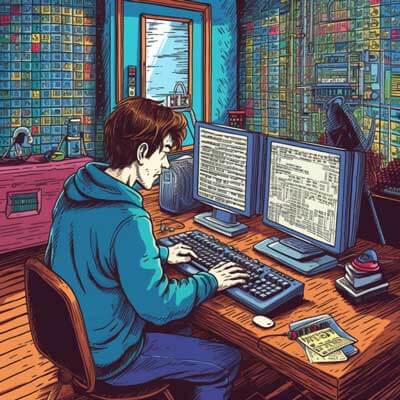
Table of Contents
The Purpose of the Render Method in ReactJS
The render method is a fundamental part of ReactJS. It is responsible for rendering the JSX (JavaScript XML) code onto the DOM (Document Object Model), which is the browser's representation of the webpage. The render method is called whenever there is a change in the component's state or props, and it determines what should be displayed on the screen.
In ReactJS, components are the building blocks of the user interface, and they are defined as JavaScript classes or functions. Each component can have a render method, which returns JSX code that describes the structure and content of the component's UI. This JSX code can include HTML-like elements, as well as custom React components.
Here is an example of a simple React component with a render method:
import React from 'react'; class MyComponent extends React.Component { render() { return ( <div> <h1>Hello, World!</h1> <p>This is my first React component.</p> </div> ); } } export default MyComponent;
In this example, the render method of the MyComponent class returns JSX code that represents a div element containing an h1 element and a p element. When this component is rendered onto the DOM, the browser will display the text "Hello, World!" inside the h1 element and the text "This is my first React component." inside the p element.
It's important to note that the render method should be pure, meaning it should not modify the component's state or props. Instead, it should only return the JSX code that describes the UI based on the current state and props. React will take care of updating the DOM efficiently when the component's state or props change.
Related Article: How to Render ReactJS Code with NPM
Understanding the For Loop in ReactJS
In JavaScript, a for loop is a control flow statement that allows you to repeatedly execute a block of code a certain number of times. It is commonly used to iterate over arrays or perform a specific task a fixed number of times. However, in ReactJS, using a traditional for loop inside the render method is not recommended because it can lead to performance issues and make the component's code harder to understand and maintain.
Instead of using a for loop, React provides other mechanisms for iterating over data and rendering dynamic content. One such mechanism is the map function, which we will explore in more detail later in this article. The map function allows you to iterate over an array and transform each element into JSX code, which can then be rendered by the render method.
While it is technically possible to use a for loop inside the render method, it is generally discouraged because it goes against the declarative nature of React. React encourages you to think in terms of data flow and component composition, rather than imperative control flow statements like for loops.
Using For Loop in Render Method
As mentioned earlier, using a for loop in the render method of a React component is not recommended. However, if you still want to use a for loop for some reason, you can do so by encapsulating the loop inside a JavaScript block, and then use the returned JSX code from the loop inside the render method.
Here is an example of using a for loop inside the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; // Using a for loop inside the render method const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li key={i}>{items[i]}</li>); } return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of items and we want to render each item as a list item inside an unordered list. We use a for loop to iterate over the items array, create a new JSX element for each item, and push it into the renderedItems array. Finally, we render the renderedItems array inside the ul element using curly braces.
While this approach works, it is not recommended because it can make the code harder to read and maintain. It is generally better to use more declarative and functional approaches, such as the map function or other higher-order functions provided by React.
Iterating and Looping in ReactJS
When it comes to iterating and looping in ReactJS, there are several approaches you can take, depending on the specific requirements of your application. Here, we will explore some of the common methods used in React components to iterate over data and render dynamic content.
Related Article: Implementing HTML Templates in ReactJS
The map function
The map function is a useful tool in React for iterating over an array and transforming each element into JSX code. It takes a callback function as an argument, which is executed for each element in the array. The callback function receives the current element, its index, and the entire array as arguments, and should return the transformed JSX code for that element.
Here is an example of using the map function to render a list of items:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; // Using the map function to render a list of items const renderedItems = items.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of items and we use the map function to transform each item into a list item element. The key prop is used to provide a unique identifier for each list item, which helps React optimize the rendering process. Finally, we render the renderedItems array inside the ul element using curly braces.
Using the map function is a more declarative and concise approach compared to using a for loop, as it separates the logic of transforming the data from the rendering code. It also allows React to efficiently update the DOM when the array changes.
