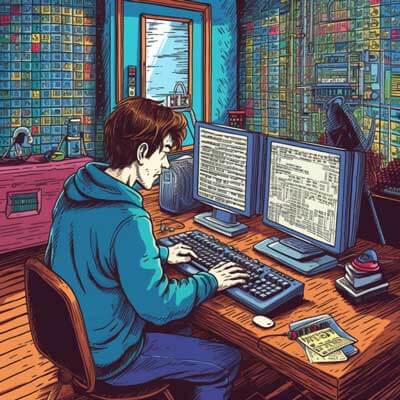
Table of Contents
Understanding the hover state in ReactJS
In ReactJS, the hover state refers to the state of an element when the user hovers over it with their mouse cursor. This state change can be used to apply different styles or perform specific actions on the element. The hover state is commonly used to provide visual feedback to the user, such as highlighting a button when it is being hovered over.
To understand how the hover state works in ReactJS, let's consider a simple example. Suppose we have a button component that we want to apply a hover effect to. We can define a state variable to keep track of whether the button is being hovered over or not. We can then use this state variable to conditionally apply a different style to the button when it is being hovered over.
import React, { useState } from 'react'; const Button = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; const buttonStyle = { background: isHovered ? 'blue' : 'red', color: 'white', padding: '10px 20px', borderRadius: '5px', }; return ( <button style={buttonStyle} onMouseEnter={handleMouseEnter} onMouseLeave={handleMouseLeave} > Hover Me </button> ); }; export default Button;
In this example, we use the useState
hook to define the isHovered
state variable and the setIsHovered
function to update its value. We also define two event handlers, handleMouseEnter
and handleMouseLeave
, which are called when the user hovers over or leaves the button, respectively. Inside the event handlers, we update the isHovered
state variable accordingly.
The buttonStyle
variable is an object that contains the styles for the button. We use the isHovered
state variable to conditionally apply different background colors to the button depending on whether it is being hovered over or not. The other styles, such as color, padding, and borderRadius, remain the same regardless of the hover state.
Related Article: How to Use a For Loop Inside Render in ReactJS
Implementing hover state using inline styles in ReactJS
Implementing the hover state in ReactJS using inline styles is straightforward. Inline styles allow us to define the styles directly inside the JSX code, making it easy to apply dynamic styles based on the hover state.
Let's continue with the previous example of the button component and enhance it by adding a hover effect using inline styles.
import React, { useState } from 'react'; const Button = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; const buttonStyle = { background: isHovered ? 'blue' : 'red', color: 'white', padding: '10px 20px', borderRadius: '5px', transition: 'background 0.3s', cursor: 'pointer', }; return ( <button style={buttonStyle} onMouseEnter={handleMouseEnter} onMouseLeave={handleMouseLeave} > Hover Me </button> ); }; export default Button;
In this example, we added two additional styles to the buttonStyle
object: transition
and cursor
. The transition
property adds a smooth transition effect when the background color changes, creating a more visually appealing hover effect. The cursor
property changes the mouse cursor to a pointer, indicating to the user that the button is interactive.
Advantages of using inline styles in ReactJS for hover states
Using inline styles in ReactJS for hover states offers several advantages:
1. Easy integration: Inline styles can be defined directly within the JSX code, making it easy to integrate styles with the component's logic.
2. Dynamic styling: Inline styles allow for dynamic styling based on component state, such as the hover state. This flexibility enables interactive and responsive user interfaces.
3. Scoped styles: Inline styles are scoped to the component they are defined in, reducing the risk of CSS class name collisions and making it easier to manage styles within a component.
4. No external dependencies: Inline styles do not require external CSS files or CSS-in-JS libraries, reducing the number of dependencies in the project and simplifying the build process.
5. Performance optimization: Inline styles can be optimized by using memoization techniques, ensuring that style objects are only re-rendered when necessary. This can improve the performance of React components.
Let's consider an example to demonstrate the advantages of using inline styles in ReactJS for hover states.
import React, { useState } from 'react'; const Button = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; const buttonStyle = { background: isHovered ? 'blue' : 'red', color: 'white', padding: '10px 20px', borderRadius: '5px', transition: 'background 0.3s', cursor: 'pointer', }; return ( <button style={buttonStyle} onMouseEnter={handleMouseEnter} onMouseLeave={handleMouseLeave} > Hover Me </button> ); }; export default Button;
In this example, we can easily integrate the inline styles with the button component. The styles are defined directly within the JSX code, making it easy to understand and maintain the component's styling. The dynamic styling based on the hover state allows for interactive user interfaces, enhancing the user experience.
The scoped nature of inline styles ensures that the styles defined for the button component do not affect other components or elements on the page. This reduces the chances of CSS class name collisions and makes it easier to manage the styles within the component.
Since inline styles do not require external CSS files or CSS-in-JS libraries, there are no additional dependencies to include in the project. This simplifies the build process and reduces the overall project complexity.
Finally, inline styles can be optimized for performance by using memoization techniques. This ensures that the style objects are only re-rendered when necessary, improving the performance of React components.
Overall, using inline styles in ReactJS for hover states offers several advantages in terms of integration, dynamic styling, scoping, simplicity, and performance optimization.
Performance considerations when using inline styles for hover states in ReactJS
When using inline styles for hover states in ReactJS, there are several performance considerations to keep in mind to ensure optimal rendering and user experience.
