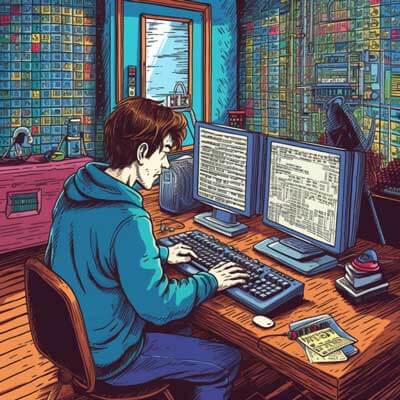
Table of Contents
Overview
Webpack is a popular module bundler used in modern web development. It allows developers to compile JavaScript modules into a single file or smaller files for optimized loading. One of the techniques to improve the bundle size and load performance is through the use of Node externals. This approach helps to exclude certain modules from being bundled, especially those that are not necessary for the client-side application, such as Node.js modules used in server-side code.
Related Article: How to Use Terser Webpack Plugin for Code Minimization
What are Externals?
Externals refer to modules or dependencies that are not included in the final bundled output by Webpack. Instead of bundling these dependencies, they are treated as external resources that the application can reference at runtime. This is particularly useful for large libraries or frameworks that are already available in the environment, such as React or Express, allowing the application to keep its bundle size smaller.
How to Configure Externals in a Build Tool
Configuring externals in Webpack is done in the webpack.config.js
file. You can specify which modules should be treated as externals by adding the externals
property to the configuration object.
Example configuration:
// webpack.config.js module.exports = { // Other configurations... externals: { // Prevent bundling of 'react' and 'react-dom' react: 'React', 'react-dom': 'ReactDOM', }, };
In this example, React and ReactDOM are marked as externals. Webpack will not include these libraries in the bundle, assuming they will be available globally.
Why Utilize Externals for Bundle Optimization
Using externals can significantly reduce the size of the final bundle. By excluding large libraries that may already be present in the environment, the overall load time of the application can improve. This is particularly useful for applications that leverage CDNs to deliver popular libraries.
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Difference Between Externals and Regular Modules
Regular modules are included in the bundle created by Webpack, meaning their code is packaged alongside the application. Externals, on the other hand, are not included in the bundle. The key difference lies in how they are loaded; externals rely on being available in the environment when the application runs, while regular modules are bundled and served with the application.
Using Externals for Node Modules
When developing server-side applications with Node.js, many libraries may not need to be bundled. For instance, libraries like express
or mongoose
can be marked as externals. This is done in a similar manner as shown before.
Example configuration for a Node.js application:
// webpack.config.js module.exports = { target: 'node', // Specify the build target externals: { express: 'commonjs express', // Use CommonJS format mongoose: 'commonjs mongoose', }, };
Setting the target to node
informs Webpack that the output will run in a Node.js environment.
Bundle Size
The impact of using externals is directly visible in the bundle size. By excluding large libraries, the size of the output file decreases, which in turn reduces the amount of data transferred when loading the application. For example, excluding a library that takes up several hundred kilobytes can lead to noticeable improvements in load times, especially for users on slower connections.
Common Use Cases for Externals in Projects
Externals are commonly used in various scenarios, such as:
- Applications that use React, Angular, or Vue.js and load these libraries via a CDN.
- Server-side applications built with Node.js where backend libraries should not be bundled.
- Microservices architecture where multiple services may share the same dependencies.
In these cases, marking certain libraries as externals can streamline the bundle process and enhance performance.
Related Article: How to Use the Fork TS Checker Webpack Plugin
Preventing Modules from Being Bundled
To prevent specific modules from being bundled, simply list them in the externals
configuration. This can include both third-party libraries and custom modules.
Example:
// webpack.config.js module.exports = { externals: { lodash: 'lodash', // Prevent lodash from being bundled myCustomModule: 'myCustomModule', // Prevent custom module from being bundled }, };
This ensures that both lodash
and myCustomModule
will not be included in the final bundle.