The forEach function
The forEach function is another method available for iterating over an array in React. However, unlike the map function, the forEach function does not return a new array of transformed elements. Instead, it executes a callback function for each element in the array, but it does not allow you to directly generate JSX code.
Here is an example of using the forEach function:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; // Using the forEach function to log each item items.forEach((item, index) => { console.log(`Item ${index + 1}: ${item}`); }); return ( <div> <p>Check the console for item logs.</p> </div> ); } } export default MyComponent;
In this example, we use the forEach function to log each item in the items array to the console. The callback function receives the current element, its index, and the entire array as arguments. We then render a simple paragraph element to indicate where to check for the item logs.
While the forEach function can be useful for performing side effects, such as logging or updating external state, it is not suitable for generating JSX code directly. If you need to render dynamic content based on an array, it is recommended to use the map function instead.
Using a for loop with a helper function
If you have a specific requirement that cannot be easily accomplished with the map function or other higher-order functions, you can use a for loop in combination with a helper function to generate the desired JSX code.
Here is an example of using a for loop with a helper function:
import React from 'react'; class MyComponent extends React.Component { renderItems() { const items = ['item1', 'item2', 'item3']; const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li key={i}>{items[i]}</li>); } return renderedItems; } render() { return ( <div> <ul> {this.renderItems()} </ul> </div> ); } } export default MyComponent;
In this example, we define a helper function called renderItems, which encapsulates the for loop logic for generating the JSX code. Inside the for loop, we push each list item element into the renderedItems array. Finally, we call the renderItems function inside the render method to render the array of list items.
Using a helper function can make the code more modular and reusable, as the logic for generating the JSX code is separated from the rendering code. However, it is important to note that using a for loop in this way should be a last resort, and you should consider if there are alternative approaches that are more in line with React's declarative and functional programming principles.
Exploring the Map Function in ReactJS
As mentioned earlier, the map function is a useful mechanism in React for iterating over an array and transforming each element into JSX code. It allows you to create dynamic content based on the data in the array, and is widely used in React components.
The map function takes a callback function as an argument, which is executed for each element in the array. The callback function receives the current element, its index, and the entire array as arguments, and should return the transformed JSX code for that element.
Here is a more detailed example of using the map function:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; const renderedItems = items.map((item, index) => { // Transform each item into JSX code return <li key={index}>{item}</li>; }); return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of items and we use the map function to transform each item into a list item element. The callback function receives the current item and its index as arguments, and we use these values to create a unique key prop for each list item. Finally, we render the renderedItems array inside the ul element using curly braces.
The map function is a declarative and functional approach to iterating over an array in React. It separates the logic of transforming the data from the rendering code, making the code more readable and maintainable. Additionally, React can efficiently update the DOM when the array changes, thanks to the unique key prop provided for each list item.
Related Article: Exploring Differences in Rendering Components in ReactJS
Dynamic Rendering in ReactJS
Dynamic rendering is a common requirement in React applications, as it allows you to generate UI components based on changing data or user interactions. React provides several mechanisms for dynamic rendering, such as conditional rendering, mapping over arrays, and using component state and props.
Conditional rendering
Conditional rendering is the process of rendering different components or elements based on certain conditions. It allows you to control what gets displayed on the screen based on the current state or props of the component.
Here is an example of conditional rendering in React:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <h1>Welcome, User!</h1> ) : ( <h1>Please log in.</h1> )} </div> ); } } export default MyComponent;
In this example, we have a component with a state property called isLoggedIn, which is initially set to false. Depending on the value of isLoggedIn, we render either a "Welcome, User!" heading or a "Please log in." heading.
Conditional rendering is a useful technique that allows you to show different content to users based on their login status, data availability, or any other condition you need to check.
Rendering arrays
React provides the map function, as discussed earlier, for rendering arrays of data. You can use this function to transform each element in an array into JSX code and render it to the screen.
Here is an example of rendering an array of items in React:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; const renderedItems = items.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of items and we use the map function to transform each item into a list item element. The renderedItems array is then rendered inside the ul element using curly braces.
Rendering arrays is a useful technique when you need to display a dynamic list of items, such as a list of blog posts, comments, or products.
Using component state and props
React components have the ability to manage their own state and receive data from their parent components via props. By leveraging component state and props, you can dynamically render components based on changes in data or user interactions.
