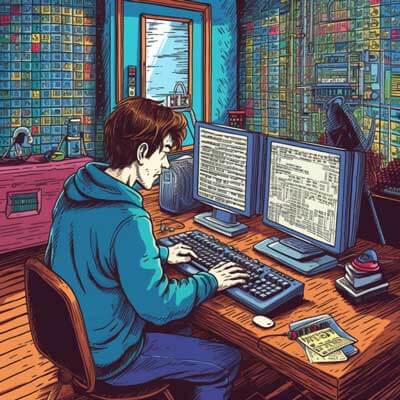
Table of Contents
Overview
Tree shaking is the process of removing unused code from the final output of a module bundler. It works by analyzing the dependency graph of the application, identifying which code is actually used, and excluding the rest. This is especially relevant in modern JavaScript applications that rely on libraries and frameworks, where only a fraction of the imported code may be necessary. The term "tree shaking" is derived from the idea of shaking a tree to remove the dead leaves, leaving only the necessary branches.
Related Article: How to Use Webpack CopyPlugin for Asset Management
How Dead Code Elimination Works with Tree Shaking
Dead code elimination is the core mechanism behind tree shaking. When a module bundler processes your code, it constructs a dependency graph. This graph shows how different modules depend on each other. During the bundling process, the bundler checks which modules are actually referenced in the code. If a module or a piece of code within a module is not used anywhere in the application, it can be safely excluded from the final bundle. This results in a smaller, more efficient bundle.
For example, consider the following code:
// utils.js export function usedFunction() { console.log('This function is used.'); } export function unusedFunction() { console.log('This function is not used.'); }
If usedFunction
is the only one imported in your application, unusedFunction
will be removed during the tree shaking process.
Using ES6 Modules for Tree Shaking Benefits
Tree shaking relies heavily on the use of ES6 module syntax (also known as ES2015 modules). This syntax allows for static analysis of the code, which is crucial for determining which parts can be removed. Unlike CommonJS modules, which use dynamic require
statements, ES6 modules use import
and export
statements that are static. This static structure allows bundlers to analyze the code during the build process.
When using ES6 modules, the following syntax is used:
// module.js export const a = 1; export const b = 2; export function sum(x, y) { return x + y; }
In this example, if only the sum
function is used in the application, the bundler can exclude the variables a
and b
from the final bundle.
Implementing Import/Export Syntax in Code
To implement tree shaking, the code must use the ES6 module syntax for importing and exporting functions, variables, or objects. Here’s a basic example of how to structure a module and then import it:
// math.js export function add(x, y) { return x + y; } export function subtract(x, y) { return x - y; }
// main.js import { add } from './math'; console.log(add(5, 3)); // 8
In this scenario, the subtract
function is not imported or used in main.js
, so it can be removed during the tree shaking process.
Related Article: How to Use Extract Text Webpack Plugin
How to Enable Tree Shaking in Configuration
To enable tree shaking in a Webpack configuration, certain settings must be applied. The most crucial step is to ensure that the mode
is set to production
, as tree shaking is enabled by default in production mode. Here’s an example of a basic Webpack configuration:
// webpack.config.js const path = require('path'); module.exports = { mode: 'production', entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, optimization: { usedExports: true, // Enables tree shaking } };
Setting usedExports
to true
helps Webpack identify which exports are used in your application, allowing for effective tree shaking.
Requirements for Tree Shaking to Function
For tree shaking to work effectively, certain requirements must be met:
1. Use ES6 Modules: The code must be written using ES6 import
and export
syntax.
2. Production Mode: The bundler must be configured to run in production mode, as tree shaking is typically disabled in development mode.
3. No Side Effects: The code should not have side effects. If importing a module causes changes to the program state, the bundler may not be able to safely remove it.
4. Proper Configuration: The bundler must be configured correctly, which includes enabling tree shaking options.
Can Tree Shaking Remove Unused Library Code
Yes, tree shaking can remove unused code from libraries, but this depends on how the library is structured. If the library is written using ES6 module syntax, the bundler can analyze which parts of the library are used and exclude the rest. However, many popular libraries still use CommonJS or may not have been designed with tree shaking in mind. In these cases, tree shaking may not be as effective.
For example, if you import a library like lodash:
import { debounce } from 'lodash';
If lodash has been properly designed with ES6 modules, then unused functions will not be included in the bundle.
Difference Between Tree Shaking and Code Splitting
Tree shaking and code splitting are two different optimization techniques used in module bundling, but they serve different purposes.
- Tree Shaking: Refers to the removal of unused code from the final bundle. It works by analyzing which parts of the code are actually used, thereby reducing the total bundle size.
- Code Splitting: Involves breaking the bundle into smaller chunks that can be loaded on demand. This means that not all code needs to be loaded at once, which can improve load times, especially for larger applications.
For example, you can use code splitting to load certain modules only when they are needed:
import(/* webpackChunkName: "myChunk" */ './myModule').then(module => { module.default(); });
This syntax tells Webpack to create a separate chunk for myModule
, which will be loaded only when required.
