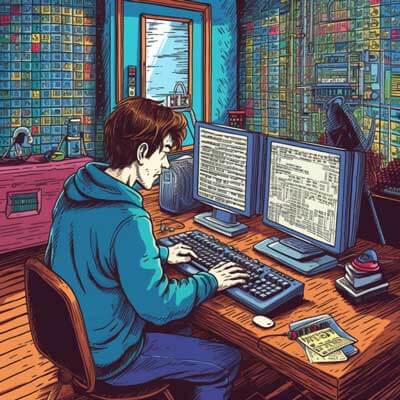
Table of Contents
What is Elixir?
Elixir is a functional, concurrent, and general-purpose programming language that runs on the Erlang virtual machine (BEAM). It was created by José Valim in 2011 with the goal of providing a scalable and maintainable language for building robust and fault-tolerant applications. Elixir combines the best features of functional programming with a syntax inspired by Ruby, resulting in a language that is both expressive and efficient.
One of the key features of Elixir is its support for lightweight processes called "actors." These processes are isolated from each other and communicate through message passing, allowing for highly concurrent and distributed systems. Elixir also provides useful abstractions for handling failures and building fault-tolerant applications.
Elixir's ecosystem is built upon the battle-tested Erlang ecosystem, which provides a rich set of libraries and tools for building scalable and fault-tolerant systems. With its emphasis on concurrency, fault tolerance, and scalability, Elixir is well-suited for building enterprise-grade applications.
Related Article: Deployment Strategies and Scaling for Phoenix Apps
What is Phoenix?
Phoenix is a web development framework written in Elixir that follows the model-view-controller (MVC) architectural pattern. It is designed for building scalable and high-performance web applications with a focus on developer productivity. Phoenix borrows many concepts from Ruby on Rails, making it easy for developers familiar with Rails to transition to Phoenix.
Phoenix provides a robust set of features out of the box, including routing, controllers, views, and templates. It also includes a useful and flexible database layer called Ecto, which supports multiple databases and provides a rich set of tools for working with data.
One of the key advantages of Phoenix is its performance. It is built on top of the BEAM virtual machine, which is known for its scalability and fault tolerance. Phoenix applications can handle a large number of concurrent connections and are well-suited for building real-time applications using WebSockets.
How does Single Sign-On work?
Single Sign-On (SSO) is a mechanism that allows users to authenticate once and access multiple applications without needing to re-enter their credentials. It provides a seamless and convenient user experience by eliminating the need for users to manage multiple usernames and passwords.
The basic flow of SSO involves three parties: the identity provider (IdP), the service provider (SP), and the user. Here's how it works:
1. The user tries to access a protected resource on the SP's application.
2. The SP redirects the user to the IdP's login page.
3. The user enters their credentials on the IdP's login page.
4. The IdP validates the user's credentials and generates a security token, also known as an assertion.
5. The IdP redirects the user back to the SP's application along with the security token.
6. The SP validates the security token with the IdP to ensure its authenticity and integrity.
7. Once the security token is validated, the user is considered authenticated and granted access to the protected resource.
This flow allows users to authenticate once with the IdP and access multiple applications without needing to enter their credentials again. It simplifies the authentication process and improves user experience.
What is OAuth2?
OAuth2 is an open standard for authorization that allows third-party applications to access resources on behalf of a user. It provides a secure and standardized way for users to grant permissions to applications without sharing their credentials.
OAuth2 involves four main entities: the resource owner (user), the client (application), the authorization server (OAuth provider), and the resource server (API). Here's how it works:
1. The client requests authorization from the resource owner to access their resources.
2. The resource owner authenticates with the authorization server and grants permission to the client.
3. The authorization server issues an access token to the client.
4. The client presents the access token to the resource server when requesting access to protected resources.
5. The resource server validates the access token and grants or denies access to the requested resources.
OAuth2 provides different grant types for different scenarios, such as authorization code grant, implicit grant, client credentials grant, and resource owner password credentials grant. Each grant type has its own requirements and security considerations.
Related Article: Phoenix with Bootstrap, Elasticsearch & Databases
How can I integrate Phoenix with LDAP for enterprise authentication?
LDAP (Lightweight Directory Access Protocol) is a protocol for accessing and managing directory services. It is commonly used for enterprise authentication, where user information is stored in a centralized directory.