Here is an example of using component state to dynamically render a component in React:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { showContent: true, }; } toggleContent = () => { this.setState(prevState => ({ showContent: !prevState.showContent, })); }; render() { const { showContent } = this.state; return ( <div> <button onClick={this.toggleContent}> {showContent ? 'Hide Content' : 'Show Content'} </button> {showContent && <p>This is some dynamic content!</p>} </div> ); } } export default MyComponent;
In this example, we have a component with a state property called showContent, which is initially set to true. Depending on the value of showContent, we render either a "Hide Content" or "Show Content" button, as well as a paragraph element containing some dynamic content.
Using component state and props allows you to build interactive and dynamic user interfaces in React, where components can respond to user input and update their rendering accordingly.
Related Article: How to Set Up Your First ReactJS Project
Implementing For Loop in React Components
While using a for loop in the render method of a React component is generally discouraged, there may be situations where you still need to use a for loop for specific requirements. In such cases, you can encapsulate the for loop inside a helper function or a separate method within the component.
Here is an example of implementing a for loop inside a React component:
import React from 'react'; class MyComponent extends React.Component { renderItems() { const items = ['item1', 'item2', 'item3']; const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li key={i}>{items[i]}</li>); } return renderedItems; } render() { return ( <div> <ul> {this.renderItems()} </ul> </div> ); } } export default MyComponent;
In this example, we define a helper method called renderItems within the MyComponent class. Inside this method, we use a for loop to iterate over the items array and push each item as a list item element into the renderedItems array. Finally, we call the renderItems method inside the render method to render the array of list items.
Using a helper method like this allows you to keep the for loop logic separate from the main render method, making the code more modular and easier to understand. However, it is important to consider if there are alternative approaches, such as using the map function, that are more in line with React's declarative and functional programming principles.
Conditional Rendering Best Practices in ReactJS
Conditional rendering is a useful technique in React that allows you to control what gets displayed on the screen based on certain conditions. While there are multiple ways to implement conditional rendering in React, there are some best practices to keep in mind to ensure clean and maintainable code.
Using the ternary operator
The ternary operator (condition ? expression1 : expression2
) is a concise way to conditionally render different elements or components in React. It allows you to write conditional logic directly within JSX code, making it easier to read and understand.
Here is an example of using the ternary operator for conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <h1>Welcome, User!</h1> ) : ( <h1>Please log in.</h1> )} </div> ); } } export default MyComponent;
In this example, we have a component with a state property called isLoggedIn, which is initially set to false. Depending on the value of isLoggedIn, we render either a "Welcome, User!" heading or a "Please log in." heading using the ternary operator.
The ternary operator is a concise and readable way to handle simple conditional rendering. However, if the condition becomes more complex or the JSX code within the expressions becomes longer, it may be better to extract the conditional logic into a separate method or component for better readability.
Using a separate method or component for conditional rendering
When the conditional rendering logic becomes more complex or involves multiple elements or components, it is recommended to extract the logic into a separate method or component for better organization and maintainability.
Here is an example of using a separate method for conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } renderContent() { const { isLoggedIn } = this.state; if (isLoggedIn) { return <h1>Welcome, User!</h1>; } else { return <h1>Please log in.</h1>; } } render() { return ( <div> {this.renderContent()} </div> ); } } export default MyComponent;
In this example, we define a separate method called renderContent within the MyComponent class. Inside this method, we encapsulate the conditional rendering logic and return the appropriate JSX code based on the value of isLoggedIn. The renderContent method is then called inside the render method to render the content.
Using a separate method or component for conditional rendering can make the code more modular and easier to read, especially when the conditional logic becomes more complex or involves multiple elements or components.
Related Article: Exploring Buffer Usage in ReactJS
Avoiding excessive nesting
When implementing conditional rendering, it's important to avoid excessive nesting of JSX code. Excessive nesting can make the code harder to read and understand, and can also negatively impact performance.
Here is an example of excessive nesting in conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <div> <h1>Welcome, User!</h1> <p>Here is some content.</p> <button>Logout</button> </div> ) : ( <div> <h1>Please log in.</h1> <p>Click the button below to log in.</p> <button>Login</button> </div> )} </div> ); } } export default MyComponent;
In this example, we have excessive nesting of div elements and other JSX code within the ternary expressions. This can make the code harder to read and understand, especially when the JSX code becomes more complex.
To avoid excessive nesting, you can use React fragments (<>...</>
) to group multiple elements without introducing additional DOM nodes. React fragments allow you to avoid unnecessary nesting and keep the JSX code clean and concise.
