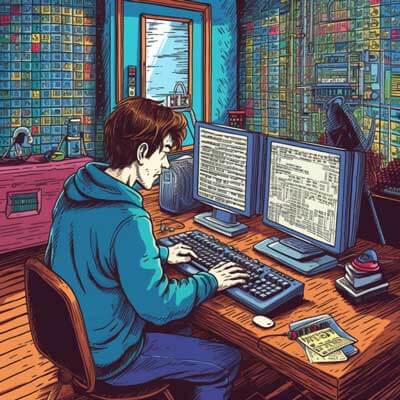
Table of Contents
Overview of Asset Management with CopyPlugin
Asset management is crucial in modern web development. CopyPlugin serves as a tool within the Webpack ecosystem to handle the copying of files and directories. This includes images, fonts, and other static assets that are essential for a web application but are not processed by Webpack. Copying these assets ensures that they are available in the output directory during the build process, allowing developers to maintain a clean and organized project structure.
Related Article: How to Configure SVGR with Webpack
Installation of CopyPlugin
To get started with CopyPlugin, it needs to be installed as a development dependency in a Webpack project. This can be done using npm or yarn. The commands are as follows:
npm install copy-webpack-plugin --save-dev
or
yarn add copy-webpack-plugin --dev
After installation, it is necessary to require the plugin in the Webpack configuration file.
// webpack.config.jsconst CopyPlugin = require('copy-webpack-plugin');
Key Features of CopyPlugin
CopyPlugin offers several features that enhance asset management:
1. Simple File Copying: Easily copy files from one location to another.
2. Directory Copying: Copy entire directories, maintaining the directory structure.
3. File Filtering: Include or exclude specific files using glob patterns.
4. Custom Output Paths: Specify where to place copied files in the output directory.
5. Conditional Copying: Copy files based on certain conditions.
These features allow for a flexible asset management strategy tailored to project needs.
Configuration of CopyPlugin for Projects
Setting up CopyPlugin requires adding it to the Webpack configuration file. The following example demonstrates a basic configuration that copies assets from a src/assets
directory to the dist/assets
output directory.
// webpack.config.jsconst path = require('path');const CopyPlugin = require('copy-webpack-plugin');module.exports = { // Other configurations plugins: [ new CopyPlugin({ patterns: [ { from: 'src/assets', to: 'dist/assets' }, ], }), ],};
This simple setup copies all files from src/assets
to dist/assets
during the build process.
Related Article: How to Configure Webpack for Expo Projects
Setting Up Output Directory for Assets
The output directory is defined in the Webpack configuration. By default, assets are copied to the dist
folder, but this can be customized. Below is how to set the output directory:
// webpack.config.jsmodule.exports = { output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, plugins: [ new CopyPlugin({ patterns: [ { from: 'src/assets', to: 'dist/assets' }, ], }), ],};
The path
option in the output
object specifies the directory structure for the output files.
Managing Static Assets with CopyPlugin
Static assets like images and fonts need to be managed effectively. CopyPlugin allows developers to ensure these files are readily available in the final build. For example, if there are various image files in src/images
, use the following configuration to manage them:
// webpack.config.jsnew CopyPlugin({ patterns: [ { from: 'src/images', to: 'dist/images' }, ],}),
This setup maintains the directory structure of images, making them accessible in the output build.
Integrating CopyPlugin with Other Tools
Integrating CopyPlugin with other tools in the Webpack ecosystem enhances the development experience. For instance, combining it with HtmlWebpackPlugin allows for dynamic HTML files that reference the copied assets. Below is an example:
const HtmlWebpackPlugin = require('html-webpack-plugin');module.exports = { plugins: [ new HtmlWebpackPlugin({ template: 'src/index.html', }), new CopyPlugin({ patterns: [ { from: 'src/assets', to: 'dist/assets' }, ], }), ],};
This integration ensures the HTML file generated includes links to the copied assets.
Types of Files Supported by CopyPlugin
CopyPlugin supports a wide range of file types, making it versatile for various projects. Common formats include:
- Images (JPEG, PNG, GIF, SVG)
- Fonts (TTF, WOFF, WOFF2)
- JSON files
- Text files (TXT)
This flexibility allows developers to handle different asset types according to project requirements.
Related Article: How to Use ESLint Webpack Plugin for Code Quality
Excluding Files from the Copy Process
Excluding specific files from being copied can be achieved with the help of glob patterns. For instance, if you want to copy all files except those with the .txt
extension, the configuration would look like this:
new CopyPlugin({ patterns: [ { from: 'src/assets', to: 'dist/assets', globOptions: { ignore: ['*.txt'], }, }, ],}),
This configuration ensures that only the desired files are included in the final build.
Compatibility of CopyPlugin with Webpack 5
CopyPlugin is compatible with Webpack 5, ensuring that developers can take advantage of the latest features and improvements. Using the plugin with Webpack 5 is straightforward, as shown in the previous examples. The newer version of Webpack allows for an enhanced build process, and CopyPlugin is designed to work seamlessly with it.
Specifying Output Paths for Copied Files
Customizing the output paths for copied files can be done within the patterns
array. For example, if you want to copy an image to a different folder structure, you can specify the to
property accordingly:
new CopyPlugin({ patterns: [ { from: 'src/images/logo.png', to: 'dist/assets/images/logo.png' }, ],}),
This setup allows for detailed control over where files are placed in the final build.
Conditional File Copying with CopyPlugin
Conditional file copying is useful when certain files should only be copied under specific conditions. This can be achieved with custom logic in the configuration. For instance:
const isProduction = process.env.NODE_ENV === 'production';new CopyPlugin({ patterns: isProduction ? [{ from: 'src/prod-assets', to: 'dist/assets' }] : [{ from: 'src/dev-assets', to: 'dist/assets' }],}),
In this example, the assets copied depend on whether the build is for production or development.
Related Article: How to Use Webpack Manifest Plugin
Common Use Cases for CopyPlugin in Development Workflow
CopyPlugin is commonly used in various scenarios, such as:
- Copying Static Assets: Copy images, fonts, and other files that do not require processing.
- Managing Versioned Assets: Copy files with versioning in their names for cache management.
- Setting up Example Projects: Copy example files that developers can use to get started quickly.
These use cases illustrate the flexibility and necessity of asset management in web development.
Enhancing the Build Process with CopyPlugin
Incorporating CopyPlugin into the build process enhances the overall workflow. It ensures that all necessary assets are included and organized, reducing manual management. The automation of asset copying minimizes errors and saves time, allowing developers to focus on core functionality.
File Watching Mechanism in CopyPlugin
CopyPlugin includes a file watching mechanism that automatically copies files when changes occur. This is particularly useful during development, as it enhances the live reloading experience. The following configuration enables watching:
new CopyPlugin({ patterns: [ { from: 'src/assets', to: 'dist/assets' }, ], options: { watch: true, },}),
With this setup, any changes in the src/assets
directory will trigger a copy to the dist/assets
directory on the fly.
Dependency Management Considerations with CopyPlugin
Managing dependencies is essential for a smooth build process. When using CopyPlugin, it is important to ensure that all necessary files are included and that any dependencies are resolved. This might involve:
- Keeping track of file paths.
- Ensuring that any dynamic imports are also considered.
- Regularly updating the plugin to maintain compatibility with the latest Webpack features.
Related Article: How to Use Webpack in Your Projects
Additional Resources
- Installing webpack copy plugin