Tree Shaking and Externals in Bundle Optimization
Tree shaking is a technique used to eliminate dead code from the final bundle. It works best with ES6 module syntax (import/export). While externals help reduce bundle size by excluding entire modules, tree shaking focuses on removing unused parts of the code.
When using externals, it's essential to ensure that the remaining code is optimized through tree shaking. This can be achieved by using ES6 imports for modules that are included in the bundle.
Code Splitting Techniques
Code splitting allows developers to split their code into smaller chunks, which can be loaded on demand. When combined with externals, this approach can further enhance performance.
Example configuration:
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, externals: { react: 'React', }, };
This configuration splits the code into separate chunks while treating React as an external dependency.
Dynamic Imports
Dynamic imports enable lazy loading of modules, improving load times by only loading code when it's needed. When working with externals, dynamic imports can be used in conjunction with them, but it's crucial to ensure that the external dependencies are available when the dynamic import is executed.
Example usage of dynamic imports:
// Example of dynamic import async function loadComponent() { const module = await import('./MyComponent'); const MyComponent = module.default; // Use MyComponent... }
If MyComponent
relies on an external library, ensure that the library is already loaded in the environment.
Related Article: How to Optimize CSS Assets with Webpack Plugin
Managing Dependencies
Managing dependencies effectively involves careful planning of which libraries to include as externals. Regularly review the dependencies of your project and consider the following:
- Are there large libraries that can be loaded from a CDN?
- Are there libraries that are only needed in specific environments (e.g., server-side only)?
- Can certain libraries be excluded in production builds but included in development?
Performance Tuning
Performance tuning requires attention to how externals are configured. Use tools like Webpack Bundle Analyzer to visualize the impact of externals on bundle size. This helps identify areas for further optimization.
Example command to analyze the bundle:
npx webpack-bundle-analyzer dist/stats.json
This command generates a visual representation of the bundle, allowing for easy identification of what is included and what can be excluded.
Versioning Strategies
With externals, managing versioning is crucial. Ensure that the external libraries are compatible with your application. Some strategies include:
- Locking specific versions in your configuration.
- Regularly updating dependencies to avoid compatibility issues.
- Using a package manager like npm or yarn to manage versions effectively.
Documenting the external versions used in your application can help maintain consistency across different environments.
Plugin Architecture
Webpack's plugin architecture allows for further customization of how externals are handled. Plugins can be created or configured to modify the behavior of externals.
Example of using a plugin:
// webpack.config.js const webpack = require('webpack'); module.exports = { plugins: [ new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify('production'), }), ], externals: { react: 'React', }, };
This setup uses the DefinePlugin
to set environment variables, which can be beneficial when working with externals.
Related Article: How to Set Up Webpack Proxy for Development
Loaders
Loaders in Webpack transform files before they are added to the bundle. While externals are not bundled, loaders can still process other files. For instance, if you have CSS or images that need to be transformed, loaders will handle them accordingly, while ignoring the externals.
Example of using a loader:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, externals: { react: 'React', }, };
This configuration uses loaders for CSS files, while treating React as an external dependency.
Assets Management
Managing assets such as images, fonts, or styles is crucial in web development. When using externals, ensure that your assets are handled properly within the bundle. Webpack can still manage these assets through loaders and plugins without affecting the externals configuration.
Example of asset management:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(png|jpg|gif)$/, use: ['file-loader'], }, ], }, externals: { react: 'React', }, };
In this setup, images are processed by the file-loader
, while React is excluded from the bundle.
Debugging
Debugging issues with externals can be challenging. Common problems include:
- External libraries not being found at runtime.
- Version mismatches between the bundled application and external dependencies.
- Issues related to module resolution.
To troubleshoot these issues, consider the following steps:
- Check the console for errors related to missing modules.
- Verify that the external libraries are loaded correctly in the environment.
- Ensure consistent versioning across development and production environments.
Additional Resources
- Understanding Webpack Externals