Here is an example of using React fragments to avoid excessive nesting:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <> <h1>Welcome, User!</h1> <p>Here is some content.</p> <button>Logout</button> </> ) : ( <> <h1>Please log in.</h1> <p>Click the button below to log in.</p> <button>Login</button> </> )} </div> ); } } export default MyComponent;
Using React fragments allows you to group multiple elements without introducing unnecessary nesting. This helps to keep the JSX code clean and readable, while avoiding potential performance issues caused by excessive nesting.
Optimizing Performance with For Loop in Render Method
Using a for loop in the render method of a React component can have performance implications, especially when dealing with large datasets or complex calculations. However, there are certain optimizations you can apply to improve the performance of your React components when using a for loop in the render method.
Minimize calculations within the loop
When using a for loop in the render method, it is important to minimize any calculations or operations that are performed within the loop. This is because the render method is called frequently, and any unnecessary calculations can negatively impact performance.
Here is an example of minimizing calculations within a for loop in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; const renderedItems = []; for (let i = 0; i < items.length; i++) { const item = items[i]; const transformedItem = transformItem(item); // Expensive calculation renderedItems.push(<li key={i}>{transformedItem}</li>); } return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we have a for loop that iterates over an array of items. Within the loop, we perform an expensive calculation using the transformItem function. To optimize performance, we move the calculation outside the loop and store the result in a variable. This way, the calculation is only performed once, instead of on each iteration of the loop.
Use keys for efficient updates
When rendering a list of items using a for loop in the render method, it is important to provide a unique key prop for each list item. The key prop helps React identify each list item and efficiently update the DOM when the list changes.
Here is an example of using keys for efficient updates in a for loop:
import React from 'react'; class MyComponent extends React.Component { render() { const items = ['item1', 'item2', 'item3']; const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li key={i}>{items[i]}</li>); } return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we use the index of each item in the array as the key prop for the corresponding list item. By providing a unique key for each list item, React can efficiently update the DOM when the list changes, without re-rendering the entire list.
Using keys for efficient updates is especially important when the list of items is dynamic and can change frequently. It helps React optimize the rendering process and improve the performance of your React components.
Related Article: How to Add If Statements in a ReactJS Render Method
Avoid unnecessary re-renders
When using a for loop in the render method, it is important to avoid unnecessary re-renders of the component. Unnecessary re-renders can occur when the component's state or props change, causing the render method to be called even if the content rendered by the for loop has not changed.
To avoid unnecessary re-renders, you can use React's PureComponent class or implement the shouldComponentUpdate method. These approaches allow you to control when the component should re-render based on changes in its state or props.
Here is an example of using PureComponent to avoid unnecessary re-renders:
import React from 'react'; class MyComponent extends React.PureComponent { render() { const items = ['item1', 'item2', 'item3']; const renderedItems = []; for (let i = 0; i < items.length; i++) { renderedItems.push(<li key={i}>{items[i]}</li>); } return ( <div> <ul> {renderedItems} </ul> </div> ); } } export default MyComponent;
In this example, we extend the React.PureComponent class instead of React.Component. PureComponent implements the shouldComponentUpdate method with a shallow comparison of the component's props and state, and automatically prevents unnecessary re-renders if the props and state have not changed.
Using PureComponent or implementing the shouldComponentUpdate method can help optimize the performance of your React components when using a for loop in the render method, by avoiding unnecessary re-renders and reducing the overall processing time.
Limitations of Using For Loop in Render Method
While it is technically possible to use a for loop in the render method of a React component, there are several limitations and considerations to keep in mind when using this approach.
Performance issues
Using a for loop in the render method can have performance implications, especially when dealing with large datasets or complex calculations. The render method is called frequently, and any calculations or operations performed within the loop can negatively impact performance.
To optimize performance, it is important to minimize calculations within the loop and use keys for efficient updates, as discussed earlier. Additionally, it is recommended to consider alternative approaches, such as using the map function or other higher-order functions provided by React, which are more in line with React's declarative and functional programming principles.
Maintainability and readability
Using a for loop in the render method can make the code harder to read and maintain, especially if the loop contains complex logic or involves multiple elements or components. It can also deviate from React's declarative and functional programming principles, which can make it more difficult for other developers to understand and work with the code.
To improve maintainability and readability, it is recommended to encapsulate the for loop logic within a helper function or a separate method within the component. This way, the logic for generating the JSX code is separated from the rendering code, making the code more modular and easier to understand.
