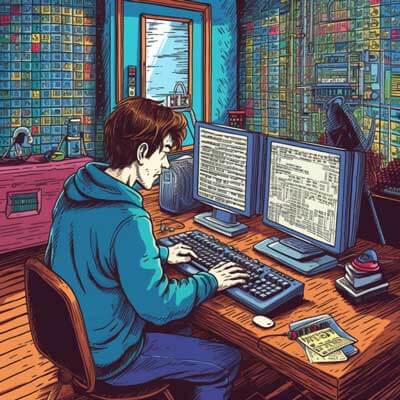
Table of Contents
The window load and document ready functions in JavaScript are essential for executing code when a web page finishes loading. These functions ensure that the necessary resources, such as images and stylesheets, are fully loaded before executing the code. In this answer, we will explore how to use these functions effectively in the context of jQuery.
Window Load Function
The window load function is triggered when all the resources on a web page, including images, scripts, and stylesheets, have finished loading. This function is useful when you need to perform actions that rely on the complete loading of the page.
To use the window load function in jQuery, you can use the following syntax:
$(window).on('load', function() { // Your code here });
In this example, the code inside the function will be executed once all the resources have loaded. This is particularly useful when you need to manipulate elements that require the complete loading of the page, such as resizing images or calculating the dimensions of elements that depend on images.
Related Article: How to Remove an Object From an Array in Javascript
Document Ready Function
The document ready function, on the other hand, is triggered when the HTML document has been completely parsed and the DOM (Document Object Model) is ready for manipulation. This function allows you to perform actions as soon as the DOM is ready, even if some external resources like images are still loading.
To use the document ready function in jQuery, you can use the following syntax:
$(document).ready(function() { // Your code here });
Alternatively, you can use a shorthand version of the document ready function:
$(function() { // Your code here });
Both versions have the same effect of executing the code inside the function as soon as the DOM is ready.
Using the document ready function is a best practice because it allows your code to execute as soon as possible without waiting for all external resources to load. This can greatly improve the perceived performance of your web page.
Choosing Between Window Load and Document Ready
When deciding whether to use the window load or document ready function, consider the following guidelines:
- Use the window load function when your code relies on the complete loading of external resources, such as images or stylesheets. This ensures that your code will only execute when all resources are available.
- Use the document ready function when your code only needs the DOM to be ready for manipulation. This allows your code to execute as soon as possible, improving the perceived performance of your web page.
It's important to note that the window load function will only be triggered after all resources have finished loading, which may cause a delay in the execution of your code. On the other hand, the document ready function will be triggered as soon as the DOM is ready, even if some external resources are still loading.
Alternative Approaches
While the window load and document ready functions in jQuery are commonly used, there are alternative approaches available in plain JavaScript:
- Using the window.onload
event: This event is similar to the window load function in jQuery and is triggered when all resources have finished loading. You can use it as follows:
window.onload = function() { // Your code here };
- Using the DOMContentLoaded
event: This event is similar to the document ready function in jQuery and is triggered when the DOM is ready for manipulation. You can use it as follows:
document.addEventListener('DOMContentLoaded', function() { // Your code here });
Both of these approaches achieve the same result as their jQuery counterparts but without the need for the jQuery library.