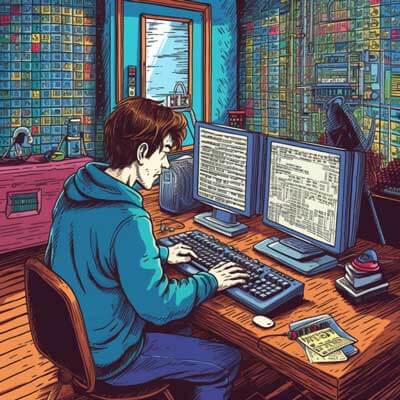
Table of Contents
React Router is a popular library in the React ecosystem that provides routing capabilities for single-page applications. One of the key features of React Router is the useHistory hook, which allows you to programmatically navigate your application to different routes. In this guide, we will explore how to use useHistory from React Router Dom correctly.
Step 1: Import the useHistory hook
To use useHistory, you need to import it from the 'react-router-dom' package. You can do this by adding the following line of code at the top of your file:
import { useHistory } from 'react-router-dom';
Related Article: How to Use Regex for Password Validation in Javascript
Step 2: Access the history object
Once you have imported useHistory, you can start using it by calling it inside your functional component. The useHistory hook returns a history object that you can use to navigate your application programmatically. Here is an example of how to access the history object:
const MyComponent = () => { const history = useHistory(); // Use the history object to navigate const navigateToHome = () => { history.push('/'); }; return ( <div> <button onClick={navigateToHome}>Go to Home</button> </div> ); };
In the example above, we define a functional component called MyComponent. Inside the component, we call the useHistory hook to get access to the history object. We then define a function called navigateToHome that uses the push method of the history object to navigate to the '/' route when a button is clicked.
Step 3: Programmatically navigate to a different route
Once you have access to the history object, you can use its methods to navigate to different routes in your application. The most commonly used method is push, which allows you to navigate to a new route. Here is an example of how to use the push method:
const MyComponent = () => { const history = useHistory(); const navigateToAbout = () => { history.push('/about'); }; return ( <div> <button onClick={navigateToAbout}>Go to About</button> </div> ); };
In the example above, we define a function called navigateToAbout that uses the push method to navigate to the '/about' route when a button is clicked.
Step 4: Go back to the previous route
In addition to navigating to a new route, you can also go back to the previous route using the goBack method of the history object. Here is an example of how to use goBack:
const MyComponent = () => { const history = useHistory(); const goBack = () => { history.goBack(); }; return ( <div> <button onClick={goBack}>Go Back</button> </div> ); };
In the example above, we define a function called goBack that uses the goBack method to navigate back to the previous route when a button is clicked.
Related Article: JavaScript HashMap: A Complete Guide
Step 5: Pass state to a new route
The push method of the history object also allows you to pass state to the new route. This can be useful when you want to pass data between routes. Here is an example of how to pass state using useHistory:
const MyComponent = () => { const history = useHistory(); const navigateToProduct = () => { history.push('/product', { productId: 123 }); }; return ( <div> <button onClick={navigateToProduct}>Go to Product</button> </div> ); };
In the example above, we define a function called navigateToProduct that uses the push method to navigate to the '/product' route and pass an object with the productId property as state.
To access the state in the new route, you can use the useLocation hook from React Router Dom. Here is an example of how to access the state in the '/product' route:
import { useLocation } from 'react-router-dom'; const Product = () => { const location = useLocation(); const { productId } = location.state; return ( <div> <h1>Product Details</h1> <p>Product ID: {productId}</p> </div> ); };
In the example above, we import the useLocation hook from React Router Dom and use it to access the location object. We then destructure the productId from the state object and display it on the page.
Step 6: Redirect to a different route
In some cases, you may want to redirect the user to a different route based on certain conditions. You can achieve this by using the Redirect component from React Router Dom along with the useHistory hook. Here is an example:
import { useHistory, Redirect } from 'react-router-dom'; const MyComponent = () => { const history = useHistory(); const shouldRedirect = true; if (shouldRedirect) { return <Redirect to="/new-route" />; } // Rest of the component code };
In the example above, we import the Redirect component from React Router Dom and use it inside the MyComponent component. If the shouldRedirect variable is true, the user will be redirected to the '/new-route' route.
Step 7: Use useHistory in a class component
If you are using class components instead of functional components, you can still use useHistory by wrapping your component with the withRouter higher-order component from React Router Dom. Here is an example:
import React from 'react'; import { withRouter } from 'react-router-dom'; class MyComponent extends React.Component { navigateToHome = () => { this.props.history.push('/'); }; render() { return ( <div> <button onClick={this.navigateToHome}>Go to Home</button> </div> ); } } export default withRouter(MyComponent);
In the example above, we import the withRouter higher-order component from React Router Dom and wrap the MyComponent class with it. This allows us to access the history object through the props.