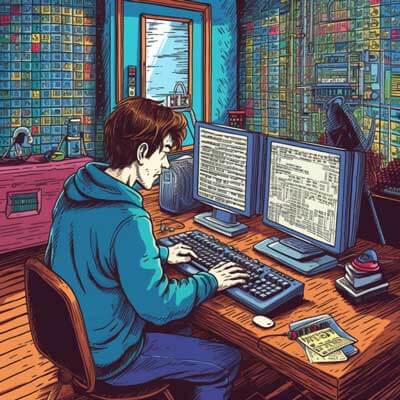
Table of Contents
Overview of Development Mode in the CLI Option -d
The -d option, when invoked, sets the mode to "development." This configuration adjusts several defaults in webpack to be more user-friendly for developers. It enables features like more verbose error messages, less aggressive optimizations, and source maps. The focus is on providing a smoother workflow for coding and testing rather than on optimizing the output for production.
Related Article: How to Set Webpack Target to Node.js
How to Enable Development Mode with the -d Flag
Enabling development mode through the CLI is simple. Just run the following command in your terminal:
webpack -d
This command tells webpack to compile your project with the development settings. It’s important to ensure that you have webpack installed globally or in your project’s dependencies to execute this command successfully.
Benefits of Using the -d Option for Development
The benefits of using the -d option are numerous. First, it speeds up the build process since webpack skips certain optimizations that are only necessary for production. Second, it generates source maps that help in debugging by mapping your minified code back to the original source files. Lastly, enabling this option allows for better error messages that provide more context, which is crucial while developing applications.
Impact of the -d Flag on the Build Process
When the -d flag is set, webpack modifies its build process in several ways. It skips optimizations such as minification and dead code elimination. This results in faster builds, which is essential for rapid development cycles. The lack of these optimizations means that the output files are larger but are easier to read and troubleshoot.
Related Article: How to Use Django Webpack Loader with Webpack
Using the -d Option with Other Commands
The -d option can be combined with other webpack commands to enhance its functionality. For example, you can use the -d option alongside the --watch flag to continuously monitor your files for changes while building in development mode:
webpack -d --watch
This command will keep webpack running and recompile whenever a file changes, allowing for a more dynamic development experience.
Configurations Applied When Using the -d Option
Several configurations are automatically applied when the -d flag is used. These include:
- Setting mode: 'development'
in the webpack configuration.
- Enabling detailed warnings and errors during the build process.
- Configuring the output to include source maps by default.
These defaults help streamline the development workflow, making it easier to identify and fix issues as they arise.
Comparison of -d and --mode development
While both the -d flag and the --mode development option accomplish the same goal of enabling development mode, they differ in their usage. The -d flag is a shorthand for the longer --mode development option. Both achieve the same outcome, but using -d is quicker and easier for those familiar with command-line tools.
webpack --mode development
This command is equivalent to:
webpack -d
Both will result in the same development mode settings.
Performance Effects
Using the -d option results in faster build times compared to production mode. This is due to fewer optimizations being performed. However, the trade-off is that the output files are larger and not optimized for performance. For most development processes, the speed of builds is prioritized over the size of the files, making -d a preferred choice.
Related Article: How to Configure Electron with Webpack
Common Errors Encountered with the -d Flag
Several common errors may arise when using the -d option. One frequent issue is forgetting to install webpack, which will result in a "command not found" error. Another common problem is misconfiguration in the webpack.config.js file, leading to build failures. It’s important to ensure that the configuration file is correctly set up to work with the development mode.
Disabling the -d Option in Development Mode
If you need to disable the -d option while still in development mode, simply specify the mode directly in your configuration file or use the --mode flag to set it to production:
webpack --mode production
This will switch the build process back to production optimizations, which include minification and other performance enhancements.
Webpack Configuration for the -d Option
In the webpack configuration file, you can specify the mode directly. If you use the -d flag, webpack will automatically set the mode to development. However, you can also set it manually in your webpack.config.js like this:
module.exports = { mode: 'development', // other configurations };
This allows for more flexibility if you want to use different modes based on conditions within your configuration.
Source Maps and the -d Flag
Source maps are a crucial feature in development mode. When the -d option is used, webpack generates source maps automatically. This allows developers to debug their applications more easily by tracing errors back to their original source code instead of the bundled output. This can be particularly useful in large applications where tracking down the source of an error can be challenging.
Related Article: How to Use Environment Variables in Webpack
Hot Module Replacement with the -d Option
Hot Module Replacement (HMR) is a feature that allows developers to update their code in real-time without a full reload. Using the -d option in conjunction with HMR can significantly enhance the development experience. To enable HMR, you can add the following configuration in your webpack.config.js:
const webpack = require('webpack'); module.exports = { // your other configurations plugins: [ new webpack.HotModuleReplacementPlugin() ], devServer: { hot: true, }, };
This setup allows for modules to be replaced without losing the application state, making it easier to see changes live.
Build Optimization Techniques Using -d
While the -d option is not about optimizing for production, there are still several optimization techniques that can be applied during development. For example, you might want to use dynamic imports to reduce initial load times or implement code splitting. Here's a simple example of how to implement code splitting:
import(/* webpackChunkName: "myChunk" */ './myModule').then(module => { // Use the module });
This approach helps keep the initial bundle size smaller, leading to faster loading times during development.
Performance Tuning in Development Mode
Although the -d option is not designed for performance optimizations, some tuning can be achieved. You can adjust the development server settings in your webpack.config.js to improve performance. For instance:
devServer: { contentBase: './dist', compress: true, port: 9000, hot: true, },
This configuration will enable compression and set the port for the development server, which can enhance the overall experience.
File Watching Features
The -d option works seamlessly with webpack's file watching capabilities. When running webpack with the -d flag, it can automatically rebuild the project when files change. By using the --watch flag in conjunction, you can set up a development environment that continuously updates as changes are made.
webpack -d --watch
This command will keep webpack monitoring your files for any updates, ensuring that you always have the latest version of your application running.
Related Article: How to Bypass a Router with Webpack Proxy
Module Bundling Practices
When using the -d option, module bundling practices may vary. Developers should focus on ensuring that their modules are correctly structured for easy debugging. Using named exports rather than default exports can improve clarity when reviewing the output bundles. For example:
// myModule.js export const myFunction = () => { /* ... */ }; export const myVariable = 42;
This approach helps maintain a clear relationship between the source code and the bundled output.
Plugins
Plugins are an essential part of any webpack configuration. When using the -d option, several plugins can enhance the development process. For instance, using the HtmlWebpackPlugin can streamline the creation of HTML files for your application:
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html' }), ], };
This setup automatically generates an HTML file that includes your bundled JavaScript, making the development workflow smoother.
Setting the Output Directory When Using -d
Setting an output directory is crucial for organizing your build files. When using the -d option, you can specify the output directory in your webpack.config.js like this:
module.exports = { output: { filename: 'bundle.js', path: __dirname + '/dist', }, };
This configuration will ensure that your bundled files are placed in the specified "dist" directory, keeping your project organized.
Additional Resources
- Webpack CLI Documentation