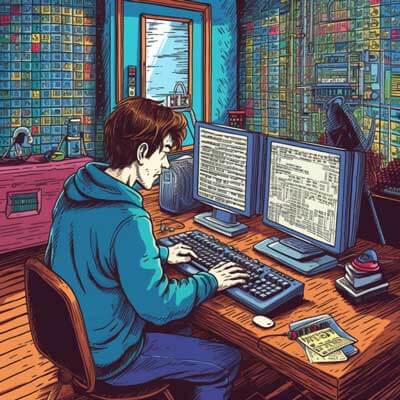
Table of Contents
Overview
The Webpack Manifest Plugin is a tool that generates a manifest file for the assets emitted by Webpack. This manifest file serves as a mapping between the logical names of assets and their corresponding physical file paths. The primary purpose of the plugin is to help manage the output files generated by Webpack, especially in scenarios involving cache busting and asset management.
When assets are built, their names may change due to hash generation for cache control. This can make it difficult to reference these assets in HTML files or other places. The Manifest Plugin addresses this issue by providing a JSON file that contains the mappings of the original asset names to the newly generated names.
Related Article: How to Fix webpack not recognized as a command error
How the Manifest Plugin Functions
The Manifest Plugin works by collecting information about the generated assets during the Webpack build process. It listens to the compilation events and records the output filenames along with their original paths. By the end of the build, it writes this information to a manifest file, typically in JSON format.
The plugin is configured in the Webpack configuration file. When the build process is initiated, the plugin hooks into Webpack's lifecycle, collects the asset data, and generates the manifest file.
Here is a simple example of how it fits into the Webpack configuration:
// webpack.config.js const ManifestPlugin = require('webpack-manifest-plugin'); module.exports = { // other configuration options plugins: [ new ManifestPlugin() ], };
Benefits
Using the Manifest Plugin provides several advantages:
1. Simplified Asset Management: The generated manifest file allows developers to reference assets without worrying about their hashed names.
2. Cache Busting: When asset files change, their names change too. The manifest file allows for easy updates in HTML or other files to point to the correct asset names.
3. Improved Build Processes: The plugin integrates seamlessly into the build process, providing a clear overview of what files were generated.
4. Better Collaboration: With a consistent mapping of asset names, teams can collaborate more efficiently on front-end projects.
Configuration Steps for the Manifest Plugin
Configuring the Manifest Plugin requires a few simple steps:
1. Install the Plugin: First, install the plugin using npm or yarn:
npm install webpack-manifest-plugin --save-dev
2. Update Webpack Configuration: Modify the Webpack configuration file to include the plugin. Here’s an example:
// webpack.config.js const { WebpackManifestPlugin } = require('webpack-manifest-plugin'); module.exports = { // other configuration options plugins: [ new WebpackManifestPlugin({ fileName: 'manifest.json', // output file name publicPath: '/', // optional, specify public path }) ], };
3. Build Your Project: Run the Webpack build command to generate the manifest file.
npx webpack --config webpack.config.js
Related Article: How to Configure SVGR with Webpack
Compatibility of the Manifest Plugin with Other Plugins
The Manifest Plugin is compatible with various other Webpack plugins. It can work alongside plugins like HtmlWebpackPlugin, MiniCssExtractPlugin, and others that modify or manage asset outputs.
When using the HtmlWebpackPlugin, for instance, the manifest can help ensure that the correct asset paths are injected into the HTML files. Here’s how it could be integrated:
// webpack.config.js const HtmlWebpackPlugin = require('html-webpack-plugin'); const { WebpackManifestPlugin } = require('webpack-manifest-plugin'); module.exports = { // other configuration options plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), new WebpackManifestPlugin() ], };
Common Use Cases
Several scenarios benefit from the use of the Manifest Plugin:
1. Single Page Applications (SPAs): In SPAs, managing assets dynamically is crucial; the manifest simplifies referencing assets that may change.
2. Microservices Architecture: When different services may produce assets with similar names, the manifest helps uniquely identify them.
3. Asset Versioning: For projects that require strict asset versioning, the manifest provides a clear reference to the current version of an asset.
4. Static Site Generation: When generating static sites, the manifest can help ensure that links to assets are always accurate.
Structure of the Generated Manifest File
The manifest file generated by the plugin is typically in JSON format. The structure of this file includes mappings of original file names to the generated file names. Here is an example of what the manifest file might look like:
{ "main.js": "main.123456.js", "style.css": "style.abcdef.css" }
Each key in the JSON object corresponds to the original asset name, while the value is the hashed name of the generated asset. This structure makes it easy to reference assets programmatically.
Compatibility with Different Versions
The Manifest Plugin is generally compatible with various Webpack versions; however, specific features may depend on the version of Webpack being used. It is essential to refer to the plugin’s documentation for compatibility notes when using newer versions of Webpack.
For example, if using Webpack 5 or later, ensure the plugin version aligns with the Webpack version to avoid any potential issues.