To integrate Phoenix with LDAP for enterprise authentication, you can use the exldap
library, which provides a convenient API for interacting with LDAP servers in Elixir.
Here's an example of how you can integrate Phoenix with LDAP for authentication:
1. Add the exldap
dependency to your mix.exs file:
defp deps do [ {:exldap, "~> 1.0"} ] end
2. Install the dependency by running mix deps.get
.
3. Configure the LDAP connection in your config/config.exs file:
config :exldap, connections: [ default: [ host: "ldap.example.com", port: 389, bind_dn: "cn=admin,dc=example,dc=com", bind_password: "password", base_dn: "dc=example,dc=com" ] ]
4. Create a module to handle the LDAP authentication logic, for example, MyApp.LDAP
:
defmodule MyApp.LDAP do def authenticate(username, password) do case Exldap.bind(username, password) do {:ok, _} -> :ok {:error, _} -> :error end end end
5. In your Phoenix controller, call the MyApp.LDAP.authenticate/2
function to authenticate the user:
defmodule MyApp.SessionController do use MyApp.Web, :controller def create(conn, %{"username" => username, "password" => password}) do case MyApp.LDAP.authenticate(username, password) do :ok -> # User is authenticated, create a session and redirect # to the desired page. :error -> # Authentication failed, show an error message. end end end
This example demonstrates a basic integration of Phoenix with LDAP for enterprise authentication. You can customize it further based on your specific requirements and LDAP server configuration.
How can I implement Single Sign-On in Phoenix with OAuth2?
Implementing Single Sign-On (SSO) in Phoenix with OAuth2 involves configuring Phoenix to act as a client application and integrating it with an OAuth2 provider.
Here's an example of how you can implement SSO in Phoenix with OAuth2:
1. Install the oauth2
library by adding it to your mix.exs file:
defp deps do [ {:oauth2, "~> 2.0"} ] end
2. Install the dependency by running mix deps.get
.
3. Configure the OAuth2 client in your config/config.exs file:
config :oauth2, client_id: "your_client_id", client_secret: "your_client_secret", redirect_uri: "http://localhost:4000/callback", authorize_url: "https://oauth2-provider.com/authorize", token_url: "https://oauth2-provider.com/token"
4. Create a module to handle the OAuth2 authentication logic, for example, MyApp.OAuth
:
defmodule MyApp.OAuth do def authorize_url(conn) do OAuth2.authorize_url(conn, :default) end def handle_callback(conn, params) do case OAuth2.handle_callback(conn, params, :default) do {:ok, token} -> # User is authenticated, create a session and redirect # to the desired page. {:error, reason} -> # Authentication failed, show an error message. end end end
5. In your Phoenix controller, call the MyApp.OAuth.authorize_url/1
function to redirect the user to the OAuth2 provider for authentication:
defmodule MyApp.SessionController do use MyApp.Web, :controller def new(conn, _params) do redirect(conn, external: MyApp.OAuth.authorize_url(conn)) end def callback(conn, %{"code" => code}) do MyApp.OAuth.handle_callback(conn, %{"code" => code}) end end
This example demonstrates a basic implementation of SSO in Phoenix with OAuth2. You can customize it further based on your specific requirements and OAuth2 provider configuration.
What are audit trails in Phoenix apps?
Audit trails are logs or records that capture and track important events and actions within a system. In the context of Phoenix apps, audit trails are used to record activities such as user logins, data modifications, and other critical operations. They provide a detailed history of actions performed within an application, which is essential for security, compliance, and troubleshooting purposes.
Implementing audit trails in Phoenix apps involves capturing relevant events and storing them in a persistent storage, such as a database. The logs or records typically include information such as the user who performed the action, the timestamp, the action itself, and any relevant data associated with the action.
Here's an example of how you can implement audit trails in a Phoenix app:
1. Create a database table to store the audit trail records. The table should have columns to store the user ID, timestamp, action, and any additional data.
2. In your Phoenix controllers or context modules, add code to capture relevant events and create audit trail records. For example, you can add a function to create an audit trail record for user logins:
defmodule MyApp.Audit do def log_login(user_id) do MyApp.Repo.insert(%MyApp.AuditTrail{user_id: user_id, action: "login"}) end end
3. Call the appropriate audit logging function whenever a relevant event occurs. For example, in your session controller, call MyApp.Audit.log_login(user_id)
when a user logs in.
