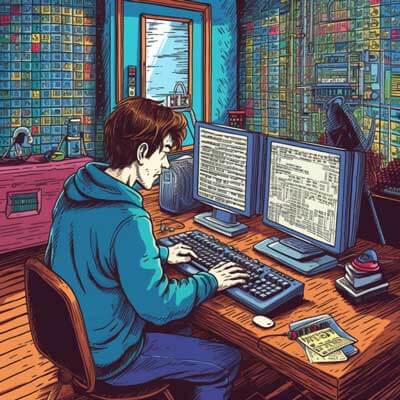
Table of Contents
Overview of Tough-Cookie
Tough-Cookie is a library for managing HTTP cookies in Node.js applications. It provides a robust way to create, read, and manipulate cookies, making it easier to work with user sessions and other cookie-based data. Cookies are small pieces of data that servers send to the client, which are then stored on the user's computer. These cookies can hold various types of information, such as user preferences, session IDs, and authentication tokens. Tough-Cookie abstracts many of the complexities associated with cookie management, making it simpler for developers to implement cookie handling within their applications.
Related Article: How to use a Next.js performance analyzer library
What is Tough-Cookie npm?
Tough-Cookie is available as an npm package, which means it can be easily installed and integrated into any Node.js project. The npm package allows developers to manage cookies seamlessly without worrying about the underlying details of cookie storage and retrieval. Using Tough-Cookie, developers can create cookie jars that serve as containers for cookies, handle various cookie attributes, and maintain the state of cookies across requests. This npm package is particularly useful for applications that require user authentication, as cookies are a common way to manage user sessions.
Setting Up Tough-Cookie in Node.js
To get started with Tough-Cookie, it is necessary to install the package via npm. This can be accomplished using the following command in your terminal:
npm install tough-cookie
Once installed, Tough-Cookie can be imported into your Node.js application. The following code snippet demonstrates how to do this:
// app.jsconst { CookieJar } = require('tough-cookie');// Create a new cookie jarconst jar = new CookieJar();
With the CookieJar instance created, developers can now manage cookies effectively. The next step involves managing cookie jars, which allows for the storage and retrieval of cookies for different domains and paths.
Managing Cookie Jars
Cookie jars serve as a container for cookies, allowing developers to organize and manage them easily. A cookie jar can store multiple cookies, each associated with specific domain names and paths. To add cookies to a jar, the setCookie
method can be used. The following example illustrates how to add a cookie to the jar:
// Set a cookie in the jarjar.setCookie('myCookie=myValue; Domain=mydomain.com; Path=/', (err) => { if (err) { console.error('Error setting cookie:', err); } else { console.log('Cookie set successfully'); }});
In this example, a cookie named myCookie
is set with the value myValue
. The cookie is associated with the domain mydomain.com
and has a path of /
, meaning it will be sent with requests to any URL under this domain.
Related Article: How to Fix npm Unsupported Engine Issues
Cookie Parsing with Tough-Cookie
Tough-Cookie provides functionality to parse cookie strings into manageable objects. This is helpful when dealing with cookies received from HTTP responses. The Cookie.parse
method can be used to convert a cookie string into a cookie object, as shown in the following example:
// Parse a cookie stringconst parsedCookie = Cookie.parse('myCookie=myValue; Path=/; Domain=mydomain.com');// Log the parsed cookieconsole.log(parsedCookie);
The output will provide a structured representation of the cookie, including its name, value, and attributes. This allows developers to inspect and manipulate cookie data easily.
Cookie Serialization Techniques
Serialization refers to the process of converting an object into a string format that can be easily stored or transmitted. Tough-Cookie offers methods for serializing cookies back into a string format. This is useful when sending cookies in HTTP headers. The Cookie.toString()
method can be utilized as follows:
// Create a cookie objectconst cookie = new Cookie('myCookie', 'myValue', { domain: 'mydomain.com', path: '/',});// Serialize the cookieconst cookieString = cookie.toString();console.log(cookieString);
The output will be a formatted string that can be sent with HTTP requests. Understanding serialization is crucial for effective cookie management, as it ensures cookies are sent correctly between clients and servers.
Handling HTTP Cookies
HTTP cookies are crucial for maintaining state between the client and server. Tough-Cookie simplifies the process of handling cookies in HTTP requests and responses. When making an HTTP request, cookies associated with the target domain can be automatically retrieved from the cookie jar. For example, using the request library in conjunction with Tough-Cookie can streamline cookie handling:
const request = require('request');// Configure request optionsconst options = { url: 'http://mydomain.com/api', jar: jar, // Use the cookie jar};// Make a requestrequest(options, (error, response, body) => { if (error) { console.error('Request failed:', error); } else { console.log('Response:', body); }});
In this code, the cookie jar is passed to the request options. This ensures that cookies stored in the jar are included automatically in the HTTP request. Similarly, responses can also update the cookie jar with new cookies from the server.
Cookie Attributes Explained
Cookies possess various attributes that determine their behavior. Some common attributes include:
- Domain: Specifies the domain that can access the cookie. This prevents other domains from accessing the cookie's value.
- Path: Defines the URL path that must exist for the cookie to be sent.
- Expires/Max-Age: Indicates when the cookie should expire. If not set, the cookie will be deleted when the session ends.