Related Article: How to Use Webpack Manifest Plugin
Performance Impact
The impact of tree shaking on performance can be significant. By eliminating unused code, the final bundle size decreases, which leads to faster load times and a better user experience. Smaller bundles also reduce the amount of data transferred over the network, which is especially crucial for users on slower connections.
Additionally, tree shaking can improve the performance of the JavaScript engine by allowing it to execute less code, leading to better runtime performance. As a result, applications can become more responsive and performant, enhancing the overall experience for users.
Tools that Assist with Tree Shaking
Several tools assist with tree shaking when using Webpack, including:
1. Webpack: The primary module bundler that supports tree shaking natively in production mode.
2. Terser: A JavaScript minifier that can further reduce bundle size by stripping out comments, whitespace, and dead code after tree shaking has been performed.
3. Webpack Bundle Analyzer: A tool that visualizes the size of webpack output files with an interactive zoomable treemap. It helps developers understand the impact of tree shaking and other optimizations.
To install the Webpack Bundle Analyzer, use the following command:
npm install --save-dev webpack-bundle-analyzer
You can then integrate it into your Webpack configuration:
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer'); module.exports = { // other configurations... plugins: [ new BundleAnalyzerPlugin() ] };
Tree Shaking Compatibility with CommonJS Modules
Tree shaking is not fully compatible with CommonJS modules. CommonJS uses dynamic require
statements, which makes it difficult for bundlers to determine which code is actually used. This often leads to the entire module being included in the final bundle, regardless of whether all parts are utilized.
For example, if a CommonJS module exports multiple functions, and only one is used, the bundler may still include the entire module:
// commonjsModule.js module.exports = { usedFunction: function() {}, unusedFunction: function() {} }; // main.js const { usedFunction } = require('./commonjsModule');
In this example, the bundler may include both usedFunction
and unusedFunction
in the final output, making tree shaking ineffective.
Verifying Tree Shaking in Your Project
To verify that tree shaking is working in your project, you can check the size of your bundle before and after implementing tree shaking. One way to do this is to use tools like Webpack Bundle Analyzer to visualize your bundle. This tool will show you which modules are included and how large they are, allowing you to see if unused code has been removed.
Another method is to inspect the output files directly. After building your project in production mode, look for the presence of unused functions or variables in the final bundle. You can also look at the source maps to trace back the code and confirm which parts were included or excluded.
Related Article: How to Set Up Webpack SCSS Loader
Setting Up Minification with UglifyJS
To further optimize your bundled code after tree shaking, you can set up minification using UglifyJS. Minification reduces the size of the output files by removing whitespace, comments, and other unnecessary characters.
First, install UglifyJS as a plugin for Webpack:
npm install --save-dev uglifyjs-webpack-plugin
Then, configure it in your Webpack configuration:
const UglifyJsPlugin = require('uglifyjs-webpack-plugin'); module.exports = { // other configurations... optimization: { minimizer: [new UglifyJsPlugin()], }, };
With this setup, UglifyJS will automatically minify your JavaScript files during the build process.
Configuring Code Splitting for Optimization
Code splitting can be configured in Webpack to optimize loading times and further enhance performance. You can use dynamic imports to split your code into separate chunks:
// main.js import('./moduleA').then(moduleA => { moduleA.default(); });
In this example, moduleA
will be loaded only when it is needed. This can be particularly useful for large applications where you want to load certain parts of the application on demand.
To configure code splitting in your Webpack configuration, you can use the optimization.splitChunks
option:
module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, };
This configuration tells Webpack to split all chunks of code, allowing for more modular loading of your application.
Examining the Tree Shaking Algorithm
The tree shaking algorithm analyzes the dependency graph built by the bundler. It follows these general steps:
1. Build the Dependency Graph: The bundler constructs a graph of all modules and their dependencies.
2. Identify Exports: It identifies which modules export functions or variables.
3. Trace Usage: The algorithm traces which exports are actually used within the application.
4. Eliminate Unused Code: Finally, the bundler removes any code that is not referenced anywhere in the application.
This process allows for thorough analysis of the code, ensuring that only necessary parts are included in the final bundle.
Alternatives to Webpack: Using Rollup for Tree Shaking
Rollup is another module bundler that also supports tree shaking. It is particularly well-suited for libraries and smaller projects where the focus is on producing optimized output. Rollup uses a similar approach to Webpack, analyzing the dependency graph and removing unused code.
To get started with Rollup, you need to install it:
npm install --save-dev rollup
Then, create a configuration file:
// rollup.config.js export default { input: 'src/index.js', output: { file: 'dist/bundle.js', format: 'es' }, treeshake: true // Enable tree shaking };
This configuration enables tree shaking by default in Rollup. By using Rollup, developers can achieve similar benefits as with Webpack, while enjoying a different set of features and optimizations focused on module bundling.
Related Article: How to Use ESLint Webpack Plugin for Code Quality
Additional Resources
- Understanding Tree Shaking in Webpack