: 10240, // Only compress files larger than 10KB minRatio: 0.8, // Compress files smaller than 80% of original size }), ],};
With this setup, your JavaScript files will be Gzip compressed, further reducing their size for network transfer.
Comparison of Terser and UglifyJS
Terser and UglifyJS are both popular tools for minifying JavaScript, but there are key differences between them:
1. Support for Modern JavaScript: Terser supports ES6+ syntax, whereas UglifyJS does not support modern JavaScript features. This makes Terser a better choice for projects using the latest JavaScript standards.
2. Performance: Terser is known to be faster and more efficient than UglifyJS, especially for larger codebases.
3. Active Development: Terser is actively maintained, ensuring it receives updates and improvements regularly, while UglifyJS has seen less frequent updates.
4. Customization: Terser provides more options for customization compared to UglifyJS, allowing for finer control over the minification process.
ES6 Support in Terser
Terser fully supports ES6 and newer JavaScript syntax. This is important for developers who want to leverage modern features such as arrow functions, async/await, and destructuring. Terser can minify these features without breaking the code, making it a suitable choice for contemporary JavaScript applications.
When using Terser, simply ensure that your project is set up to use ES6 modules. The minification process will handle the syntax appropriately.
Common Issues with Terser Webpack Plugin
Some common issues developers may encounter when using the Terser Webpack Plugin include:
1. Source Maps Not Working: Ensure that the sourceMap
option is set to true
both in Terser and in your Webpack configuration. Check that your development tools are configured to read the source maps.
2. Code Not Running After Minification: This can occur if there are issues with the code itself, such as using global variables or relying on non-standard syntax. Review your code for compatibility with ES6 and ensure there are no undefined variables.
3. Long Build Times: Enabling parallel processing in the Terser configuration can help speed up the build process. Make sure to set the parallel
option to true
.
Related Article: How to Configure Webpack for Expo Projects
Performance Enhancement with Terser
Terser can significantly enhance the performance of web applications by reducing JavaScript file sizes and optimizing code. The following approaches can be taken to maximize performance:
1. Always Use Production Mode: Ensure that Webpack is set to production mode for optimal builds.
2. Leverage Code Splitting and Tree Shaking: Use these techniques to reduce the amount of code loaded initially.
3. Configure Terser Options Wisely: Take advantage of Terser’s options to tailor the minification process to your specific needs.
4. Monitor Bundle Size: Regularly check the size of your bundles using tools like Webpack Bundle Analyzer to identify areas for improvement.
Additional Resources
- Terser Webpack Plugin Documentation