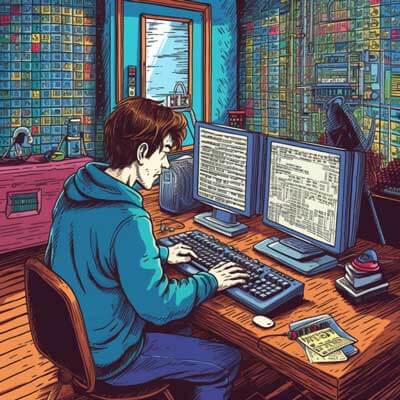
Table of Contents
Understanding the 'this' keyword in jQuery
The 'this' keyword in jQuery is a useful tool that allows you to refer to the current element or elements in a selected set. It provides a way to interact with and manipulate the selected elements in a more dynamic and flexible manner. In this answer, we will explore how to use the 'this' keyword effectively in jQuery.
Related Article: How to Use Async Await with a Foreach Loop in JavaScript
1. Using 'this' in event handlers
When working with event handlers in jQuery, the 'this' keyword refers to the DOM element that triggered the event. It allows you to access and manipulate the properties and attributes of the specific element that triggered the event.
For example, let's say you have a list of buttons and you want to change the background color of the button that is clicked:
$('button').click(function() { $(this).css('background-color', 'red'); });
In the above example, when a button is clicked, the 'this' keyword refers to the specific button element that triggered the click event. By using $(this), we can modify the CSS properties of the clicked button.
2. Using 'this' in each() function
The 'this' keyword can also be used inside the jQuery each() function to iterate over a collection of elements and perform operations on each individual element.
For example, let's say you have a list of paragraphs and you want to add a class to each paragraph:
$('p').each(function() { $(this).addClass('highlight'); });
In the above example, the 'this' keyword inside the each() function refers to each individual paragraph element. By using $(this), we can add the 'highlight' class to each paragraph.
Best practices and considerations
- Remember that the value of 'this' can change depending on the context in which it is used. It is important to understand the scope and context in which you are using 'this' to avoid any unexpected behavior.
- When using 'this' in nested functions or callbacks, it is a good practice to store the reference of 'this' in a variable to avoid any confusion or conflicts with the outer scope. For example:
var self = this; $('button').click(function() { // 'this' refers to the button element // 'self' refers to the outer scope });
- It is recommended to use arrow functions instead of traditional function expressions when working with 'this' inside event handlers or callbacks. Arrow functions do not have their own 'this' value, so they inherit the 'this' value from the surrounding context.
$('button').click(() => { // 'this' refers to the element that triggered the click event });
- If you need to pass the value of 'this' to another function, you can use the jQuery proxy() method or the bind() method to preserve the context of 'this'.
$('button').click(function() { anotherFunction.call(this); });
In the above example, the call() method is used to invoke the anotherFunction with the context of 'this'.
Related Article: How To Check Checkbox Status In jQuery
Alternative ideas
While the 'this' keyword is a common and widely used feature in jQuery, there are alternative ways to achieve similar functionality in certain scenarios:
- Using the event.target property: In event handlers, you can access the element that triggered the event using the event.target property. This allows you to directly manipulate the element without relying on the 'this' keyword.
$('button').click(function(event) { $(event.target).css('background-color', 'red'); });
- Using the jQuery selector: Instead of relying on the 'this' keyword, you can use the jQuery selector to explicitly select and manipulate the desired elements.
$('button').click(function() { $('button').css('background-color', 'red'); });
While these alternatives can be useful in specific cases, the 'this' keyword remains a useful and commonly used feature in jQuery for working with the current element or elements in a selected set.