- Secure: Ensures that the cookie is only sent over HTTPS connections.
- HttpOnly: Prevents client-side scripts from accessing the cookie, adding a layer of security.
Developers can set these attributes when creating cookies with Tough-Cookie. For example:
const cookie = new Cookie('myCookie', 'myValue', { domain: 'mydomain.com', path: '/', secure: true, httpOnly: true, expires: new Date(Date.now() + 3600 * 1000), // Expires in 1 hour});
These attributes play a critical role in cookie security and management, ensuring that cookies are only accessible when appropriate.
Related Article: How to Fix npm is Not Recognized as an Internal Command
Secure Cookies in Tough-Cookie
Secure cookies are an important aspect of web security. By using the secure
attribute, developers can ensure that cookies are only transmitted over secure HTTPS connections. This prevents potential attackers from intercepting cookies over insecure connections. Here’s how to set a secure cookie:
const secureCookie = new Cookie('secureCookie', 'secureValue', { domain: 'mydomain.com', path: '/', secure: true, // Only sent over HTTPS});
Same-Site Cookie Settings
Same-Site cookie settings help mitigate the risk of cross-origin request forgery attacks. This attribute can take three values: Strict
, Lax
, and None
.
- Strict: The cookie will only be sent in a first-party context (i.e., the same site).
- Lax: The cookie is sent with top-level navigations and will be sent along with GET requests initiated by third-party websites.
- None: The cookie is sent in all contexts, including cross-origin requests, but the Secure
attribute must also be set.
To set the Same-Site attribute using Tough-Cookie, use the following code:
const sameSiteCookie = new Cookie('sameSiteCookie', 'value', { domain: 'mydomain.com', path: '/', sameSite: 'Lax', // Adjust according to needs});
Implementing Same-Site cookie settings is a practical method to enhance the security of web applications.
Retrieving Cookies from the Jar
Cookies stored in a cookie jar can be retrieved easily using the getCookieString
method. This method allows developers to obtain all cookies for a specific URL in a single call. Here’s an example:
// Retrieve cookies for a specific URLjar.getCookieString('http://mydomain.com', (err, cookies) => { if (err) { console.error('Error retrieving cookies:', err); } else { console.log('Cookies for mydomain.com:', cookies); }});
This code will return a string of cookie values associated with the specified URL, which can then be utilized in requests or for other purposes.
Clearing Cookies with Tough-Cookie
Clearing cookies is essential for managing user sessions and ensuring that outdated or unwanted cookies are removed. Tough-Cookie provides the removeCookie
method for this purpose. Here’s how to use it:
// Remove a cookie from the jarjar.removeCookie('mydomain.com', 'myCookie', (err) => { if (err) { console.error('Error removing cookie:', err); } else { console.log('Cookie removed successfully'); }});
Related Article: How to Use npm with Next.js
Comparing Tough-Cookie to Other Libraries
Tough-Cookie stands out among other cookie management libraries due to its extensive feature set and ease of use. Libraries such as cookie and cookies-js offer basic cookie handling capabilities, but they lack the advanced features provided by Tough-Cookie, such as cookie jars and extensive attribute handling.
For example, while cookie is primarily focused on parsing and serializing cookies, Tough-Cookie includes built-in mechanisms for managing cookies across multiple domains and paths, which is critical for complex applications.
Here’s a comparison of basic functionalities:
- Tough-Cookie: Manages cookie jars, supports Same-Site settings, and offers robust parsing/serialization.
- cookie: Focuses on parsing and serializing cookies without a cookie jar concept.
- cookies-js: Provides simple client-side cookie management but lacks server-side features.
In evaluating cookie management options, Tough-Cookie provides a comprehensive solution, making it a suitable choice for Node.js applications.
Working with Multiple Cookies
Handling multiple cookies simultaneously is a common requirement in web applications. Tough-Cookie allows developers to manage multiple cookies easily within a single jar. Cookies can be added, retrieved, and removed independently, providing flexibility in managing user sessions.
To set multiple cookies, developers can use the setCookie
method multiple times:
// Add multiple cookies to the jarjar.setCookie('cookie1=value1; Path=/; Domain=mydomain.com', (err) => { if (err) console.error(err);});jar.setCookie('cookie2=value2; Path=/; Domain=mydomain.com', (err) => { if (err) console.error(err);});
Retrieving all cookies at once can be done using the getCookieString
method, as shown previously. This approach simplifies cookie management, especially in applications that rely on multiple cookies for user authentication and other features.
Setting Cookie Options
When creating cookies, developers can specify various options to customize their behavior. Options include domain, path, expiry, secure, httpOnly, and Same-Site attributes. Setting these options correctly is crucial for ensuring that cookies are sent and received as intended.
Here’s an example of setting various cookie options:
const customCookie = new Cookie('customCookie', 'customValue', { domain: 'mydomain.com', path: '/', expires: new Date(Date.now() + 86400 * 1000), // Expires in 1 day secure: true, httpOnly: true, sameSite: 'Strict',});
This code snippet demonstrates how to create a cookie with multiple attributes, enhancing security and controlling the cookie’s accessibility. Properly setting cookie options is vital for maintaining security and functionality in web applications.