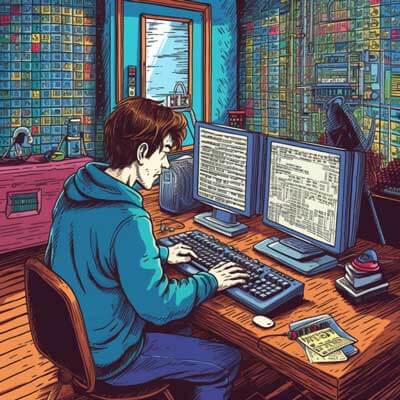
Table of Contents
Overview of Webpack
Webpack is a module bundler primarily used for JavaScript applications. It takes modules with dependencies, compiles them into a single file or a few files, and optimizes them for performance. This process allows developers to manage and serve their assets efficiently. Webpack can handle not just JavaScript, but also CSS, images, and other file types. It streamlines development by allowing developers to write modular code, which improves maintainability.
The core concept revolves around its ability to bundle assets. When you have a project with various files that depend on each other, Webpack can analyze these dependencies and create a dependency graph. This graph is essential for determining the order in which files should be loaded. When a project grows, managing dependencies manually becomes cumbersome. Webpack automates this process, ensuring that all necessary files are included in the final output.
Related Article: How to Configure Webpack for Expo Projects
Configuring Webpack
Configuring Webpack begins with creating a configuration file, typically named webpack.config.js
. This file is written in JavaScript and exports an object that defines the various options for Webpack. Basic configurations include specifying the entry point, output file, loaders, and plugins.
To set up a basic Webpack configuration, create a file called webpack.config.js
in your project root:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, };
This example sets the entry point to ./src/index.js
and specifies that the output will be a file named bundle.js
located in a dist
directory. The path
module is used to ensure that the output path is correctly resolved.
Entry Point Definition
The entry point is the starting point for Webpack to build its dependency graph. It tells Webpack which module to start with and follows the imports/exports to include all dependent modules. You can define multiple entry points if your application requires it.
For instance, you might have a scenario where you want separate bundles for different parts of your application. You can define multiple entry points like this:
// webpack.config.js module.exports = { entry: { app: './src/app.js', vendor: './src/vendor.js', }, output: { filename: '[name].bundle.js', path: path.resolve(__dirname, 'dist'), }, };
In this configuration, Webpack will create two separate bundles: app.bundle.js
for your application code and vendor.bundle.js
for third-party libraries.
Output Configuration
The output configuration specifies how and where Webpack will output the bundles it generates. Key properties include filename
, which determines the name of the output file, and path
, which specifies the directory where the output file will be created.
You can use placeholders in the filename to generate dynamic names. For example, using [name]
will replace it with the name of the entry point, while [contenthash]
helps in cache busting by generating a unique hash for the file based on its content.
Example output configuration:
// webpack.config.js module.exports = { output: { filename: '[name].[contenthash].js', path: path.resolve(__dirname, 'dist'), clean: true, // cleans the output directory before each build }, };
The clean
option ensures that old files in the output directory are removed before a new build, keeping the output directory tidy.
Related Article: How To Fix Unhandled Exception Cannot Find Module Webpack
Working with Loaders
Loaders in Webpack transform the files as they are added to the dependency graph. For instance, if you want to include CSS or images in your JavaScript files, you will use loaders. Loaders can process a variety of file types, making it possible to include many assets in your bundles.
To use loaders, first install them via npm. For example, to use the css-loader and style-loader, you can run:
npm install --save-dev css-loader style-loader
After installing, you can configure them in your Webpack file:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, };
In this setup, any files ending in .css
will be processed first by css-loader
, which interprets @import
and url()
like import/require()
and then by style-loader
, which injects the styles into the DOM. For more information on configuring loaders, check out our article on setting up SCSS loaders.
Using Plugins
Plugins extend Webpack's functionality beyond what loaders provide. They can perform a wide range of tasks such as optimizing the output, managing environment variables, and more.
To use a plugin, it must be installed and added to the plugins
array in the Webpack configuration. For example, to use the HtmlWebpackPlugin, which simplifies the creation of HTML files to serve your bundles, install it using npm:
npm install --save-dev html-webpack-plugin
Then configure it in your webpack.config.js
:
// webpack.config.js const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', filename: 'index.html', }), ], };
This setup tells Webpack to generate an HTML file based on the specified template and automatically include the bundled JavaScript files. For more advanced plugin usage, consider reading about using the Webpack Manifest Plugin.