4. Retrieve and display audit trail records as needed. For example, you can create a page in your Phoenix app to display the audit trail history, allowing administrators to review and analyze the recorded events.
How can I track compliance in Phoenix apps?
Tracking compliance in Phoenix apps involves ensuring that the application adheres to relevant regulations, standards, and internal policies. Compliance requirements can vary depending on the industry and the nature of the application. Some common areas of compliance include data privacy, security, accessibility, and industry-specific regulations.
Here are some steps to track compliance in Phoenix apps:
1. Identify the relevant compliance requirements based on the industry and the application's use case. For example, if the application handles personal data, compliance with data protection regulations such as GDPR (General Data Protection Regulation) may be necessary.
2. Review the existing codebase and infrastructure to identify any potential compliance issues. This may involve conducting a security audit, reviewing data handling processes, and ensuring proper access controls are in place.
3. Implement necessary controls and measures to address compliance requirements. This may include encrypting sensitive data, implementing access controls, conducting regular security assessments, and ensuring proper logging and monitoring.
4. Regularly review and update compliance measures to ensure ongoing adherence. Compliance requirements can change over time, so it's important to stay up to date with the latest regulations and best practices.
5. Conduct regular audits and assessments to verify compliance. This may involve internal or external audits, penetration testing, vulnerability scanning, and code reviews.
6. Document compliance measures and keep records of audits and assessments. This documentation is important for demonstrating compliance to regulators, clients, or auditors.
Related Article: Optimizing Database Queries with Elixir & Phoenix
Why is Elixir a good choice for enterprise functionalities?
Elixir is a good choice for enterprise functionalities for several reasons:
1. Scalability and Performance: Elixir's concurrency model, based on lightweight processes and message passing, allows for highly concurrent and distributed systems. This makes it well-suited for handling the high load and scalability requirements often found in enterprise applications.
2. Fault Tolerance: Elixir's supervision tree and built-in mechanisms for handling failures make it easy to build fault-tolerant systems. In enterprise applications where uptime and reliability are critical, Elixir's fault tolerance features can help ensure the application stays running even in the face of failures.
3. Productivity and Maintainability: Elixir's syntax, inspired by Ruby, is elegant and expressive, making it enjoyable to write and read code. The language also provides a useful toolset for building maintainable applications, such as pattern matching, macros, and a strong emphasis on code organization and separation of concerns.
4. Ecosystem and Community: Elixir is built on top of the battle-tested Erlang ecosystem, which provides a rich set of libraries and tools for building scalable and fault-tolerant systems. The Elixir community is vibrant and supportive, with many open-source libraries and resources available to help developers build enterprise-grade applications.
5. Integration with existing systems: Elixir can easily integrate with existing systems and technologies, making it a good choice for enterprises that already have established infrastructure and legacy systems. Elixir provides libraries and tools for working with databases, APIs, message queues, and more, allowing for seamless integration with existing systems.
Overall, Elixir's combination of scalability, fault tolerance, productivity, and integration capabilities make it a strong choice for building enterprise functionalities.
What are the benefits of using Single Sign-On in enterprise applications?
Using Single Sign-On (SSO) in enterprise applications offers several benefits:
1. Enhanced User Experience: SSO provides a seamless and convenient user experience by eliminating the need for users to remember and enter multiple usernames and passwords. Users can authenticate once and access multiple applications without needing to re-enter their credentials, improving efficiency and reducing frustration.
2. Improved Security: SSO allows for centralized authentication and access control. This means that user credentials are stored and managed in a single location, reducing the risk of weak or compromised passwords. It also simplifies the management of user access rights, making it easier to enforce security policies and revoke access when necessary.
3. Simplified User Provisioning and Deprovisioning: With SSO, user provisioning and deprovisioning can be centralized, making it easier to onboard new users and remove access for departing employees. This simplifies user management processes and reduces the risk of orphaned accounts.
4. Compliance and Auditing: SSO provides a centralized point of control for authentication and access, making it easier to track and audit user activities. This is particularly important in enterprise applications where compliance with regulations and industry standards is required. SSO allows for better visibility and accountability, helping organizations meet compliance requirements and pass audits.
5. Cost and Time Savings: Implementing SSO can result in cost and time savings for both users and IT departments. Users save time by not having to remember and enter multiple credentials, while IT departments save time by reducing the number of password-related support requests. Additionally, SSO can streamline the development and maintenance of authentication systems, reducing development and operational costs.