Related Article: How Rendering Works in ReactJS
Inefficiencies in re-rendering
When using a for loop in the render method, React may re-render the entire list of items even if only one item has changed. This can result in inefficiencies in re-rendering and can negatively impact performance, especially when dealing with long lists or frequently changing data.
To address this issue, it is important to provide a unique key prop for each list item when rendering a list using a for loop. The key prop helps React identify each list item and efficiently update the DOM when the list changes, without re-rendering the entire list.
Declarative vs imperative programming
Using a for loop in the render method can introduce imperative programming into the component, which goes against React's declarative programming paradigm. React encourages you to think in terms of data flow and component composition, rather than imperative control flow statements like for loops.
To maintain a declarative programming style, it is recommended to use more declarative and functional approaches, such as the map function or other higher-order functions provided by React. These approaches separate the logic of transforming the data from the rendering code, making the code more readable and maintainable.
Iterating Through Data in ReactJS
Iterating through data is a common task in React applications, as it allows you to dynamically generate UI components based on the data. React provides several mechanisms for iterating through data and rendering dynamic content, such as the map function, iterators, and higher-order functions.
The map function
The map function is a useful tool in React for iterating over an array and transforming each element into JSX code. It allows you to create dynamic content based on the data in the array, and is widely used in React components.
Here is an example of using the map function to iterate through data in React:
import React from 'react'; class MyComponent extends React.Component { render() { const data = ['item1', 'item2', 'item3']; const renderedData = data.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedData} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of data and we use the map function to transform each item into a list item element. The renderedData array is then rendered inside the ul element using curly braces.
The map function is a declarative and functional approach to iterating through data in React. It separates the logic of transforming the data from the rendering code, making the code more readable and maintainable. Additionally, React can efficiently update the DOM when the array changes, thanks to the unique key prop provided for each list item.
Related Article: Accessing Array Length in this.state in ReactJS
Iterators and generators
In addition to the map function, React supports other mechanisms for iterating through data, such as iterators and generators. These mechanisms provide more flexibility and control over the iteration process, allowing you to implement custom iteration logic.
Here is an example of using an iterator to iterate through data in React:
import React from 'react'; class MyComponent extends React.Component { *iterateData() { yield 'item1'; yield 'item2'; yield 'item3'; } render() { const renderedData = []; for (const item of this.iterateData()) { renderedData.push(<li key={item}>{item}</li>); } return ( <div> <ul> {renderedData} </ul> </div> ); } } export default MyComponent;
In this example, we define a generator function called iterateData within the MyComponent class. The generator function uses the yield keyword to define the iteration logic. Inside the render method, we use a for...of loop to iterate over the data generated by the iterateData function and push each item as a list item element into the renderedData array.
Using iterators and generators allows you to implement custom iteration logic, which can be useful in certain scenarios where the data needs to be processed or transformed before rendering.
Higher-order functions
Higher-order functions are functions that take one or more functions as arguments and/or return a function. They can be used to iterate through data and perform operations or transformations on each element.
Here is an example of using a higher-order function to iterate through data in React:
import React from 'react'; class MyComponent extends React.Component { render() { const data = ['item1', 'item2', 'item3']; const renderedData = data.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedData} </ul> </div> ); } } export default MyComponent;
In this example, we use the map function as a higher-order function to iterate through the data array and transform each item into a list item element. The higher-order function takes a callback function as an argument, which is executed for each element in the array.
Using higher-order functions like map allows you to encapsulate the iteration logic within a single function, making the code more modular and easier to understand. Additionally, higher-order functions provide a declarative and functional approach to iterating through data in React.
Alternatives to Using For Loop in Render Method
While it is technically possible to use a for loop in the render method of a React component, there are alternative approaches that are more in line with React's declarative and functional programming principles. These alternative approaches provide more concise and readable code, as well as better performance and maintainability.
The map function
The map function is a useful mechanism in React for iterating over an array and transforming each element into JSX code. It allows you to create dynamic content based on the data in the array, and is widely used in React components.
Here is an example of using the map function as an alternative to using a for loop in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const data = ['item1', 'item2', 'item3']; const renderedData = data.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedData} </ul> </div> ); } } export default MyComponent;
In this example, we have an array of data and we use the map function to transform each item into a list item element. The renderedData array is then rendered inside the ul element using curly braces.
Using the map function provides a more declarative and functional approach to iterating over data in React. It separates the logic of transforming the data from the rendering code, making the code more readable and maintainable. Additionally, React can efficiently update the DOM when the array changes, thanks to the unique key prop provided for each list item.