Setting Up Dev Server
A development server provides a local server environment to serve your application, allowing for faster development. Webpack comes with a built-in dev server that can be easily configured.
To install the Webpack Dev Server, run:
npm install --save-dev webpack-dev-server
Next, modify your Webpack configuration to include the dev server settings:
// webpack.config.js module.exports = { devServer: { static: './dist', open: true, // opens the browser after the server starts }, };
You can then run the dev server using:
npx webpack serve
This command will start the Webpack dev server, serving your application at http://localhost:8080
by default, and will automatically open this URL in your default web browser. If you want to learn more about optimizing your development experience, check out our guide on configuring dev server proxy.
Enabling Hot Module Replacement
Hot Module Replacement (HMR) allows you to update modules in your application without a full page reload. This enhances the development experience by preserving the application state even when changes are made.
To enable HMR, you need to modify your Webpack Dev Server settings and add the HotModuleReplacementPlugin in your plugins:
// webpack.config.js const webpack = require('webpack'); module.exports = { devServer: { hot: true, // enable HMR }, plugins: [ new webpack.HotModuleReplacementPlugin(), ], };
With this configuration, when you make changes to your application code, only the modified modules will be updated in the browser, rather than refreshing the entire page.
Related Article: How to Use Extract Text Webpack Plugin
Implementing Code Splitting
Code splitting is a technique that allows you to split your code into multiple bundles, which can be loaded on demand. This is particularly useful for improving the initial load time of your application by only loading the necessary code upfront.
Webpack supports code splitting through dynamic imports and configuration options. To implement dynamic imports, you can use the import()
function:
// In your JavaScript file import(/* webpackChunkName: "my-chunk-name" */ './myModule.js') .then(module => { // Use the module here });
Alternatively, you can configure code splitting in your webpack.config.js
:
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', // split all types of chunks }, }, };
This configuration will split chunks automatically based on usage, ensuring that shared dependencies are not duplicated across bundles.
Tree Shaking Techniques
Tree shaking is a technique used to eliminate dead code from your final bundle. It relies on ES6 module syntax and helps reduce the size of your output files by removing unused exports.
To enable tree shaking, ensure that your project uses ES6 modules (i.e., import
and export
) and set the mode
to production
in your Webpack configuration:
// webpack.config.js module.exports = { mode: 'production', optimization: { usedExports: true, // enables tree shaking }, };
Optimization Strategies
Optimizing your Webpack configuration can greatly improve the performance of your application. There are several strategies to consider:
1. Minification: Use the Terser plugin to minify your JavaScript code. This reduces file size by eliminating whitespace and shortening variable names.
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
2. Caching: Use [contenthash]
in your output filenames to enable caching. This ensures that when files change, the filename changes, prompting the browser to download the new file.
3. Code Splitting: As previously discussed, splitting your code into smaller chunks can help reduce the initial load time.
4. Analyze Bundle Size: Tools like webpack-bundle-analyzer can help visualize the size of your output files, allowing you to identify potential areas for optimization.
npm install --save-dev webpack-bundle-analyzer
Then, add it to your plugins:
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer'); module.exports = { plugins: [ new BundleAnalyzerPlugin(), ], };
Launching your build will now provide a visual representation of your bundle size.
Comparing Production and Development Builds
Development builds are focused on improving the developer experience, while production builds aim for performance and optimization. Important differences include:
1. Mode: Development mode is set to development
, which provides detailed error messages and warnings. Production mode is set to production
, enabling optimizations like minification and tree shaking.
2. Source Maps: Development builds typically include source maps for easier debugging, while production builds may disable or use less detailed source maps to reduce size.
3. Performance Optimizations: Production builds include optimizations such as minification, dead code elimination, and caching strategies to enhance performance.
Example configuration for production:
// webpack.config.js module.exports = { mode: 'production', devtool: 'source-map', // generates source maps for production };
Conversely, for development:
// webpack.config.js module.exports = { mode: 'development', devtool: 'inline-source-map', // better for debugging };