1. Avoid excessive re-rendering: Inline styles are defined as objects, and any change in the style object will trigger a re-render of the component. To avoid excessive re-rendering, consider using memoization techniques, such as the useMemo
hook, to memoize the style object and only recompute it when necessary.
import React, { useState, useMemo } from 'react'; const Button = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; const buttonStyle = useMemo( () => ({ background: isHovered ? 'blue' : 'red', color: 'white', padding: '10px 20px', borderRadius: '5px', transition: 'background 0.3s', cursor: 'pointer', }), [isHovered] ); return ( <button style={buttonStyle} onMouseEnter={handleMouseEnter} onMouseLeave={handleMouseLeave} > Hover Me </button> ); }; export default Button;
2. Minimize inline style complexity: Inline styles are defined within the JSX code, and complex styles can lead to bloated and hard-to-read components. To maintain code readability and performance, try to keep inline styles simple and concise. If the styles become too complex, consider extracting them into a separate CSS file or using CSS-in-JS libraries like styled-components.
3. Avoid inline style conflicts: When using inline styles, it's important to avoid conflicts between different style properties or components. Make sure to namespace your style properties to avoid clashes with other components or external styles. Additionally, avoid using the same property in different inline styles within the same component, as this can lead to unpredictable rendering results.
4. Consider CSS-in-JS libraries for complex scenarios: While inline styles can be sufficient for simple hover effects, more complex styling scenarios may require the use of CSS-in-JS libraries like styled-components or emotion. These libraries provide additional features, such as CSS preprocessing, theming, and style composition, which can improve the maintainability and performance of your styles.
Related Article: Executing Multiple Fetch Calls Simultaneously in ReactJS
Using CSS classes vs inline styles for hover states in ReactJS
In ReactJS, you have the option to use CSS classes or inline styles for implementing hover states. Both approaches have their advantages and considerations, and the choice between them depends on the specific requirements of your project.
Let's compare the usage of CSS classes and inline styles for implementing hover states in ReactJS.
CSS Classes:
Using CSS classes for hover states in ReactJS involves defining separate CSS classes with hover styles and applying them to the elements that need to change their styles on hover. This approach follows the traditional separation of concerns principle, where styles are defined separately from the component logic.
import React from 'react'; import './Button.css'; const Button = () => { return ( <button className="button">Hover Me</button> ); }; export default Button;
/* Button.css */ .button { background: red; color: white; padding: 10px 20px; border-radius: 5px; transition: background 0.3s; cursor: pointer; } .button:hover { background: blue; }
In this example, we define a CSS class named "button" that sets the initial styles of the button. We also define a separate CSS rule for the ":hover" pseudo-class, which changes the background color of the button when it is being hovered over.
Using CSS classes for hover states offers the following advantages:
1. Separation of concerns: CSS classes allow for a clear separation between the component logic and the styles. This can make the codebase more maintainable and easier to understand, especially for larger projects.
2. Reusability: CSS classes can be reused across multiple components, reducing code duplication and making it easier to maintain consistent styles throughout the application.
3. Familiarity: Many developers are already familiar with CSS and its concepts, making it easier to work with and understand CSS classes.
However, using CSS classes for hover states also has some considerations:
1. Global scope: CSS classes are applied globally unless scoped using CSS modules or a CSS-in-JS library. This can lead to class name clashes and unintended style changes if not managed carefully.
2. Limited dynamic styling: CSS classes are not designed for dynamic styling based on component state. While it is possible to conditionally apply different classes based on component state, this can lead to more complex code and potentially introduce bugs.
Inline Styles:
Using inline styles for hover states in ReactJS involves defining the styles directly within the JSX code, using JavaScript objects.
import React, { useState } from 'react'; const Button = () => { const [isHovered, setIsHovered] = useState(false); const handleMouseEnter = () => { setIsHovered(true); }; const handleMouseLeave = () => { setIsHovered(false); }; const buttonStyle = { background: isHovered ? 'blue' : 'red', color: 'white', padding: '10px 20px', borderRadius: '5px', transition: 'background 0.3s', cursor: 'pointer', }; return ( <button style={buttonStyle} onMouseEnter={handleMouseEnter} onMouseLeave={handleMouseLeave} > Hover Me </button> ); }; export default Button;
In this example, we define an object named buttonStyle
that contains the styles for the button. The isHovered
state variable is used to conditionally apply different styles to the button based on whether it is being hovered over or not.
Using inline styles for hover states offers the following advantages:
1. Dynamic styling: Inline styles allow for dynamic styling based on component state, such as the hover state. This flexibility enables interactive and responsive user interfaces.
2. Scoped styles: Inline styles are scoped to the component they are defined in, reducing the risk of CSS class name collisions and making it easier to manage styles within a component.
3. No external dependencies: Inline styles do not require external CSS files or CSS-in-JS libraries, reducing the number of dependencies in the project and simplifying the build process.
However, using inline styles for hover states also has some considerations:
1. Increased complexity: Inline styles can become complex and hard to read, especially for large components or when dealing with complex styles. This can make the code harder to maintain and understand.
2. Performance implications: Inline styles can lead to excessive re-rendering if not optimized properly. Memoization techniques, such as the useMemo
hook, can be used to optimize the rendering performance of components with inline styles.