Related Article: How To Develop a Full Application with ReactJS
Higher-order functions
Higher-order functions are functions that take one or more functions as arguments and/or return a function. They can be used as an alternative to using a for loop in the render method to iterate through data and perform operations or transformations on each element.
Here is an example of using a higher-order function as an alternative to using a for loop in the render method:
import React from 'react'; class MyComponent extends React.Component { render() { const data = ['item1', 'item2', 'item3']; const renderedData = data.map((item, index) => ( <li key={index}>{item}</li> )); return ( <div> <ul> {renderedData} </ul> </div> ); } } export default MyComponent;
In this example, we use the map function as a higher-order function to iterate through the data array and transform each item into a list item element. The higher-order function takes a callback function as an argument, which is executed for each element in the array.
Using higher-order functions like map allows you to encapsulate the iteration logic within a single function, making the code more modular and easier to understand. Additionally, higher-order functions provide a declarative and functional approach to iterating through data in React.
Best Practices for Conditional Rendering in ReactJS
Conditional rendering is a useful technique in React that allows you to control what gets displayed on the screen based on certain conditions. To ensure clean and maintainable code, it is important to follow best practices when implementing conditional rendering in your React components.
Use the ternary operator for simple conditions
The ternary operator (condition ? expression1 : expression2
) is a concise and readable way to implement simple conditional rendering in React. It allows you to conditionally render different elements or components based on a single condition.
Here is an example of using the ternary operator for simple conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <h1>Welcome, User!</h1> ) : ( <h1>Please log in.</h1> )} </div> ); } } export default MyComponent;
In this example, we have a component with a state property called isLoggedIn, which is initially set to false. Depending on the value of isLoggedIn, we render either a "Welcome, User!" heading or a "Please log in." heading using the ternary operator.
Using the ternary operator is a concise and readable way to handle simple conditional rendering. It clearly expresses the condition and the corresponding JSX code, making the code easier to understand and maintain.
Use a separate method or component for complex conditions
When the conditional rendering logic becomes more complex or involves multiple elements or components, it is recommended to extract the logic into a separate method or component. This improves the maintainability and readability of the code, as well as promotes code reuse.
Here is an example of using a separate method for complex conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } renderContent() { const { isLoggedIn } = this.state; if (isLoggedIn) { return <h1>Welcome, User!</h1>; } else { return <h1>Please log in.</h1>; } } render() { return ( <div> {this.renderContent()} </div> ); } } export default MyComponent;
In this example, we define a separate method called renderContent within the MyComponent class. Inside this method, we encapsulate the conditional rendering logic and return the appropriate JSX code based on the value of isLoggedIn. The renderContent method is then called inside the render method to render the content.
Using a separate method or component for complex conditional rendering improves the maintainability and readability of the code, as well as promotes code reuse. It allows you to clearly separate the conditional logic from the rendering code, making the code easier to understand and maintain.
Related Article: How to Fetch and Post Data to a Server Using ReactJS
Avoid excessive nesting
When implementing conditional rendering, it is important to avoid excessive nesting of JSX code. Excessive nesting can make the code harder to read and understand, and can potentially introduce performance issues.
Here is an example of excessive nesting in conditional rendering:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <div> <h1>Welcome, User!</h1> <p>Here is some content.</p> <button>Logout</button> </div> ) : ( <div> <h1>Please log in.</h1> <p>Click the button below to log in.</p> <button>Login</button> </div> )} </div> ); } } export default MyComponent;
In this example, we have excessive nesting of div elements and other JSX code within the ternary expressions. This can make the code harder to read and understand, especially when the JSX code becomes more complex.
To avoid excessive nesting, you can use React fragments (<>...</>
) to group multiple elements without introducing additional DOM nodes. React fragments allow you to avoid unnecessary nesting and keep the JSX code clean and concise.
Here is an example of using React fragments to avoid excessive nesting:
import React from 'react'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { isLoggedIn: false, }; } render() { const { isLoggedIn } = this.state; return ( <div> {isLoggedIn ? ( <> <h1>Welcome, User!</h1> <p>Here is some content.</p> <button>Logout</button> </> ) : ( <> <h1>Please log in.</h1> <p>Click the button below to log in.</p> <button>Login</button> </> )} </div> ); } } export default MyComponent;
Using React fragments allows you to group multiple elements without introducing unnecessary nesting. This helps to keep the JSX code clean and readable, while avoiding potential performance issues caused by excessive nesting.
Additional Resources
- Functional Components vs Class Components in React