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Alternatives
While the Manifest Plugin is widely used, there are alternative plugins and methods for managing asset references:
1. HtmlWebpackPlugin: This plugin can automatically inject script and link tags into your HTML files based on the assets generated.
2. Webpack Asset Manifest: Similar to the Manifest Plugin, it generates a manifest but may offer different configuration options.
3. Custom Scripts: Some developers prefer to write custom scripts to manage asset paths, though this can become cumbersome for larger projects.
Troubleshooting Issues with the Manifest Plugin
Common issues that may arise when using the Manifest Plugin include:
1. Manifest File Not Generated: Ensure that the plugin is correctly included in the Webpack configuration file and that the build process is running without errors.
2. Incorrect Paths in Manifest: Check the configuration options provided to the plugin. Ensure that the publicPath
and fileName
options are set correctly.
3. Version Conflicts: If using multiple Webpack plugins, ensure that there are no conflicts between their configurations.
4. Build Errors: If the build fails, check the console for errors related to the plugin. Often, these can provide clues for resolving the issue.
Bundle Management with the Manifest Plugin
The Manifest Plugin aids in bundle management by creating a clear mapping of all generated assets. It allows developers to easily update references in their code based on the latest built files. This becomes especially important in large applications where many bundles are created.
Chunk Optimization
Chunk optimization is a key concern in modern web development. The Manifest Plugin can help with this by allowing developers to understand which chunks are being generated and their corresponding names.
Using the manifest, developers can analyze which chunks are larger than expected or not being used effectively. This insight can lead to better strategies for code splitting and chunking, ultimately improving load times and performance.
Related Article: How to Use Extract Text Webpack Plugin
Loader Configuration
The Manifest Plugin does not require specific loader configurations, as it operates at the plugin level. However, ensuring that loaders for assets (like images, fonts, etc.) are configured correctly will ensure that the manifest contains all relevant assets.
For example, if using file-loader for images, the configuration might look like this:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(png|jpe?g|gif|svg)$/, use: [ { loader: 'file-loader', options: { name: '[name].[hash].[ext]', outputPath: 'images/', }, }, ], }, ], }, };
This ensures that images are hashed and can be tracked in the manifest.
Asset Management Techniques
Managing assets can be challenging without a clear strategy. The Manifest Plugin helps by providing a JSON file that serves as a reference point for all generated assets.
Developers can implement techniques such as:
1. Dynamic Imports: Use dynamic imports for lazy loading of assets, which can then be referenced through the manifest.
2. Environment-Specific Builds: Generate different manifests for different environments (development, production) by configuring the plugin accordingly.
3. Automated Asset Updates: Write scripts that read from the manifest to update asset references in HTML or JavaScript files automatically.
Code Splitting Strategies Using the Manifest Plugin
Code splitting allows developers to break up their application into smaller chunks. The Manifest Plugin plays a vital role in this by providing a clear mapping of these chunks.
Strategies for code splitting might include:
1. Entry Points: Define multiple entry points in the Webpack configuration to create separate bundles for different parts of the application.
2. Dynamic Imports: Use dynamic imports for specific modules that are not needed immediately. The manifest will reflect these chunks, allowing for optimized loading.
3. Vendor Splitting: Separate third-party libraries into their own chunks to facilitate caching.
Example of entry points configuration:
// webpack.config.js module.exports = { entry: { main: './src/index.js', admin: './src/admin.js', }, };
This creates separate bundles for the main application and the admin section.
Tree Shaking Benefits
Tree shaking refers to the elimination of unused code during the build process. The Manifest Plugin supports tree shaking by ensuring that only the necessary assets are included in the manifest file.
When used alongside ES6 modules, Webpack can identify and remove unused exports, leading to smaller bundle sizes. The manifest will help developers track which assets are actually being used, further enhancing the tree shaking process.
To enable tree shaking, ensure that the mode
is set to production
in the Webpack configuration:
// webpack.config.js module.exports = { mode: 'production', };
This tells Webpack to optimize the build, including tree shaking.
Related Article: How to Use webpack -d for Development Mode
Hot Module Replacement
Hot Module Replacement (HMR) allows developers to update modules in a running application without a full refresh. The Manifest Plugin can complement HMR by ensuring that the correct asset paths are maintained during development.
When using HMR, the manifest can help track which modules have been updated and their corresponding paths, minimizing the risk of broken references.
To enable HMR, add the following to the Webpack development server configuration:
// webpack.config.js module.exports = { devServer: { hot: true, }, };
This allows for a smoother development experience with live updates.
Dependency Graph Insights
The Manifest Plugin provides insights into the dependency graph of your application by documenting how assets relate to each other. This can help in understanding which files are being included in the build and how they are interconnected.
To visualize your bundle, you might integrate the following:
npm install webpack-bundle-analyzer --save-dev
Then, add it to your Webpack configuration:
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer'); module.exports = { plugins: [ new BundleAnalyzerPlugin(), ], };
This combination of tools will reveal insights into the overall structure and dependencies of your application.