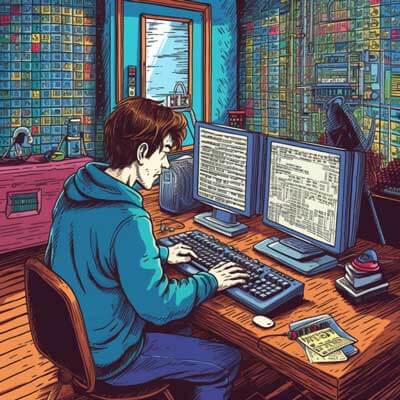
Table of Contents
Overview of Using -d for Development Mode
The -d
flag in webpack is shorthand for --debug
, which enables development mode. This mode optimizes the build process for debugging instead of performance. It can simplify the development cycle by providing helpful features such as detailed error messages, source maps, and more manageable bundle sizes. The primary aim is to give developers quick feedback while they work on their applications.
To start webpack in development mode, the command looks like this:
webpack -d
This command compiles the application while enabling debugging features.
Related Article: How to Fix Webpack Command Not Found Error
Setting Up a Development Server
A development server allows for live reloading, which means when files are changed, the server automatically updates the browser. To set up a development server, install webpack-dev-server
:
npm install --save-dev webpack-dev-server
Next, add a script to your package.json
:
"scripts": { "start": "webpack-dev-server --open"}
Configuring Entry Points
Entry points define where webpack starts the bundling process. You can specify a single entry point or multiple ones. For a single entry point, your webpack.config.js
might look like this:
module.exports = { entry: './src/index.js', // other configurations...};
If your application has multiple entry points, the configuration can be:
module.exports = { entry: { app: './src/index.js', admin: './src/admin.js' }, // other configurations...};
In both cases, webpack will begin its bundling from these files.
Defining Output Bundles
Output bundles are the final product created by webpack. You define where and how these bundles are named in the output
section of your configuration:
module.exports = { output: { filename: 'bundle.js', path: __dirname + '/dist' }, // other configurations...};
Here, the output file bundle.js
will be created in the dist
directory. If you have multiple entry points, you can use:
module.exports = { output: { filename: '[name].bundle.js', path: __dirname + '/dist' }, // other configurations...};
With [name]
, webpack substitutes the name of each entry point.
Related Article: How to Fix webpack Build Cannot Find Name ‘vi’ Vitest
Using Loaders for Asset Management
Loaders allow webpack to process different types of files. For instance, if you want to process CSS files, you can use css-loader
and style-loader
. Install these loaders:
npm install --save-dev style-loader css-loader
Then, configure them in webpack.config.js
:
module.exports = { module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'] } ] }, // other configurations...};
With this setup, any CSS file imported into your JavaScript will be processed correctly.
Implementing Plugins for Enhanced Functionality
Plugins extend webpack's capabilities and can be used for various tasks such as optimizing bundles or defining environment variables. For example, to use the HtmlWebpackPlugin
, install it first:
npm install --save-dev html-webpack-plugin
Then, configure it in your webpack.config.js
:
const HtmlWebpackPlugin = require('html-webpack-plugin');module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', filename: 'index.html' }) ], // other configurations...};
This plugin will generate an HTML file that includes your bundled JavaScript.
Enabling Hot Module Replacement
Hot Module Replacement (HMR) allows you to replace modules without a full browser refresh. This can greatly speed up development. To enable HMR, update your webpack.config.js
:
const webpack = require('webpack');module.exports = { devServer: { hot: true }, plugins: [ new webpack.HotModuleReplacementPlugin() ], // other configurations...};
When you run the development server, any changes made will automatically reflect in the browser.
Integrating Babel for JavaScript Transpilation
Babel is a tool that allows you to use the latest JavaScript features without worrying about browser compatibility. To use Babel with webpack, first, install the necessary packages:
npm install --save-dev babel-loader @babel/core @babel/preset-env
Next, add Babel configuration to webpack.config.js
:
module.exports = { module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'] } } } ] }, // other configurations...};
This setup allows you to write modern JavaScript while Babel compiles it for older browsers.
Related Article: How to Use Django Webpack Loader with Webpack
Configuring Source Maps for Better Debugging
Source maps help in debugging by mapping your compiled code back to the original source code. To enable source maps in development mode, add the following to webpack.config.js
:
module.exports = { devtool: 'source-map', // other configurations...};
With this setting, you can see the original source files in your browser's developer tools, making it easier to trace errors.
Exploring Tree Shaking for Reducing Bundle Size
Tree shaking is a technique used to eliminate dead code from the final bundle. To enable tree shaking, ensure you are using ES6 module syntax (i.e., import
and export
). In your webpack.config.js
, set the mode to production:
module.exports = { mode: 'production', // other configurations...};
During the build process, webpack will automatically remove unused code, reducing the bundle size.
Implementing Code Splitting for Efficient Loading
Code splitting allows you to divide your code into smaller chunks that can be loaded on demand. This can improve the initial loading time of your application. To implement code splitting, use dynamic import()
syntax:
import('./module').then(module => { // Use the module here});
Webpack will automatically create separate bundles for each dynamically imported module.
Optimizing Bundles for Performance
Optimizing bundles can significantly improve the performance of your application. Some common techniques include minifying JavaScript and CSS files. To minify your output, you can use the TerserWebpackPlugin
for JavaScript:
npm install --save-dev terser-webpack-plugin
Then configure it in your webpack.config.js
:
const TerserPlugin = require('terser-webpack-plugin');module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, // other configurations...};
For CSS, use css-minimizer-webpack-plugin
:
npm install --save-dev css-minimizer-webpack-plugin
And configure it like this:
const CssMinimizerPlugin = require('css-minimizer-webpack-plugin');module.exports = { optimization: { minimizer: [ new TerserPlugin(), new CssMinimizerPlugin(), ], }, // other configurations...};
These optimizations will result in smaller bundle sizes and faster load times.
Related Article: How to Bypass a Router with Webpack Proxy
Distinguishing Development and Production Modes
Setting up different configurations for development and production is essential for efficient workflows. In webpack.config.js
, you can create separate configurations or use environment variables to toggle settings.
For example, you can use webpack-merge
to merge common configurations:
npm install --save-dev webpack-merge
Create a webpack.common.js
for shared settings:
module.exports = { // Common settings};
Then create webpack.dev.js
and webpack.prod.js
for development and production, respectively:
// webpack.dev.jsconst { merge } = require('webpack-merge');const common = require('./webpack.common');module.exports = merge(common, { mode: 'development', // Development specific settings});
// webpack.prod.jsconst { merge } = require('webpack-merge');const common = require('./webpack.common');module.exports = merge(common, { mode: 'production', // Production specific settings});
This structure allows for clean separation of configurations based on the environment.
Additional Resources
- Understanding Webpack's -d Flag